Android实现蓝牙聊天APP
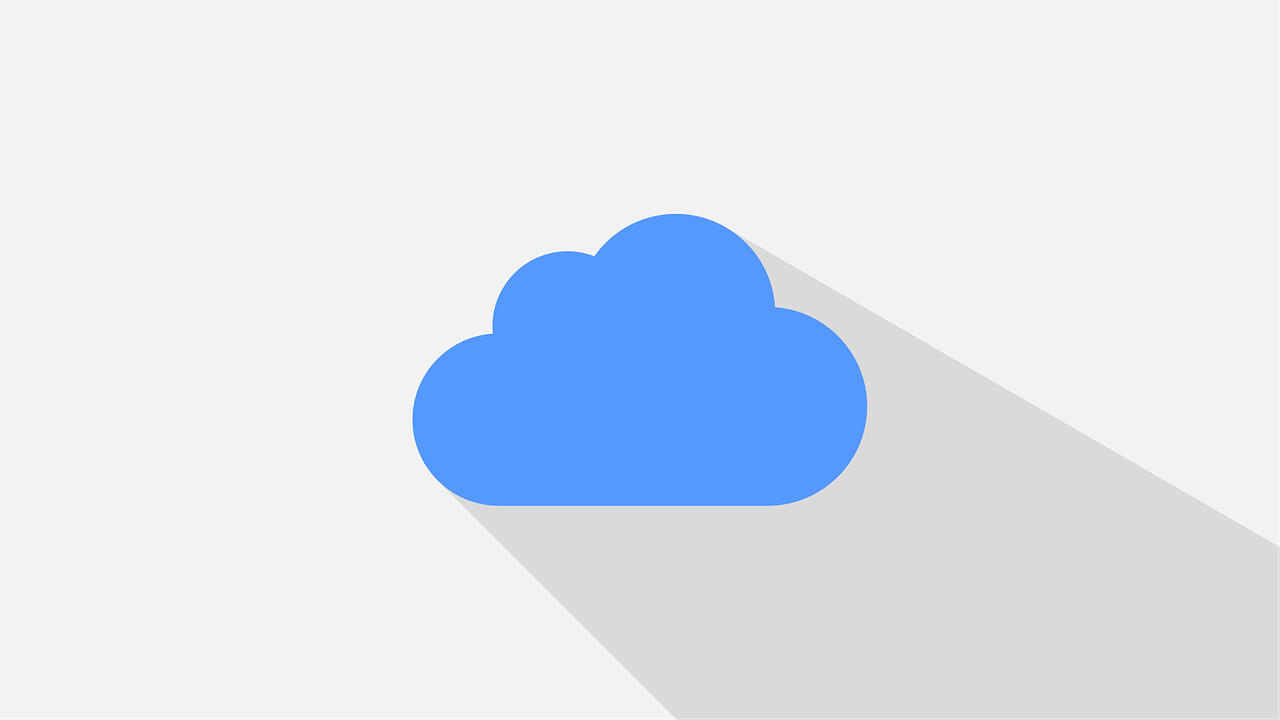
我们将详细介绍如何使用Android开发一个蓝牙聊天应用程序,该应用程序将允许用户通过蓝牙连接进行文本消息的发送和接收,以下是实现这个应用的步骤和代码示例。
1. 环境准备
你需要确保你的开发环境中安装了以下工具:
Android Studio
Android SDK
JDK 8或以上版本
2. 创建项目
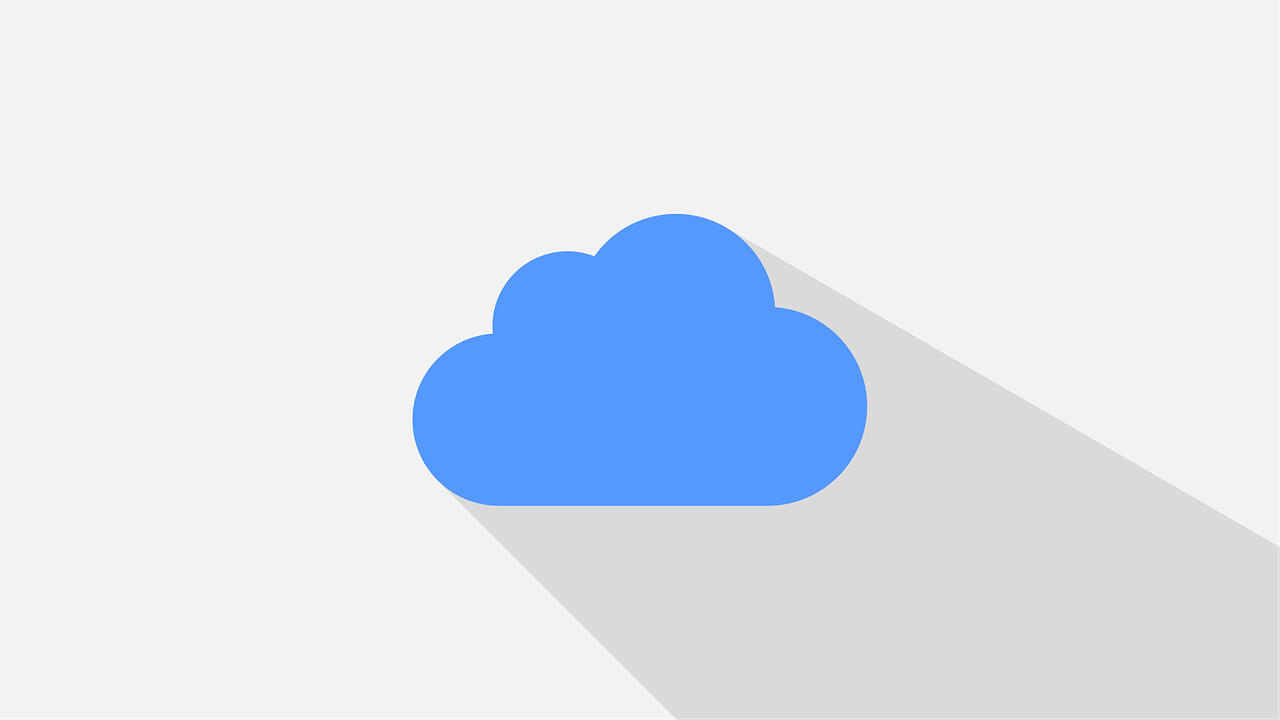
打开Android Studio,创建一个新的项目,选择“Empty Activity”模板。
3. 添加权限
在AndroidManifest.xml
文件中添加必要的权限:
<uses-permission android:name="android.permission.BLUETOOTH" /> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN" /> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
4. 设计UI
在activity_main.xml
中设计一个简单的用户界面,包括一个列表视图来显示聊天记录和一个文本框及按钮用于输入和发送消息。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <ListView android:id="@+id/chat_list_view" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_above="@+id/input_layout" android:divider="@android:color/darker_gray" android:dividerHeight="1dp" /> <LinearLayout android:id="@+id/input_layout" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:layout_alignParentBottom="true" android:padding="10dp"> <EditText android:id="@+id/message_input" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:hint="Enter message" /> <Button android:id="@+id/send_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Send" /> </LinearLayout> </RelativeLayout>
5. 初始化蓝牙适配器
在你的MainActivity
中,初始化蓝牙适配器并检查设备是否支持蓝牙。
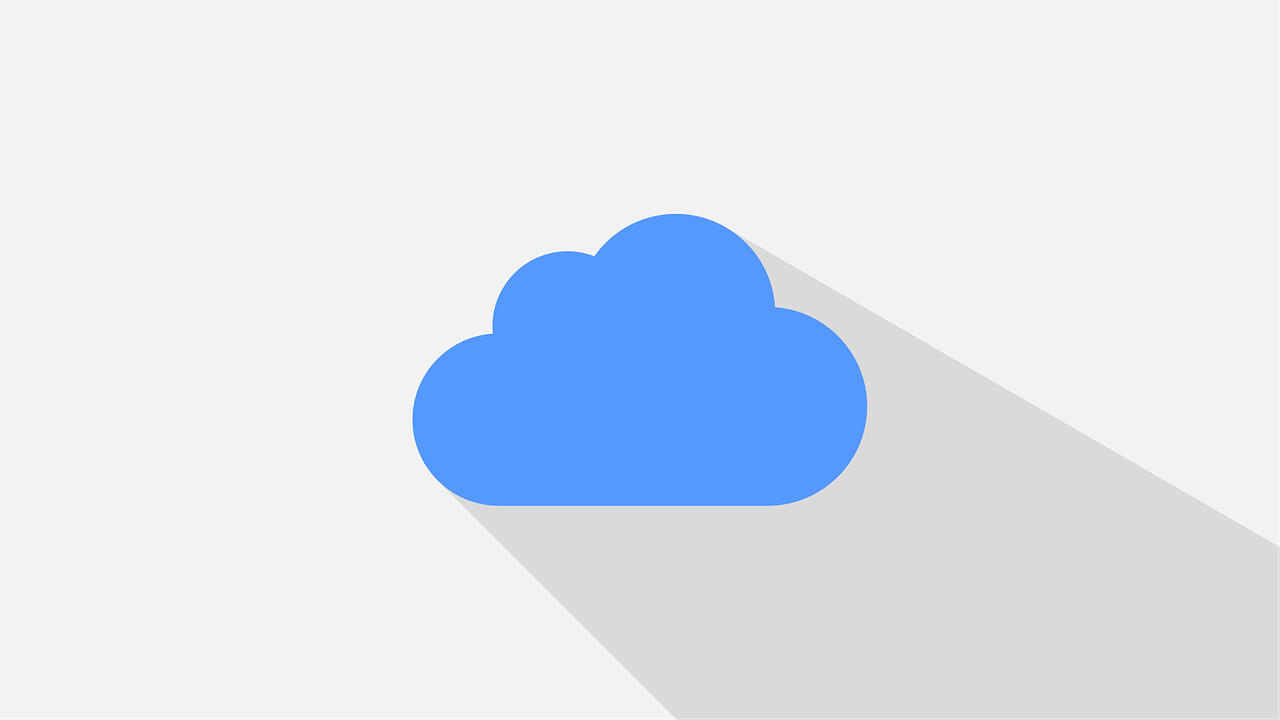
import android.bluetooth.BluetoothAdapter; import android.bluetooth.BluetoothDevice; import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; import android.content.IntentFilter; import android.os.Bundle; import android.view.View; import android.widget.ArrayAdapter; import android.widget.Button; import android.widget.EditText; import android.widget.ListView; import androidx.appcompat.app.AppCompatActivity; import java.util.ArrayList; public class MainActivity extends AppCompatActivity { private BluetoothAdapter bluetoothAdapter; private ArrayAdapter<String> adapter; private ArrayList<String> chatMessages; private ListView chatListView; private EditText messageInput; private Button sendButton; private static final int REQUEST_ENABLE_BT = 1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); chatListView = findViewById(R.id.chat_list_view); messageInput = findViewById(R.id.message_input); sendButton = findViewById(R.id.send_button); chatMessages = new ArrayList<>(); adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, chatMessages); chatListView.setAdapter(adapter); bluetoothAdapter = BluetoothAdapter.getDefaultAdapter(); if (bluetoothAdapter == null) { // Device doesn't support Bluetooth finish(); return; } else if (!bluetoothAdapter.isEnabled()) { Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE); startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT); } sendButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { sendMessage(); } }); }
6. 搜索设备并配对
为了连接到其他蓝牙设备,我们需要搜索附近的设备并进行配对,以下是如何实现这一功能的代码:
private void searchForDevices() { if (bluetoothAdapter.isDiscovering()) { bluetoothAdapter.cancelDiscovery(); } bluetoothAdapter.startDiscovery(); IntentFilter filter = new IntentFilter(BluetoothDevice.ACTION_FOUND); registerReceiver(receiver, filter); } private final BroadcastReceiver receiver = new BroadcastReceiver() { public void onReceive(Context context, Intent intent) { String action = intent.getAction(); if (BluetoothDevice.ACTION_FOUND.equals(action)) { BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE); String deviceName = device.getName(); String deviceAddress = device.getAddress(); adapter.add("Found device: " + deviceName + " [" + deviceAddress + "]"); chatListView.setSelection(adapter.getCount() 1); } } };
7. 发送和接收消息
我们需要实现发送和接收消息的功能,以下是发送消息的代码:
private void sendMessage() { String message = messageInput.getText().toString(); if (message.length() > 0) { adapter.add("Me: " + message); chatListView.setSelection(adapter.getCount() 1); messageInput.setText(""); // TODO: Send the message via Bluetooth to the connected device } }
接收消息的部分需要使用一个BluetoothSocket
来监听连接,并在收到消息时更新UI,这部分代码较为复杂,涉及到线程处理和网络通信,这里只提供一个简单的示例:
private class ChatService extends Thread { private final BluetoothSocket socket; private final InputStream inputStream; private final OutputStream outputStream; public ChatService(BluetoothSocket socket) throws IOException { this.socket = socket; inputStream = socket.getInputStream(); outputStream = socket.getOutputStream(); } public void run() { byte[] buffer = new byte[1024]; int bytes; while (true) { try { bytes = inputStream.read(buffer); String receivedMessage = new String(buffer, 0, bytes); adapter.add("Friend: " + receivedMessage); chatListView.setSelection(adapter.getCount() 1); } catch (IOException e) { break; } } } public void write(byte[] bytes) throws IOException { outputStream.write(bytes); } }
8. 连接设备和管理连接状态
我们需要管理设备的连接状态,确保在连接断开时能够重新连接或者提示用户,这部分代码涉及到更多的细节,如错误处理、重连机制等,这里仅提供一个基本的框架:
private BluetoothSocket connectToDevice(BluetoothDevice device) throws IOException { return device.createRfcommSocketToServiceRecord(MY_UUID); }
本文介绍了如何使用Android开发一个基本的蓝牙聊天应用程序,实际应用中,你可能需要处理更多的细节,如加密通信、更复杂的UI设计、错误处理等,希望这篇文章能帮助你入门蓝牙开发。
到此,以上就是小编对于“Android实现蓝牙聊天APP”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1290216.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复