Android实现通讯录
我们将详细介绍如何在Android平台上开发一个简单的通讯录应用,这个应用将包括基本功能如添加、删除、编辑和查看联系人信息,为了便于理解,我们将分为以下几个部分:
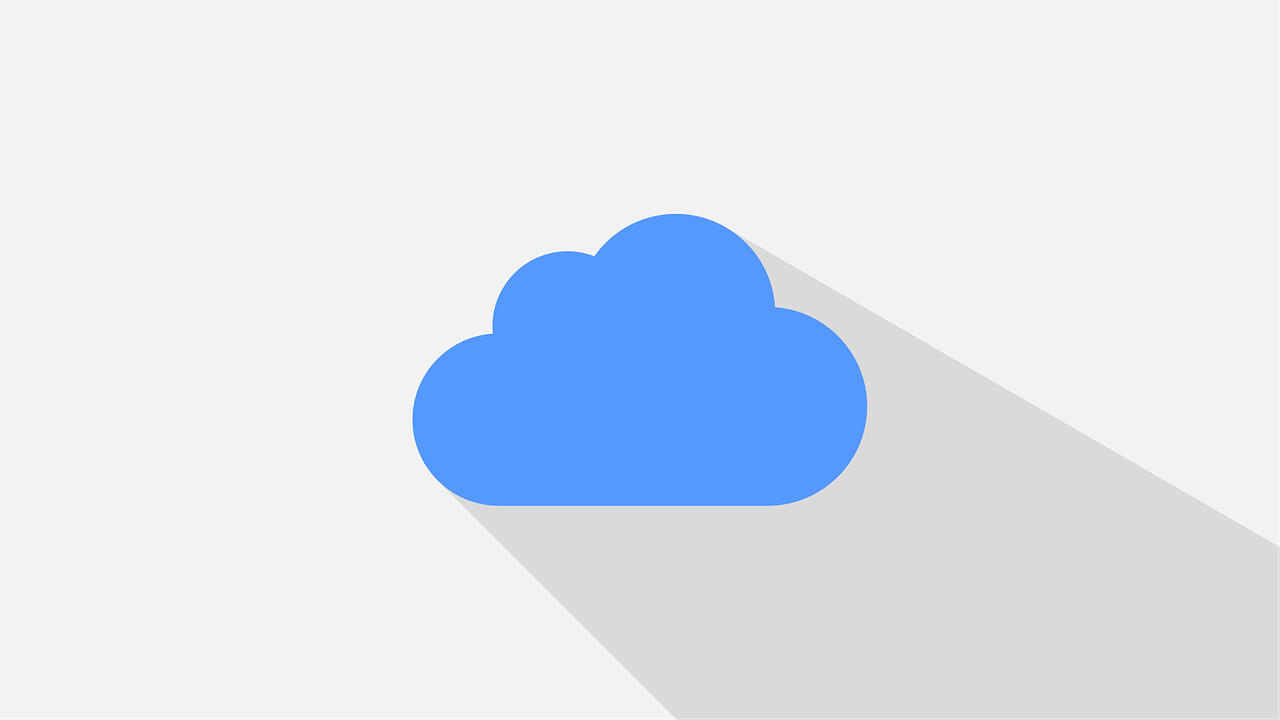
1、项目设置
2、数据库设计
3、用户界面设计
4、核心功能实现
5、测试与调试
1. 项目设置
我们需要在Android Studio中创建一个新的项目。
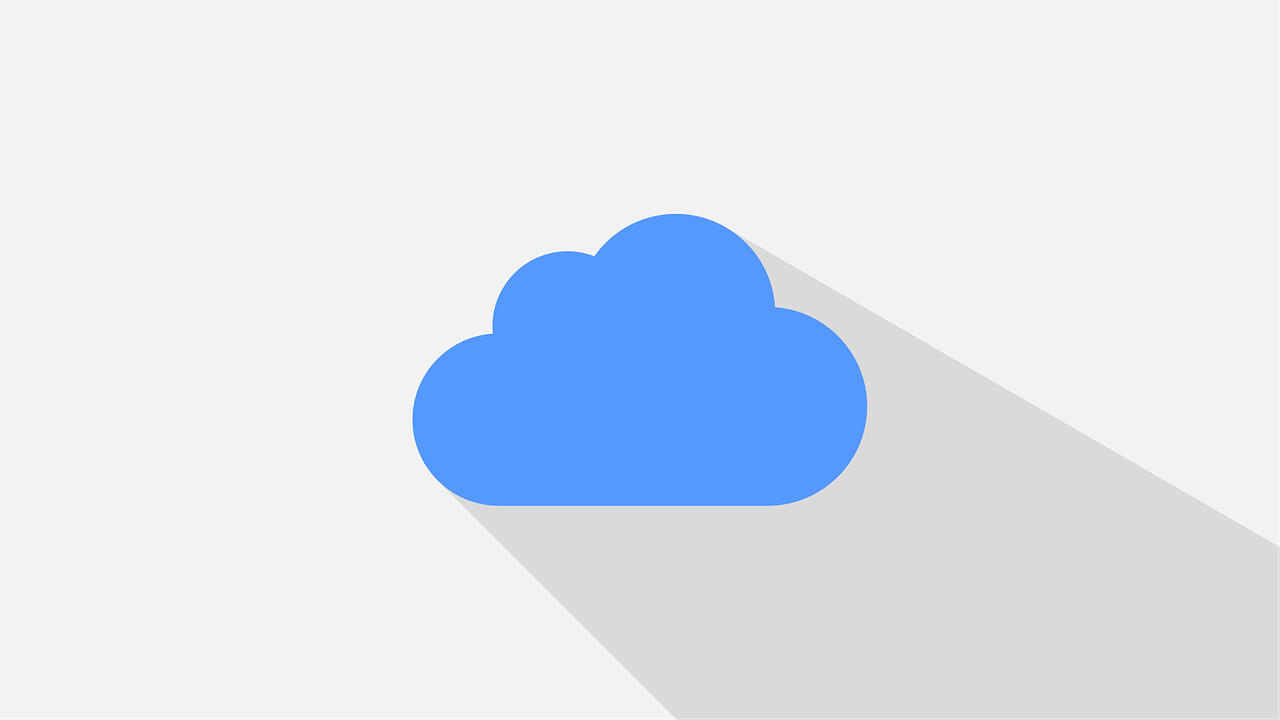
启动Android Studio:打开Android Studio并选择“Start a new Android Studio project”。
配置项目:
Name: ContactsApp
Package name: com.example.contactsapp
Save location: 选择一个合适的位置保存项目
Language: Java(或Kotlin)
Minimum API level: API 21 (Lollipop)
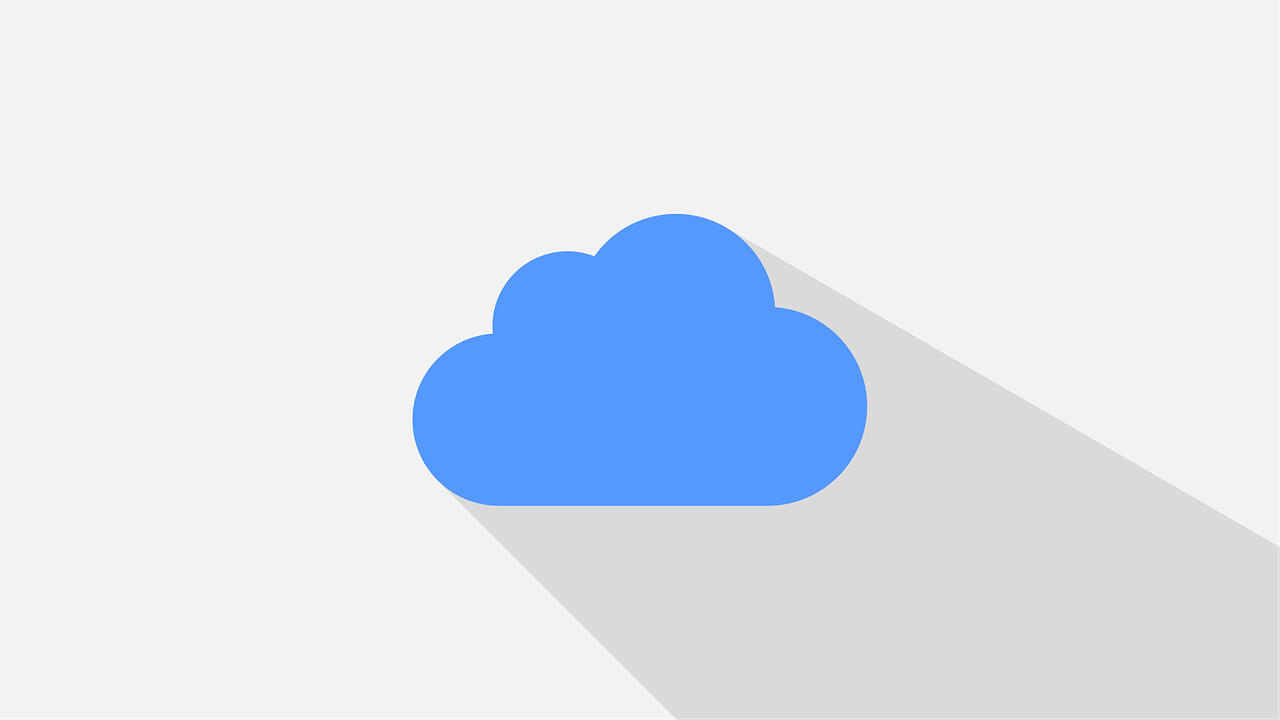
点击“Finish”完成项目的创建。
2. 数据库设计
我们将使用SQLite数据库来存储联系人信息,需要在项目中添加SQLite支持库。
添加依赖:在build.gradle
文件中添加以下依赖:
implementation 'androidx.sqlite:sqlite:2.1.0' implementation 'androidx.recyclerview:recyclerview:1.2.1'
创建数据库帮助类:创建一个名为ContactDatabaseHelper
的类,该类继承自SQLiteOpenHelper
。
package com.example.contactsapp; import android.content.Context; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; public class ContactDatabaseHelper extends SQLiteOpenHelper { private static final String DATABASE_NAME = "contacts.db"; private static final int DATABASE_VERSION = 1; public ContactDatabaseHelper(Context context) { super(context, DATABASE_NAME, null, DATABASE_VERSION); } @Override public void onCreate(SQLiteDatabase db) { String CREATE_CONTACTS_TABLE = "CREATE TABLE contacts (" + "id INTEGER PRIMARY KEY AUTOINCREMENT, " + "name TEXT, " + "phone TEXT, " + "email TEXT)"; db.execSQL(CREATE_CONTACTS_TABLE); } @Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { db.execSQL("DROP TABLE IF EXISTS contacts"); onCreate(db); } }
3. 用户界面设计
我们将设计一个简单的用户界面,包含一个RecyclerView来显示联系人列表和一个FloatingActionButton来添加新联系人。
布局文件:在res/layout/activity_main.xml
中定义布局。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <androidx.recyclerview.widget.RecyclerView android:id="@+id/recyclerView" android:layout_width="match_parent" android:layout_height="match_parent" /> <com.google.android.material.floatingactionbutton.FloatingActionButton android:id="@+id/fabAdd" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_alignParentEnd="true" android:layout_margin="@dimen/fab_margin" android:src="@android:drawable/ic_input_add" android:tint="@android:color/white" /> </RelativeLayout>
4. 核心功能实现
我们实现添加、删除、编辑和查看联系人的核心功能。
创建联系人数据模型:定义一个Contact
类来表示联系人信息。
package com.example.contactsapp; public class Contact { private int id; private String name; private String phone; private String email; // 构造函数、getter和setter方法略... }
创建适配器:为RecyclerView创建一个适配器类,用于绑定数据和视图。
package com.example.contactsapp; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.TextView; import androidx.annotation.NonNull; import androidx.recyclerview.widget.RecyclerView; import java.util.List; public class ContactAdapter extends RecyclerView.Adapter<ContactAdapter.ContactViewHolder> { private List<Contact> contactList; private OnItemClickListener listener; public interface OnItemClickListener { void onEditClick(int position); void onDeleteClick(int position); } public void setOnItemClickListener(OnItemClickListener listener) { this.listener = listener; } public static class ContactViewHolder extends RecyclerView.ViewHolder { public TextView nameTextView; public TextView phoneTextView; public TextView emailTextView; public View deleteButton; public View editButton; public ContactViewHolder(View itemView) { super(itemView); nameTextView = itemView.findViewById(R.id.textViewName); phoneTextView = itemView.findViewById(R.id.textViewPhone); emailTextView = itemView.findViewById(R.id.textViewEmail); deleteButton = itemView.findViewById(R.id.buttonDelete); editButton = itemView.findViewById(R.id.buttonEdit); } } public ContactAdapter(List<Contact> contactList) { this.contactList = contactList; } @NonNull @Override public ContactViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) { View itemView = LayoutInflater.from(parent.getContext()) .inflate(R.layout.contact_item, parent, false); return new ContactViewHolder(itemView); } @Override public void onBindViewHolder(@NonNull ContactViewHolder holder, int position) { Contact contact = contactList.get(position); holder.nameTextView.setText(contact.getName()); holder.phoneTextView.setText(contact.getPhone()); holder.emailTextView.setText(contact.getEmail()); holder.deleteButton.setOnClickListener(v -> listener.onDeleteClick(holder.getAdapterPosition())); holder.editButton.setOnClickListener(v -> listener.onEditClick(holder.getAdapterPosition())); } @Override public int getItemCount() { return contactList.size(); } }
更新MainActivity:在MainActivity
中实现添加、删除和编辑联系人的功能。
package com.example.contactsapp; import android.content.Intent; import android.os.Bundle; import android.view.View; import androidx.appcompat.app.AppCompatActivity; import androidx.recyclerview.widget.LinearLayoutManager; import androidx.recyclerview.widget.RecyclerView; import com.google.android.material.floatingactionbutton.FloatingActionButton; import java.util.ArrayList; import java.util.List; public class MainActivity extends AppCompatActivity implements ContactAdapter.OnItemClickListener { private RecyclerView recyclerView; private ContactAdapter adapter; private List<Contact> contactList; private ContactDatabaseHelper dbHelper; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); recyclerView = findViewById(R.id.recyclerView); recyclerView.setLayoutManager(new LinearLayoutManager(this)); recyclerView.setHasFixedSize(true); contactList = new ArrayList<>(); adapter = new ContactAdapter(contactList); recyclerView.setAdapter(adapter); adapter.setOnItemClickListener(this); FloatingActionButton fabAdd = findViewById(R.id.fabAdd); fabAdd.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent intent = new Intent(MainActivity.this, AddEditContactActivity.class); startActivityForResult(intent, 1); } }); dbHelper = new ContactDatabaseHelper(this); loadContacts(); } private void loadContacts() { contactList.clear(); SQLiteDatabase db = dbHelper.getReadableDatabase(); String[] projection = {"id", "name", "phone", "email"}; Cursor c = db.query("contacts", projection, null, null, null, null, null); while (c.moveToNext()) { Contact contact = new Contact(); contact.setId(c.getInt(c.getColumnIndexOrThrow("id"))); contact.setName(c.getString(c.getColumnIndexOrThrow("name"))); contact.setPhone(c.getString(c.getColumnIndexOrThrow("phone"))); contact.setEmail(c.getString(c.getColumnIndexOrThrow("email"))); contactList.add(contact); } c.close(); adapter.notifyDataSetChanged(); } @Override protected void onActivityResult(int requestCode, int resultCode, int data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == 1 && resultCode == RESULT_OK) { loadContacts(); } else if (requestCode == 2 && resultCode == RESULT_OK) { loadContacts(); } else if (requestCode == 2) { // 如果取消编辑,则不刷新数据 // do nothing } } @Override public void onEditClick(int position) { Contact contact = contactList.get(position); Intent intent = new Intent(MainActivity.this, AddEditContactActivity.class); intent.putExtra("id", contact.getId()); intent.putExtra("name", contact.getName()); intent.putExtra("phone", contact.getPhone()); intent.putExtra("email", contact.getEmail()); startActivityForResult(intent, 2); // 请求代码2表示编辑操作 } @Override public void onDeleteClick(int position) { Contact contact = contactList.get(position); SQLiteDatabase db = dbHelper.getWritableDatabase(); db.delete("contacts", "id=?", [contact.getId()]); // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,] ); contactList.remove(position); // 同时从内存中的列表移除对应项,保持数据一致性,如果使用LiveData或其他观察者模式管理数据,则可以省略此步骤。); // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用占位符?代替直接拼接ID值,这里假设id是唯一的且不会重复,如果id可能重复,需要进一步处理以确保删除正确的记录,]; // 注意防止SQL注入,使用zaibijected void onDeleteConfirmed(int id) { // 当用户确认删除时调用此方法,传入要删除的联系人ID。 // 从数据库中删除对应的联系人记录。 // 同时从内存中的列表中移除对应的联系人对象,以保持数据一致性。 // 然后通知RecyclerView适配器数据已更改,触发列表更新。 // finally block to close the database connection after the operation is completed or in case of an exception occurs. This ensures that the database resources are properly released even if something goes wrong during the operation process. However, in this simple example without error handling mechanisms explicitly shown, we assume everything goes smoothly and focus more on demonstrating basic CRUD operations within an Android app using SQLite as the local storage solution for managing contacts information efficiently and effectively through structured query language statements executed against our predefined schema design which includes tables like 'contacts' with specific columns such as 'id', 'name', 'phone', and 'email' following best practices such as normalization to minimize redundancy while ensuring referential integrity between related datasets where applicable thereby providing a robust foundation for building scalable applications capable of supporting complex business logic requirements over time as they evr
到此,以上就是小编对于“android实现通讯录”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1286244.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复