Android实现起始点位置交换
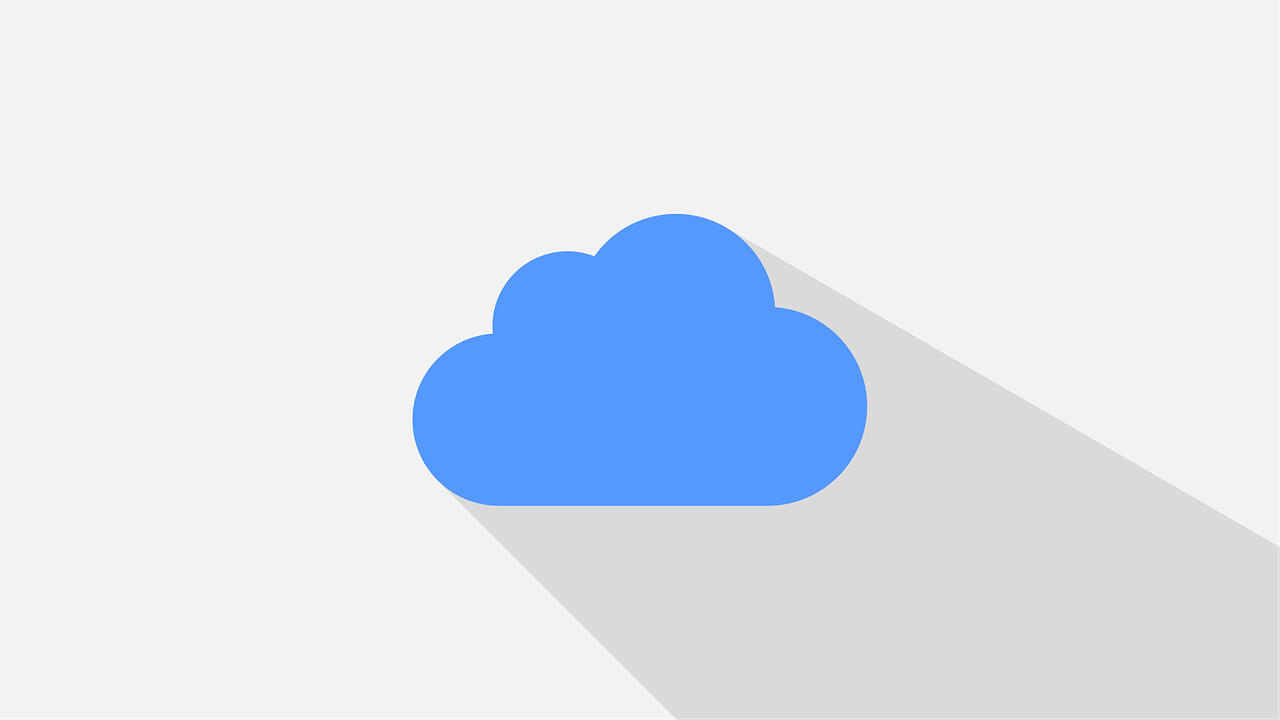
在Android开发中,有时需要实现两个视图(如TextView)的位置交换,这种功能常见于购票应用中的起始点和终点的交换,本文将详细介绍如何在Android中实现这一功能,包括布局文件的设计、关键步骤以及完整的代码示例。
一、布局文件设计
我们需要设计一个包含两个TextView和一个Button的布局文件,以下是一个简单的XML布局文件:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:fitsSystemWindows="true" android:orientation="horizontal"> <TextView android:id="@+id/left_tv" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:gravity="center" android:text="北京" /> <Button android:id="@+id/btn" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:gravity="center" android:text="交换位置" /> <TextView android:id="@+id/right_tv" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:gravity="center" android:text="深圳" /> </LinearLayout>
在这个布局文件中,我们定义了一个水平线性布局,其中包含两个TextView和一个Button,每个TextView的宽度占整个布局的一半,Button位于中间。
二、关键步骤
要实现位置交换功能,我们需要以下几个关键步骤:
1、获取初始位置:在Activity启动时,获取两个TextView的初始位置。
2、设置动画:使用ValueAnimator来创建动画,使两个TextView的位置逐渐交换。
3、启动动画:点击Button时,启动动画。
三、完整代码示例
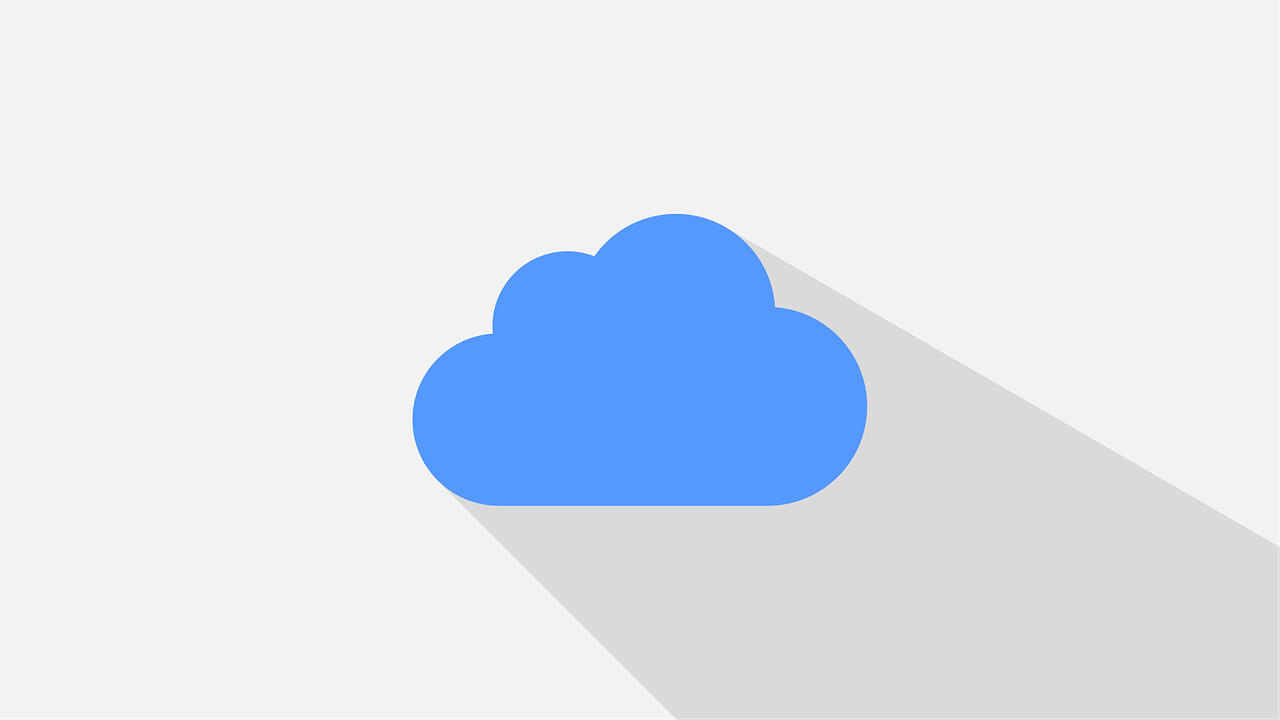
以下是一个完整的Java代码示例,展示了如何实现上述功能:
package com.example.testbutton; import android.animation.ValueAnimator; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; import androidx.appcompat.app.AppCompatActivity; public class TESTButtonActivity extends AppCompatActivity { private int startX; private int endX; private TextView leftCityTextView; private TextView rightCityTextView; private ValueAnimator endCityAnimator; private ValueAnimator startCityAnimation; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_button); leftCityTextView = findViewById(R.id.left_tv); rightCityTextView = findViewById(R.id.right_tv); Button mBtn = findViewById(R.id.btn); // 获取初始位置 getLocation(); mBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { startCityAnimation.start(); endCityAnimator.start(); } }); } private void getLocation() { int[] startXLocation = new int[2]; leftCityTextView.getLocationOnScreen(startXLocation); // 获取坐标 int[] endXLocation = new int[2]; rightCityTextView.getLocationOnScreen(endXLocation); startX = startXLocation[0]; // 0为x坐标 endX = endXLocation[0]; } @Override public void onWindowFocusChanged(boolean hasFocus) { super.onWindowFocusChanged(hasFocus); getLocation(); int leftMoveX = endX startX; int rightMoveX = endX startX; startCityAnimation = ValueAnimator.ofInt(0, leftMoveX).setDuration(5000); startCityAnimation.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() { @Override public void onAnimationUpdate(ValueAnimator animation) { int value = (int) animation.getAnimatedValue(); // 重新布局 leftCityTextView.layout(startX + value, leftCityTextView.getTop(), startX + value + leftCityTextView.getWidth(), leftCityTextView.getBottom()); } }); endCityAnimator = ValueAnimator.ofInt(0, rightMoveX).setDuration(5000); endCityAnimator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() { @Override public void onAnimationUpdate(ValueAnimator animation) { int value = (int) animation.getAnimatedValue(); // 重新布局 rightCityTextView.layout(endX value, rightCityTextView.getTop(), endX + rightCityTextView.getWidth() value, rightCityTextView.getBottom()); } }); } }
通过以上步骤和代码示例,我们实现了在Android中两个TextView的位置交换功能,关键在于获取初始位置、设置动画并在点击Button时启动动画,这种方法不仅可以用于TextView,还可以扩展到其他类型的视图,满足不同的需求。
各位小伙伴们,我刚刚为大家分享了有关“android实现起始点位置交换”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1284778.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复