Android 应用程序开发指南
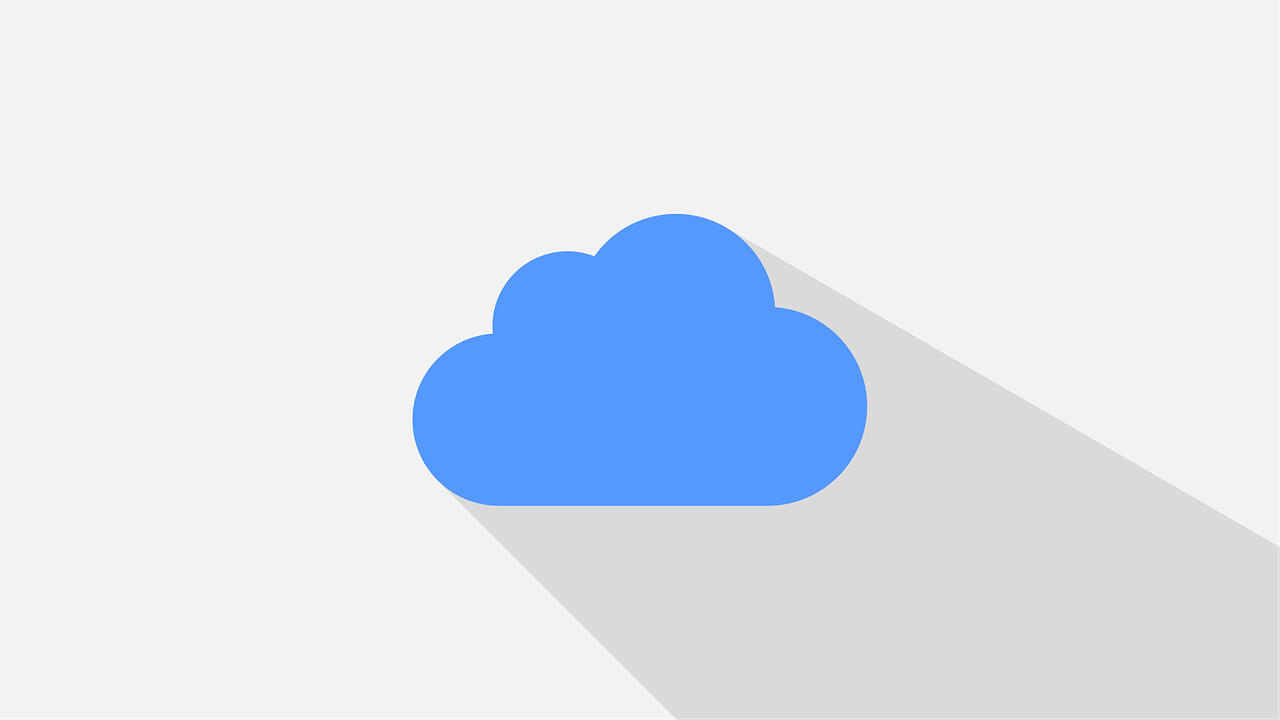
Android 应用程序开发是一个涉及多个阶段的过程,从设计到编码再到测试和发布,本文将详细介绍如何进行 Android 应用程序开发,包括环境搭建、基本架构、UI 设计、数据存储、网络通信等关键方面。
1. 环境搭建
1.1 安装 Java Development Kit (JDK)
下载: 前往 [Oracle 官方网站](https://www.oracle.com/java/technologies/javase-jdk11-downloads.html) 下载 JDK。
安装: 根据操作系统的不同,按照提示完成安装。
1.2 安装 Android Studio
下载: 前往 [Android Studio 官方网站](https://developer.android.google.cn/studio) 下载适用于您操作系统的版本。
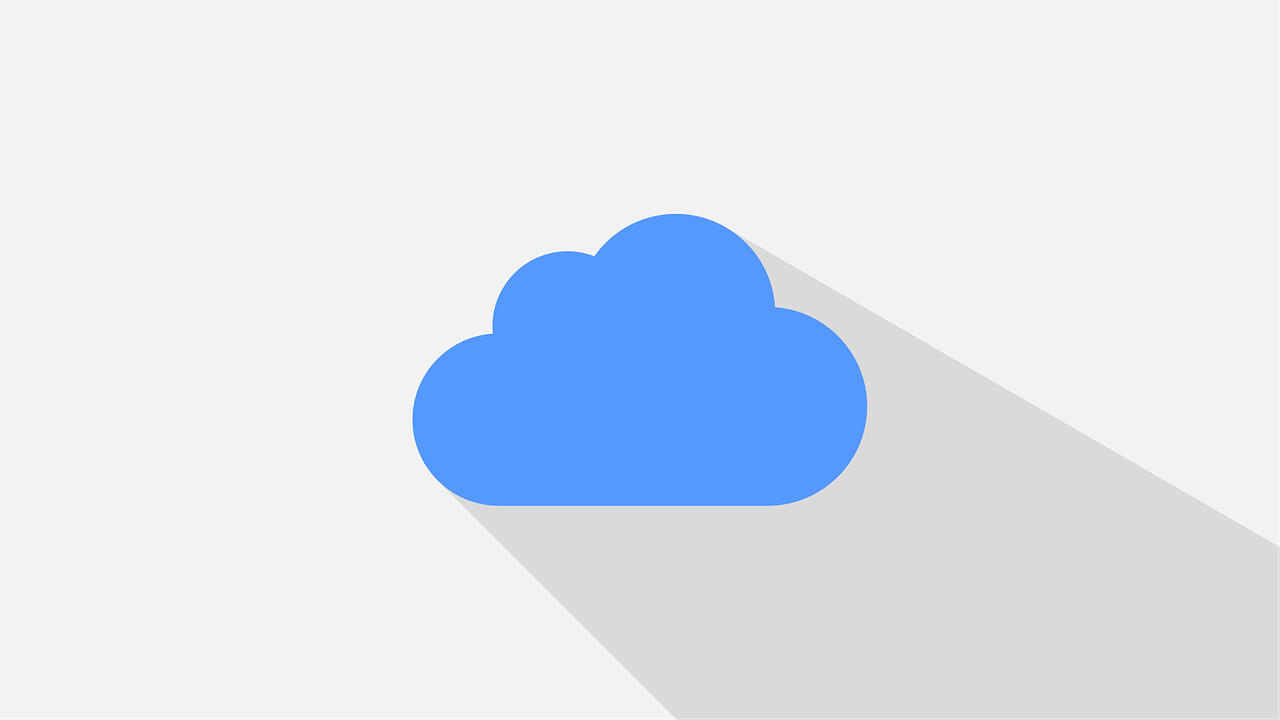
安装: 双击下载的安装包并按照提示完成安装。
配置: 首次启动 Android Studio 时,会要求您设置一些初始配置,如选择 UI 主题等。
3 创建第一个项目
启动 Android Studio: 打开 Android Studio。
创建新项目: 点击 “Start a new Android Studio project”。
配置项目: 填写应用名称、公司域名等信息。
选择模板: 选择一个适合初学者的模板,“Empty Activity”。
完成创建: 点击 “Finish” 完成项目的创建。
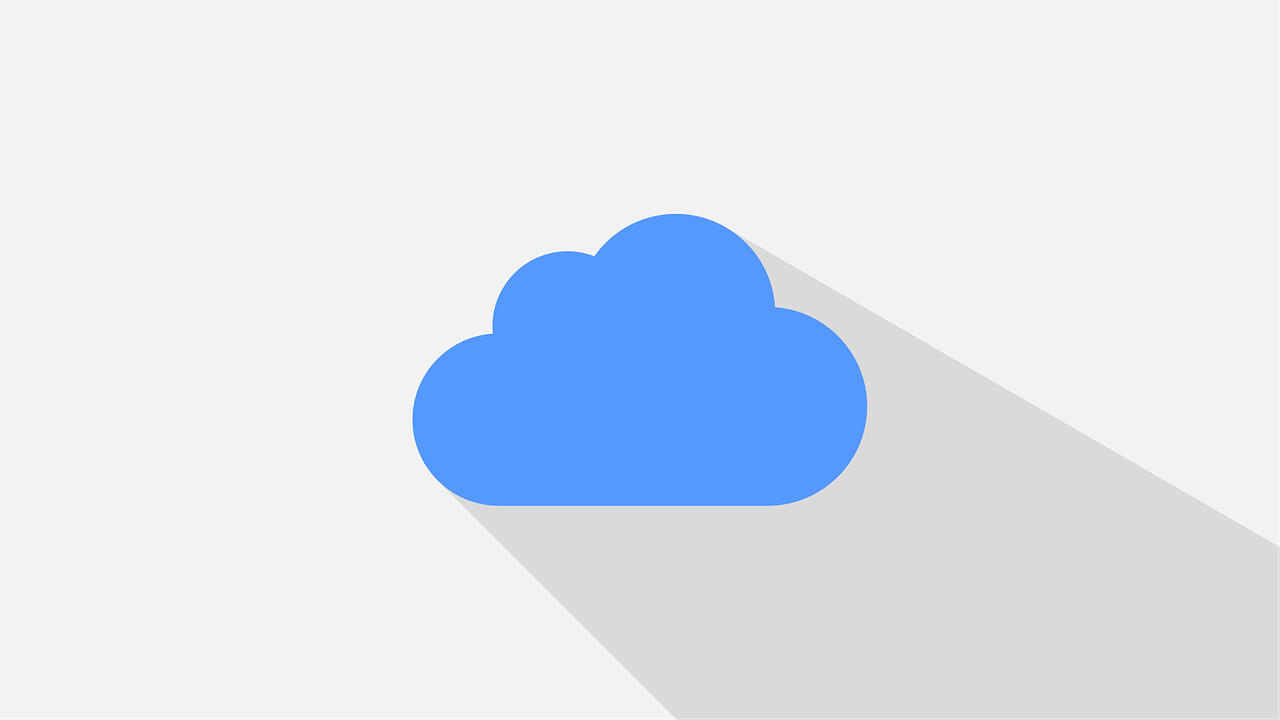
2. Android 应用的基本架构
1 组件
Android 应用由以下四个主要组件构成:
组件 | 描述 |
Activity | 用于呈现用户界面。 |
Service | 在后台运行长时间操作。 |
Content Provider | 用于在不同应用之间共享数据。 |
Broadcast Receiver | 监听系统范围内的广播事件。 |
2 Manifest 文件
每个 Android 应用都必须有一个AndroidManifest.xml
文件,它描述了应用的各个组件及其权限需求。
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.myfirstapp"> <application ... > <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
3. UI 设计
1 布局
Android 提供了多种布局方式,如线性布局、相对布局、约束布局等,常用的布局 XML 代码如下:
线性布局 (LinearLayout)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Click Me"/> </LinearLayout>
约束布局 (ConstraintLayout)
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Click Me" app:layout_constraintTop_toTopOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent"/> </androidx.constraintlayout.widget.ConstraintLayout>
2 控件
Android 提供了丰富的 UI 控件,如按钮 (Button)、文本框 (TextView)、编辑框 (EditText) 等。
<Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Click Me"/>
4. 数据存储
4.1 SharedPreferences
用于存储简单的键值对数据。
// 保存数据 SharedPreferences sharedPref = getSharedPreferences("MyPrefs", MODE_PRIVATE); SharedPreferences.Editor editor = sharedPref.edit(); editor.putString("username", "JohnDoe"); editor.apply(); // 读取数据 String username = sharedPref.getString("username", "defaultValue");
2 SQLite 数据库
用于存储结构化的数据,需要使用SQLiteOpenHelper
类来管理数据库的创建和版本管理。
public class MyDatabaseHelper extends SQLiteOpenHelper { private static final String DATABASE_NAME = "mydatabase.db"; private static final int DATABASE_VERSION = 1; private static final String TABLE_NAME = "users"; private static final String COLUMN_ID = "_id"; private static final String COLUMN_NAME = "name"; private static final String COLUMN_AGE = "age"; public MyDatabaseHelper(Context context) { super(context, DATABASE_NAME, null, DATABASE_VERSION); } @Override public void onCreate(SQLiteDatabase db) { String createTable = "CREATE TABLE " + TABLE_NAME + "(" + COLUMN_ID + " INTEGER PRIMARY KEY AUTOINCREMENT," + COLUMN_NAME + " TEXT," + COLUMN_AGE + " INTEGER)"; db.execSQL(createTable); } @Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME); onCreate(db); } }
5. 网络通信
1 HTTP 请求
可以使用HttpURLConnection
或第三方库如 Retrofit、OkHttp 来进行 HTTP 请求。
使用 Retrofit
首先添加依赖:
implementation 'com.squareup.retrofit2:retrofit:2.9.0' implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
然后定义接口:
public interface APIService { @GET("posts") Call<List<Post>> getPosts(); }
使用 Retrofit 实例化接口:
Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://jsonplaceholder.typicode.com/") .addConverterFactory(GsonConverterFactory.create()) .build(); APIService apiService = retrofit.create(APIService.class); Call<List<Post>> call = apiService.getPosts(); call.enqueue(new Callback<List<Post>>() { @Override public void onResponse(Call<List<Post>> call, Response<List<Post>> response) { if (response.isSuccessful()) { List<Post> posts = response.body(); // 处理返回的数据 } else { // 处理错误 } } @Override public void onFailure(Call<List<Post>> call, Throwable t) { // 处理失败情况 } });
2 WebSockets
用于实现实时双向通信,可以使用第三方库如 OkHttp、Java-WebSocket。
import okhttp3.*; import javax.swing.*; import java.util.concurrent.TimeUnit; public class WebSocketExample { public static void main(String[] args) throws Exception { OkHttpClient client = new OkHttpClient.Builder() .readTimeout(0, TimeUnit.MILLISECONDS) .build(); Request request = new Request.Builder() .url("ws://echo.websocket.org") .build(); WebSocketListener listener = new WebSocketListener() { @Override public void onOpen(WebSocket webSocket, Response response) { webSocket.send("Hello, World!"); } @Override public void onMessage(WebSocket webSocket, String text) { System.out.println("Receiving : " + text); } @Override public void onFailure(WebSocket webSocket, Throwable t, Response response) { t.printStackTrace(); } }; WebSocket ws = client.newWebSocket(request, listener); JOptionPane.showMessageDialog(null, "WebSocket Opened"); // Keep the program running to allow messages to be sent and received. Thread.sleep(5000); // Sleep for 5 seconds before closing the connection. ws.close(1000, "Goodbye"); // Close the connection with status code 1000 and message "Goodbye". } }
6. 调试与测试
1 Logcat
Android Studio 内置的 Logcat 工具可以帮助开发者查看应用的日志输出,便于调试,可以通过Log
类来记录日志信息。
Log.d("MyApp", "This is a debug message"); Log.i("MyApp", "This is an info message"); Log.w("MyApp", "This is a warning message"); Log.e("MyApp", "This is an error message");
6.2 Android Emulator & Real Devices
Android Studio 提供了强大的模拟器功能,可以模拟各种设备和硬件条件进行测试,也可以连接真实设备进行测试,确保应用在多种设备和屏幕尺寸上都能正常运行。
7. 发布应用
在发布应用之前,需要在 Google Play Developer Console 中创建一个开发者账号,并上传 APK 文件,还需要准备应用图标、截图、描述等信息,发布之后,用户就可以在 Google Play Store 上下载和安装你的应用了。
以上内容就是解答有关“android应用程序开发”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1290161.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复