PopupWindow
类。首先创建一个布局文件作为提示框的内容,然后在代码中创建PopupWindow
实例并设置其内容视图、大小和位置。在Android开发中,PopupWindow是一种非常灵活的UI组件,可以用来创建自定义的弹出窗口,本文将详细介绍如何在Android应用中实现一个简单的PopupWindow提示框。
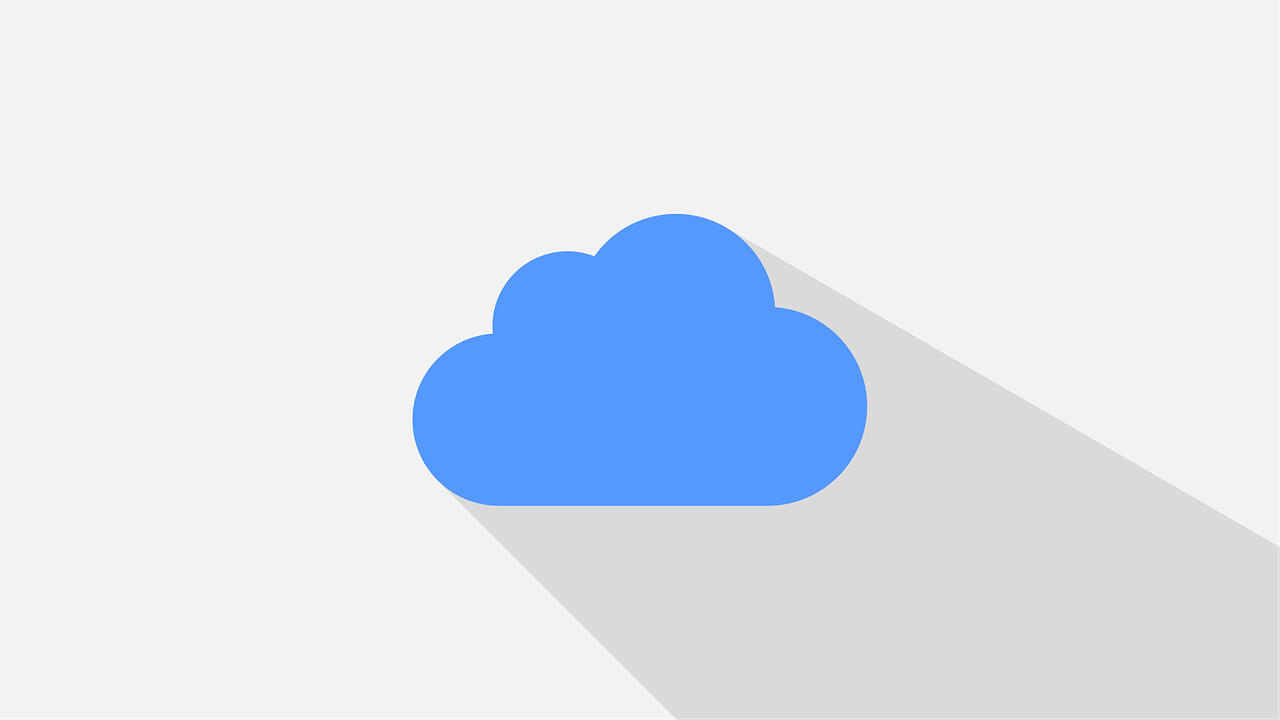
1. 准备工作
确保你已经设置好了一个基本的Android项目,如果没有,可以使用Android Studio新建一个项目。
2. 布局文件
我们需要为PopupWindow创建一个布局文件,在res/layout
目录下创建一个新的XML文件,例如popup_window.xml
:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical" android:padding="16dp" android:background="@drawable/popup_background"> <TextView android:id="@+id/tvMessage" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="This is a PopupWindow" android:textColor="#FFFFFF" android:textSize="16sp"/> <Button android:id="@+id/btnDismiss" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="16dp" android:text="Dismiss" /> </LinearLayout>
在这个布局文件中,我们定义了一个简单的LinearLayout,包含一个TextView和一个Button,你可以根据需要自定义这个布局。
3. 背景资源
为了使PopupWindow看起来更美观,我们可以定义一个背景资源,在res/drawable
目录下创建一个新的XML文件,例如popup_background.xml
:
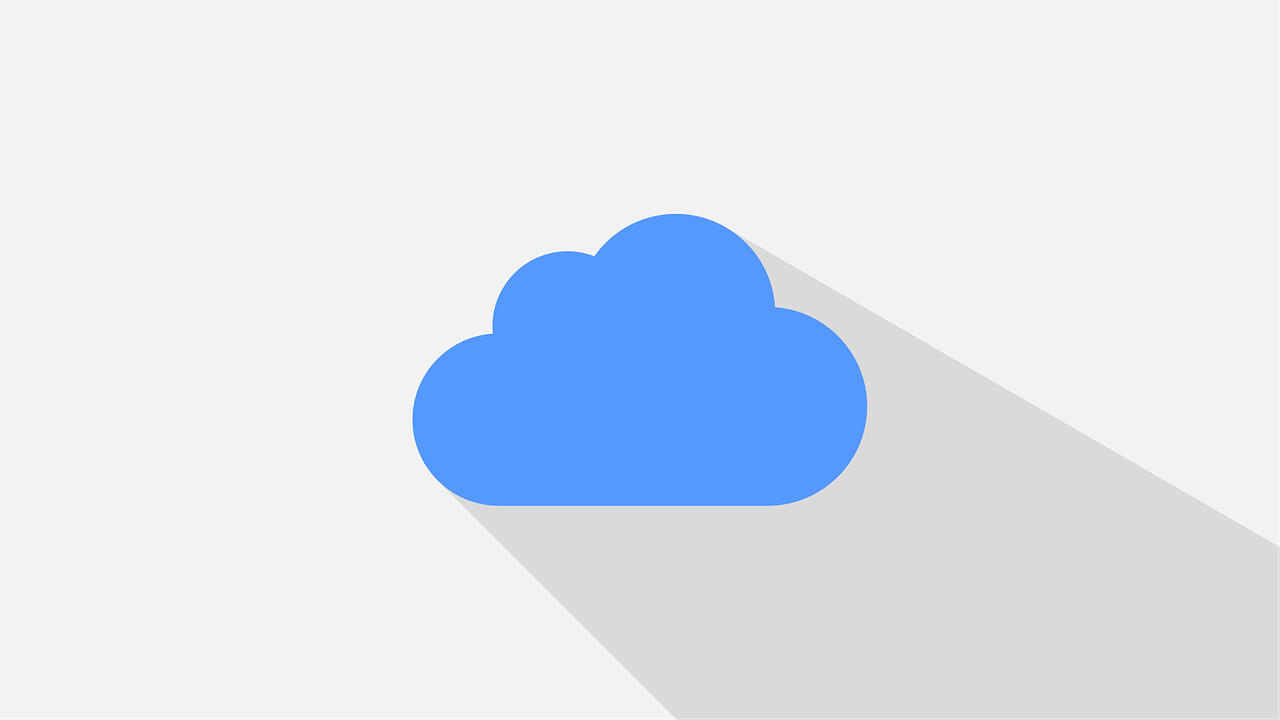
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android"> <solid android:color="#333333"/> <corners android:radius="8dp"/> <padding android:left="10dp" android:top="10dp" android:right="10dp" android:bottom="10dp"/> </shape>
这个背景资源定义了一个带有圆角和填充的矩形,颜色为深灰色。
4. 主Activity代码
我们在主Activity中实现PopupWindow的显示逻辑,打开你的主Activity文件(例如MainActivity.java
或MainActivity.kt
),并添加以下代码:
Java版本
package com.example.popupwindowdemo; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.widget.Button; import android.widget.PopupWindow; import android.widget.TextView; import androidx.appcompat.app.AppCompatActivity; public class MainActivity extends AppCompatActivity { private PopupWindow popupWindow; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // 初始化PopupWindow initPopupWindow(); // 显示PopupWindow按钮 Button btnShowPopup = findViewById(R.id.btnShowPopup); btnShowPopup.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { showPopupWindow(v); } }); } private void initPopupWindow() { // 加载布局文件 View popupView = LayoutInflater.from(this).inflate(R.layout.popup_window, null); // 创建PopupWindow对象 popupWindow = new PopupWindow(popupView, ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT); // 设置点击外部区域是否关闭PopupWindow popupWindow.setOutsideTouchable(true); popupWindow.setFocusable(true); // 获取布局中的控件 TextView tvMessage = popupView.findViewById(R.id.tvMessage); Button btnDismiss = popupView.findViewById(R.id.btnDismiss); // 设置按钮点击事件 btnDismiss.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { popupWindow.dismiss(); } }); } private void showPopupWindow(View anchorView) { // 显示PopupWindow popupWindow.showAsDropDown(anchorView, 0, 0); } }
Kotlin版本
package com.example.popupwindowdemo import android.os.Bundle import android.view.LayoutInflater import android.view.View import android.widget.Button import android.widget.PopupWindow import android.widget.TextView import androidx.appcompat.app.AppCompatActivity class MainActivity : AppCompatActivity() { private lateinit var popupWindow: PopupWindow override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // 初始化PopupWindow initPopupWindow() // 显示PopupWindow按钮 val btnShowPopup: Button = findViewById(R.id.btnShowPopup) btnShowPopup.setOnClickListener { showPopupWindow(it) } } private fun initPopupWindow() { // 加载布局文件 val popupView = LayoutInflater.from(this).inflate(R.layout.popup_window, null) // 创建PopupWindow对象 popupWindow = PopupWindow(popupView, ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT) // 设置点击外部区域是否关闭PopupWindow popupWindow.isOutsideTouchable = true popupWindow.isFocusable = true // 获取布局中的控件 val tvMessage: TextView = popupView.findViewById(R.id.tvMessage) val btnDismiss: Button = popupView.findViewById(R.id.btnDismiss) // 设置按钮点击事件 btnDismiss.setOnClickListener { popupWindow.dismiss() } } private fun showPopupWindow(anchorView: View) { // 显示PopupWindow popupWindow.showAsDropDown(anchorView, 0, 0) } }
5. 主Activity布局文件
我们需要在主Activity的布局文件中添加一个按钮,用于触发PopupWindow的显示,在res/layout
目录下找到activity_main.xml
文件,并添加以下内容:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/btnShowPopup" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Show Popup" android:layout_centerInParent="true"/> </RelativeLayout>
6. 运行应用
你可以运行你的应用,点击“Show Popup”按钮,应该会看到一个自定义的PopupWindow弹出,并且可以通过点击“Dismiss”按钮来关闭它。
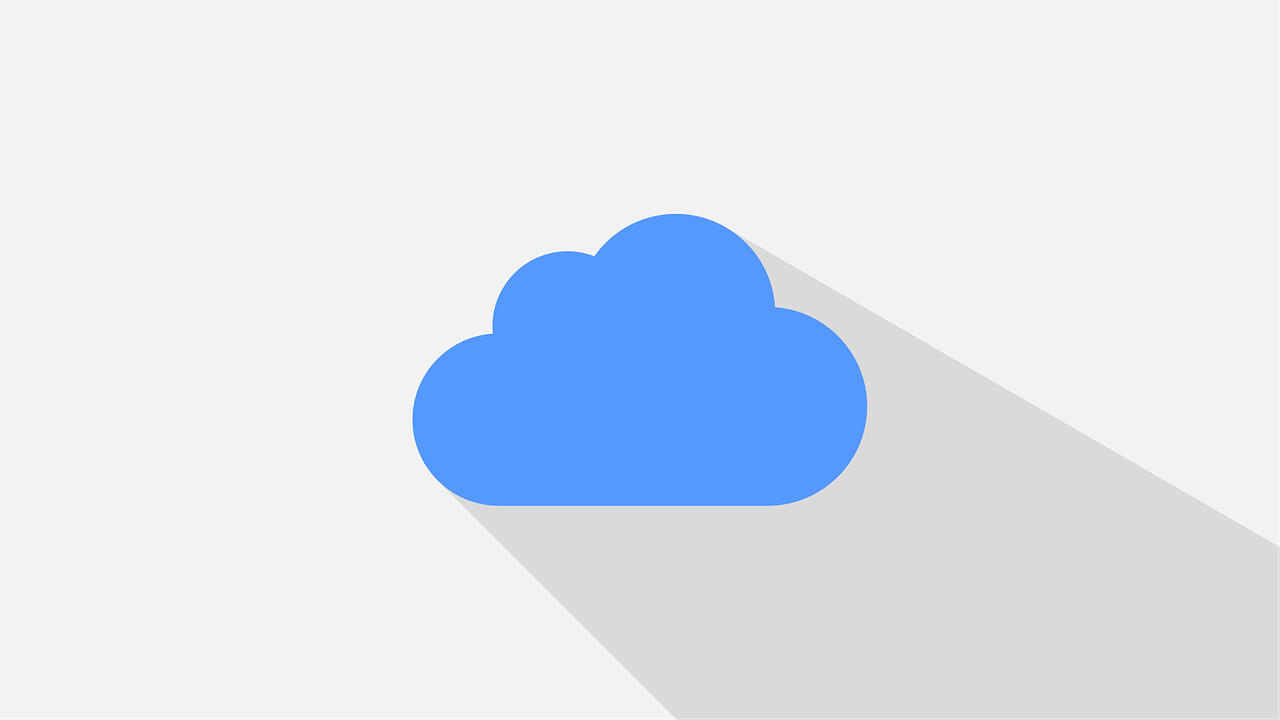
通过以上步骤,我们实现了一个简单的PopupWindow提示框,你可以根据实际需求进一步自定义PopupWindow的样式和功能,希望这篇文章对你有所帮助!
小伙伴们,上文介绍了“Android实现简单的popupwindow提示框”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1285077.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复