在Android平台上实现纸飞机功能,涉及自定义视图、动画处理和事件监听等多个技术点,以下是详细的步骤和代码示例:
一、自定义RelativeLayout
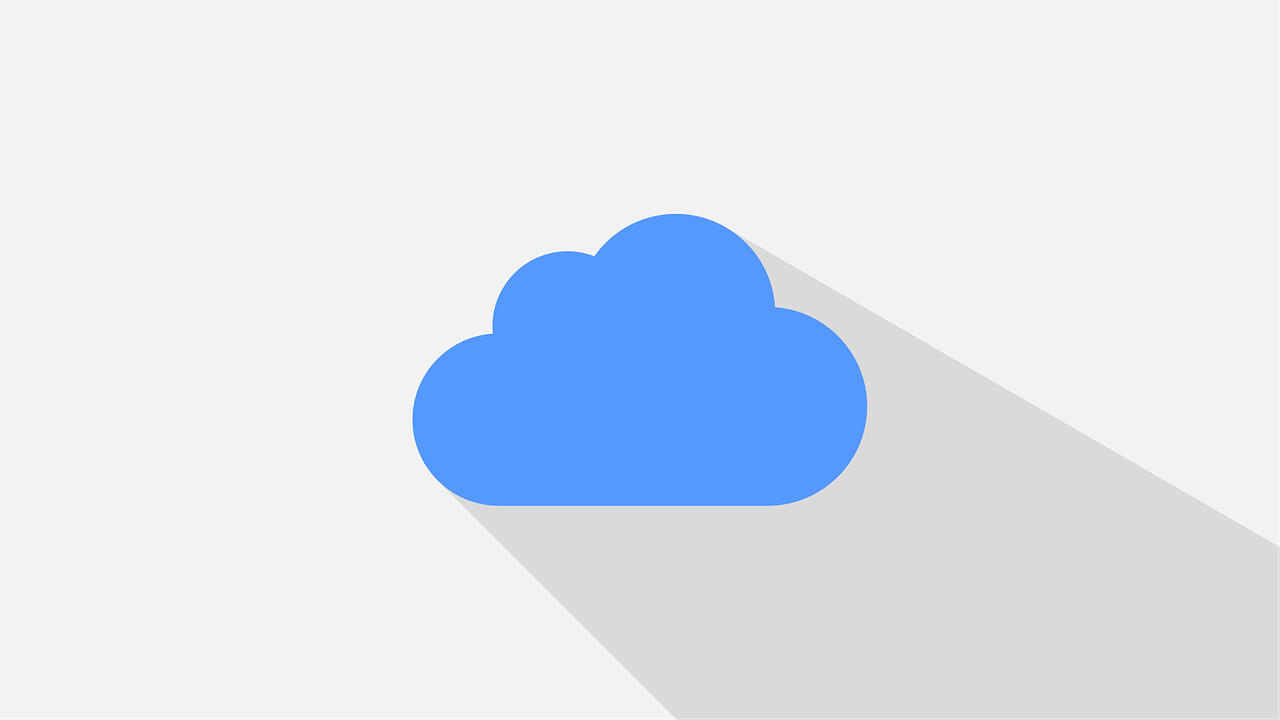
创建一个名为PaperPlaneLayout
的布局,它将负责纸飞机的动画展示,这个布局类继承自RelativeLayout
并实现了View.OnClickListener
接口,以便监听纸飞机的点击事件。
public class PaperPlaneLayout extends RelativeLayout implements View.OnClickListener{ private OnClickListener mOnClickListener; private int mHeight; private int mWidth; private LayoutParams lp; private Drawable[] drawables; private Random random = new Random(); private int dHeight; private int dWidth; private int mX; private int mY; public PaperPlaneLayout(Context context) { super(context); init(); } public PaperPlaneLayout(Context context, AttributeSet attrs) { super(context, attrs); init(); } public PaperPlaneLayout(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); init(); } @TargetApi(Build.VERSION_CODES.LOLLIPOP) public PaperPlaneLayout(Context context, AttributeSet attrs, int defStyleAttr, int defStyleRes) { super(context, attrs, defStyleAttr, defStyleRes); init(); } private void init() { drawables = new Drawable[4]; drawables[0] = getResources().getDrawable(R.drawable.pl_pink); drawables[1] = getResources().getDrawable(R.drawable.pl_yellow); drawables[2] = getResources().getDrawable(R.drawable.pl_green); drawables[3] = getResources().getDrawable(R.drawable.pl_blue); dHeight = UIUtility.dipTopx(getContext(), 80); dWidth = UIUtility.dipTopx(getContext(), 80); lp = new LayoutParams(dWidth, dHeight); } @Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { super.onMeasure(widthMeasureSpec, heightMeasureSpec); mWidth = getMeasuredWidth(); mHeight = getMeasuredHeight(); } public void addHeart(int x, int y, int position) { mX = x; mY = y; ImageView imageView = new ImageView(getContext()); imageView.setImageDrawable(drawables[position]); imageView.setLayoutParams(lp); addView(imageView); Animator set = getAnimator(imageView); set.start(); imageView.setOnClickListener(this); } @Override public void onClick(View v) { if (mOnClickListener != null) { mOnClickListener.onClick(v); } } private Animator getAnimator(View target) { AnimatorSet set = getEnterAnimator(target); AnimatorSet set2 = getLineAnimation(target); AnimatorSet finalSet = new AnimatorSet(); finalSet.playSequentially(set, set2); finalSet.setInterpolator(new LinearInterpolator()); finalSet.setTarget(target); return finalSet; } private AnimatorSet getEnterAnimator(final View target) { ObjectAnimator alpha = ObjectAnimator.ofFloat(target, View.ALPHA, 0.2f, 1f); ObjectAnimator translationX = ObjectAnimator.ofFloat(target, View.TRANSLATION_X, -mWidth, mX); AnimatorSet set = new AnimatorSet(); set.playTogether(alpha, translationX); set.setDuration(500); return set; } private AnimatorSet getLineAnimation(final View target) { ObjectAnimator translationY = ObjectAnimator.ofFloat(target, View.TRANSLATION_Y, mY, mY + 50); translationY.setRepeatCount(ValueAnimator.INFINITE); translationY.setRepeatMode(ValueAnimator.REVERSE); translationY.setDuration(1000); return translationY; } }
二、布局文件
在布局文件中使用自定义的PaperPlaneLayout
。
<com.example.PaperPlaneLayout android:layout_width="match_parent" android:layout_height="match_parent"/>
三、Activity中初始化和使用
在Activity中初始化PaperPlaneLayout
,并添加纸飞机。
public class MainActivity extends AppCompatActivity { private PaperPlaneLayout paperPlaneLayout; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); paperPlaneLayout = findViewById(R.id.paperPlaneLayout); paperPlaneLayout.addHeart(100, 200, 0); // 添加纸飞机到指定位置 } }
四、点击事件处理
当用户点击纸飞机时,触发onClick
方法,执行特定的飞行路径。
@Override public void onClick(View v) { // 暂停当前的入场动画,并执行一个相反的动画,让飞机向上飞出屏幕,再次从左侧飞回原位。 v.clearAnimation(); ObjectAnimator flyOut = ObjectAnimator.ofFloat(v, View.TRANSLATION_Y, mY, -mHeight); flyOut.setDuration(500); flyOut.addListener(new AnimatorListenerAdapter() { @Override public void onAnimationEnd(Animator animation) { super.onAnimationEnd(animation); // 当飞机回到原来位置时,弹出一个消息框。 new AlertDialog.Builder(getContext()).setTitle("纸飞机").setMessage("纸飞机已经返回!").show(); } }); flyOut.start(); }
五、云彩飘动效果
同样地,可以通过ObjectAnimator
来实现云彩从屏幕右侧向左侧飘动的动画。
private void startClouds() { ImageView cloud = new ImageView(this); cloud.setImageResource(R.drawable.cloud); RelativeLayout.LayoutParams params = new RelativeLayout.LayoutParams(RelativeLayout.LayoutParams.WRAP_CONTENT, RelativeLayout.LayoutParams.WRAP_CONTENT); params.addRule(RelativeLayout.ALIGN_PARENT_RIGHT); addView(cloud, params); ObjectAnimator cloudAnimator = ObjectAnimator.ofFloat(cloud, "translationX", mWidth, -cloud.getWidth()); cloudAnimator.setDuration(5000); cloudAnimator.setRepeatCount(ValueAnimator.INFINITE); cloudAnimator.start(); }
六、兼容性处理
对于不同版本的Android系统,可能需要使用@TargetApi
注解来确保在支持API 21及以上版本的设备上使用Lollipop及更高版本的特性。
各位小伙伴们,我刚刚为大家分享了有关“Android实现纸飞机”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1286334.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复