在 Linux 系统中,进行多线程编程通常使用 POSIX 线程库(pthread),为了在 Linux 下编译包含多线程的程序,需要确保正确链接 pthread 库。
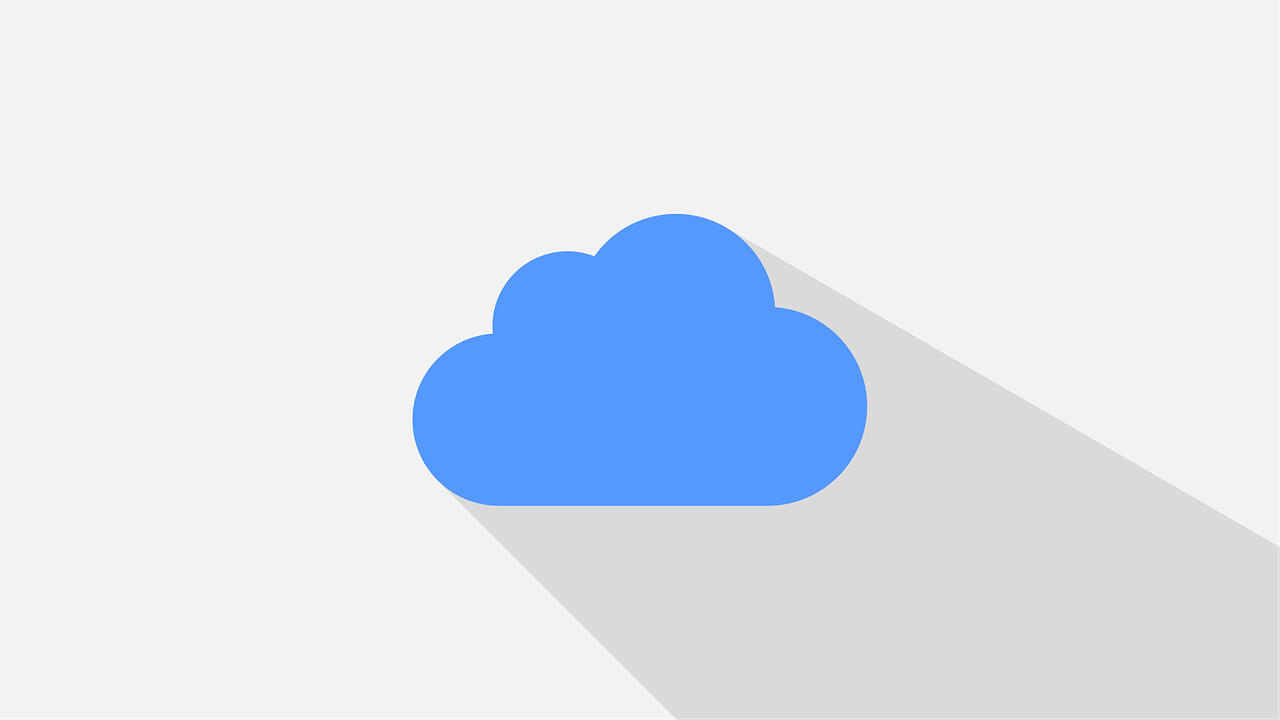
使用 gcc 编译器进行编译时,可以通过添加-pthread
选项来指示编译器链接 pthread 库。
gcc -pthread -o my_program my_program.c
这条命令会将my_program.c
文件编译为可执行文件my_program
,并在编译过程中链接 pthread 库,以便程序能够使用多线程相关的功能。
如果在编译多个源文件时,同样需要在编译命令中添加-pthread
。
gcc -pthread -o my_program main.c thread_func.c
这将把main.c
和thread_func.c
两个源文件一起编译,并生成可执行文件my_program
,同时链接 pthread 库。
还可以在 Makefile 中使用-pthread
选项。
CC = gcc CFLAGS = -Wall -g LDFLAGS = -pthread my_program: main.o thread_func.o $(CC) $(LDFLAGS) -o $@ $^ main.o: main.c $(CC) $(CFLAGS) -c $< -o $@ thread_func.o: thread_func.c $(CC) $(CFLAGS) -c $< -o $@ clean: rm -f *.o my_program
在这个 Makefile 中,LDFLAGS
变量包含了-pthread
选项,这样在链接阶段会自动链接 pthread 库。
在 Linux 下编译多线程程序时,关键是要确保使用-pthread
选项来链接 pthread 库,这样才能正确使用多线程相关的功能。
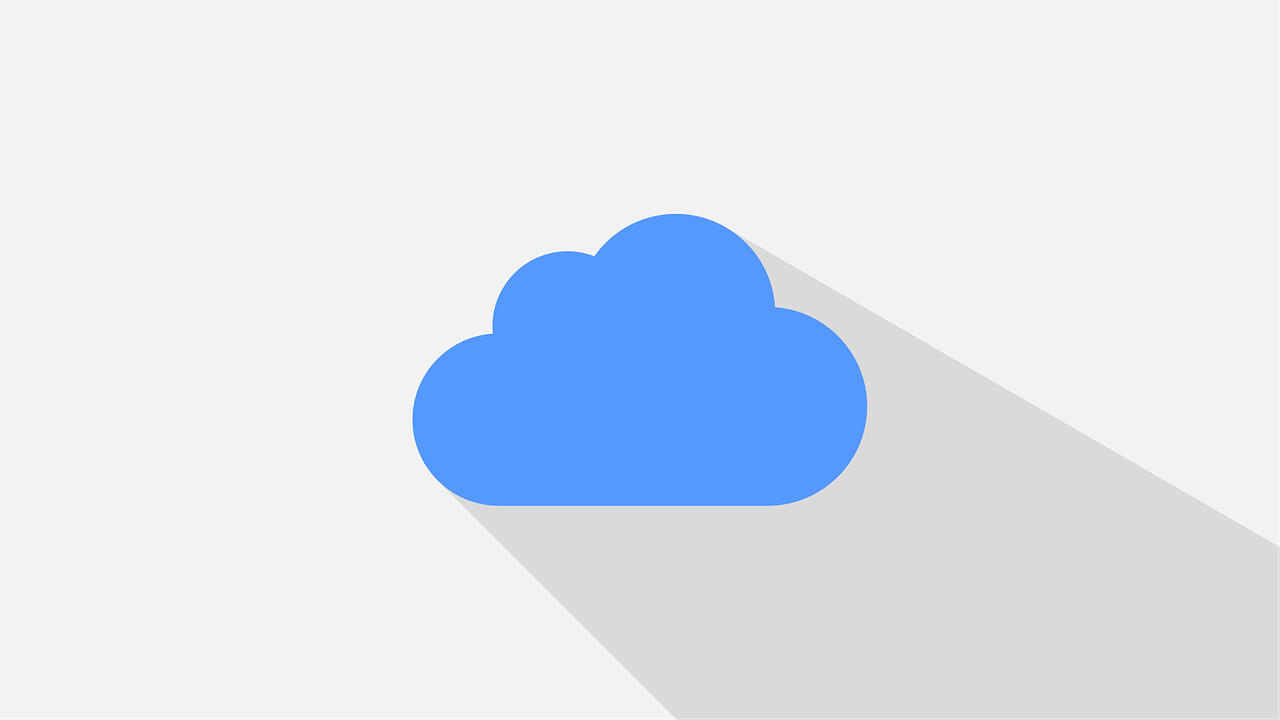
以下是一个简单的多线程示例程序:
#include <stdio.h> #include <stdlib.h> #include <pthread.h> void* thread_func(void* arg) { printf("Hello from the thread! "); return NULL; } int main() { pthread_t thread; int result; result = pthread_create(&thread, NULL, thread_func, NULL); if (result != 0) { fprintf(stderr, "Error creating thread "); return 1; } result = pthread_join(thread, NULL); if (result != 0) { fprintf(stderr, "Error joining thread "); return 1; } printf("Hello from the main program! "); return 0; }
要编译这个程序,可以使用以下命令:
gcc -pthread -o my_program my_program.c
运行生成的可执行文件:
./my_program
输出结果应该是:
Hello from the thread! Hello from the main program!
FAQs
问题 1:为什么编译多线程程序时需要使用-pthread
选项?
答:在 Linux 系统中,pthread 是一个独立的库,提供了多线程的支持,使用-pthread
选项可以告知编译器在链接阶段包含 pthread 库,这样程序才能调用 pthread 提供的各种函数和接口,实现多线程功能,如果不使用-pthread
,编译器在链接时不会包含 pthread 库,可能会导致程序在运行时出现未定义符号的错误。
问题 2:如何在多线程程序中使用互斥锁来保护共享资源?
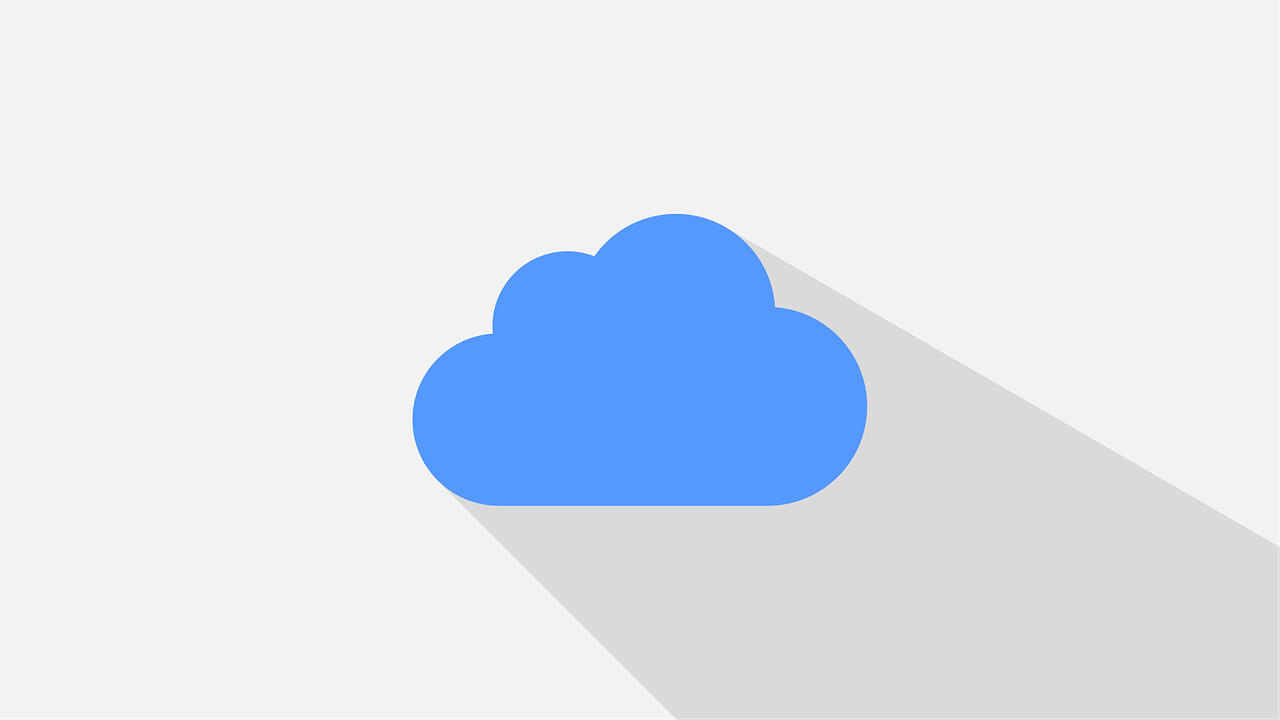
答:在多线程程序中,当多个线程需要访问共享资源时,可以使用互斥锁(mutex)来保护共享资源,避免数据竞争和不一致的问题,可以使用 pthread 库中的互斥锁相关函数来实现,以下是一个示例:
#include <stdio.h> #include <stdlib.h> #include <pthread.h> pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; int shared_counter = 0; void* thread_func(void* arg) { for (int i = 0; i < 100000; i++) { pthread_mutex_lock(&mutex); shared_counter++; pthread_mutex_unlock(&mutex); } return NULL; } int main() { pthread_t threads[10]; int result; for (int i = 0; i < 10; i++) { result = pthread_create(&threads[i], NULL, thread_func, NULL); if (result != 0) { fprintf(stderr, "Error creating thread %d ", i); return 1; } } for (int i = 0; i < 10; i++) { result = pthread_join(threads[i], NULL); if (result != 0) { fprintf(stderr, "Error joining thread %d ", i); return 1; } } printf("Final counter value: %d ", shared_counter); return 0; }
在这个示例中,定义了一个全局的互斥锁mutex
和一个共享的计数器shared_counter
,在每个线程中,通过pthread_mutex_lock
和pthread_mutex_unlock
函数来加锁和解锁,确保对shared_counter
的访问是互斥的,从而保证计数器的正确性。
以上内容就是解答有关“linux线程编译”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1286959.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复