实现Android购物车添加商品动画
在现代电商应用中,用户体验是一个非常重要的方面,当用户将商品添加到购物车时,一个流畅且吸引人的动画可以大大提升用户体验,本文将介绍如何在Android应用中实现购物车添加商品的动画效果。
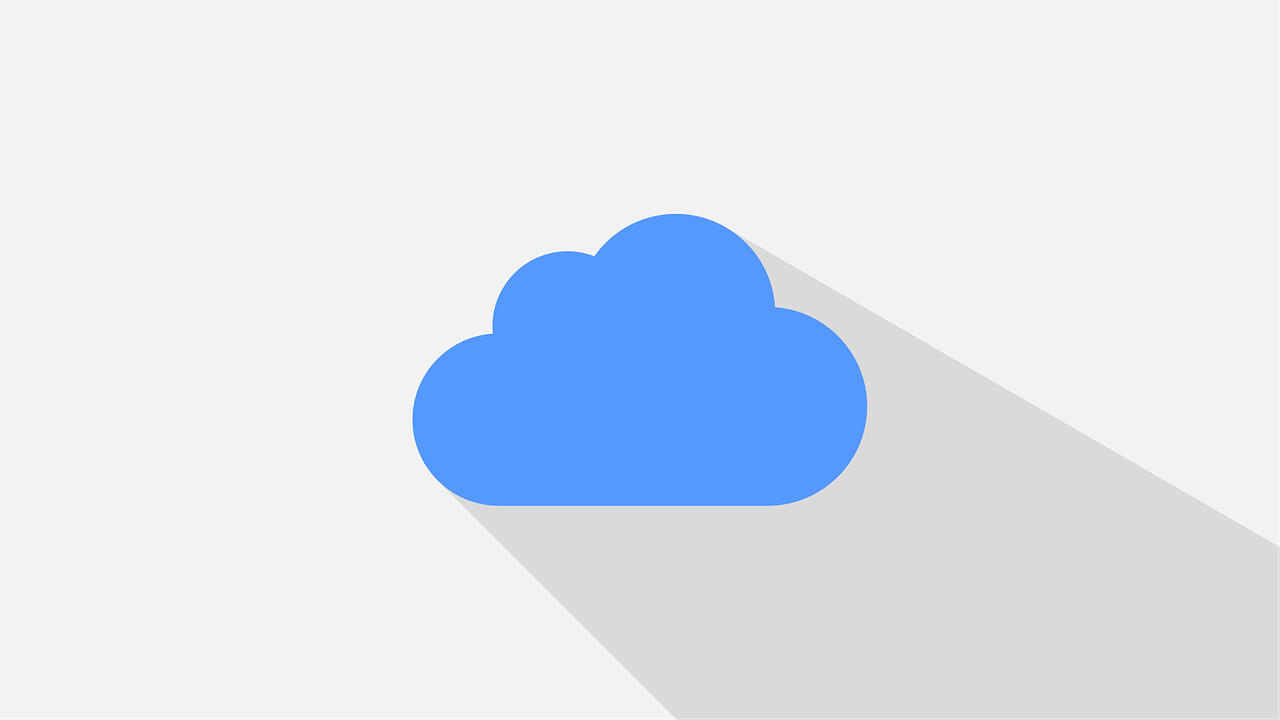
1. 准备工作
确保你已经设置好了一个基本的Android项目,并且已经创建了必要的布局和活动,在这个例子中,我们假设你有一个RecyclerView
来显示商品列表,以及一个FloatingActionButton
(FAB)用于添加商品到购物车。
项目结构概览:
activity_main.xml
: 主活动布局,包含RecyclerView
和FloatingActionButton
item_product.xml
: 商品项布局
ProductAdapter.java
: 商品适配器类
MainActivity.java
: 主活动类
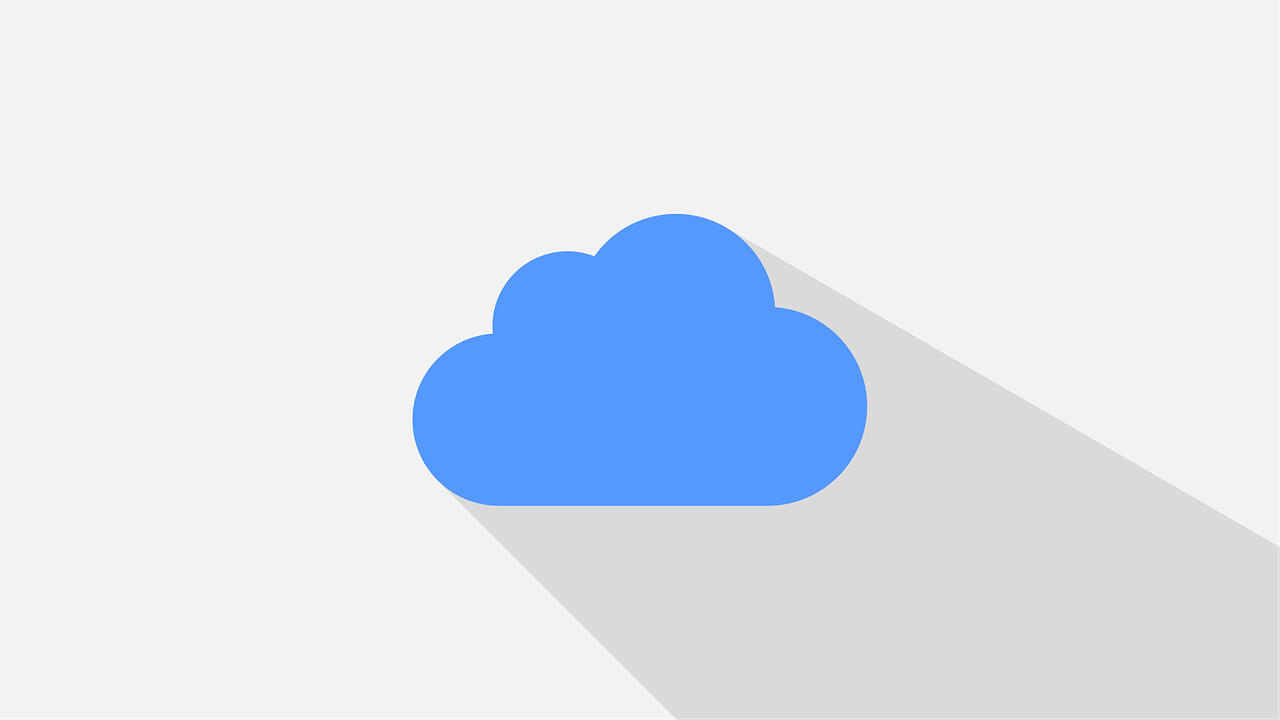
2. 更新布局文件
activity_main.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent"> <androidx.recyclerview.widget.RecyclerView android:id="@+id/recyclerView" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_above="@+id/fab" /> <com.google.android.material.floatingactionbutton.FloatingActionButton android:id="@+id/fab" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_alignParentEnd="true" android:layout_margin="@dimen/fab_margin" android:src="@drawable/ic_add_shopping_cart_black_24dp" app:tint="@android:color/white" /> </RelativeLayout>
item_product.xml:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:padding="8dp"> <ImageView android:id="@+id/productImage" android:layout_width="50dp" android:layout_height="50dp" android:src="@drawable/ic_launcher_background" /> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical" android:layout_marginStart="8dp" android:layout_gravity="center_vertical"> <TextView android:id="@+id/productName" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Product Name" android:textSize="16sp" /> <TextView android:id="@+id/productPrice" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="$9.99" android:textSize="14sp" /> </LinearLayout> </LinearLayout>
3. 创建商品适配器类
ProductAdapter.java:
import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ImageView; import android.widget.TextView; import androidx.annotation.NonNull; import androidx.recyclerview.widget.RecyclerView; import java.util.List; public class ProductAdapter extends RecyclerView.Adapter<ProductAdapter.ProductViewHolder> { private List<Product> productList; public static class ProductViewHolder extends RecyclerView.ViewHolder { public ImageView productImage; public TextView productName; public TextView productPrice; public ProductViewHolder(View view) { super(view); productImage = view.findViewById(R.id.productImage); productName = view.findViewById(R.id.productName); productPrice = view.findViewById(R.id.productPrice); } } public ProductAdapter(List<Product> productList) { this.productList = productList; } @NonNull @Override public ProductViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) { View itemView = LayoutInflater.from(parent.getContext()) .inflate(R.layout.item_product, parent, false); return new ProductViewHolder(itemView); } @Override public void onBindViewHolder(@NonNull ProductViewHolder holder, int position) { Product product = productList.get(position); holder.productImage.setImageResource(product.getImage()); holder.productName.setText(product.getName()); holder.productPrice.setText("$" + product.getPrice()); } @Override public int getItemCount() { return productList.size(); } }
4. 更新主活动类
MainActivity.java:
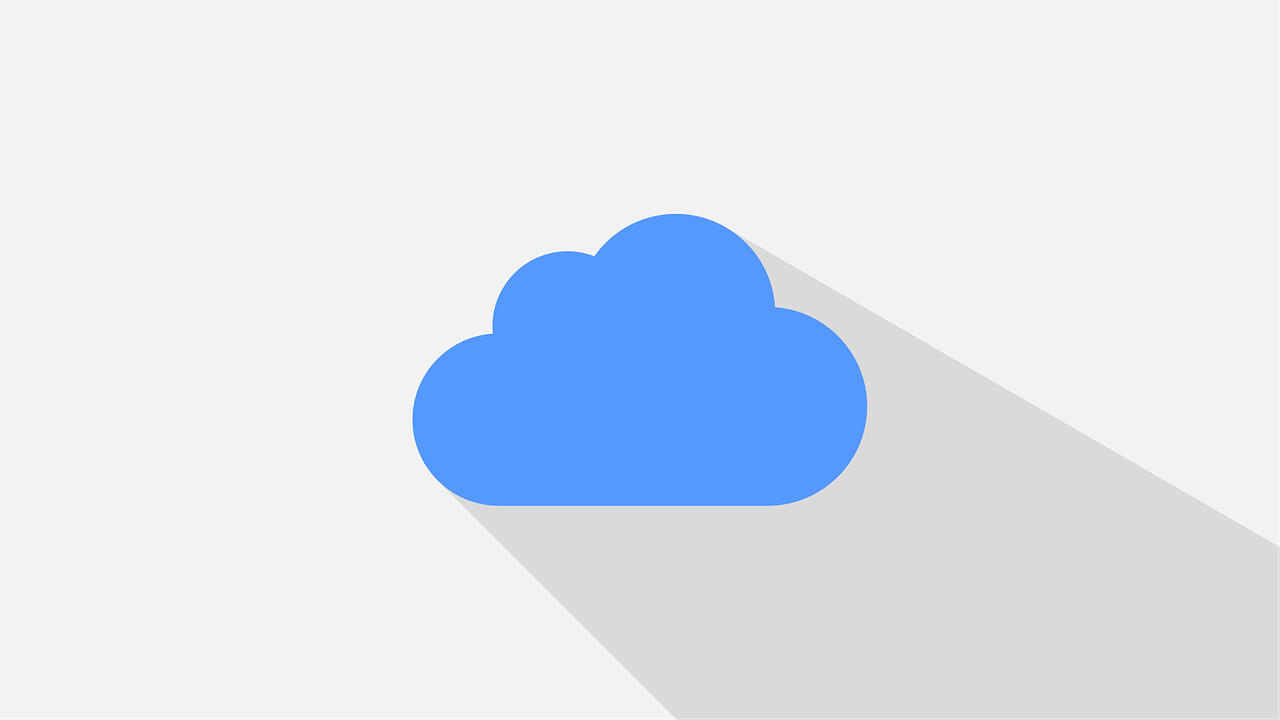
import android.os.Bundle; import android.view.View; import android.widget.Toast; import androidx.appcompat.app.AppCompatActivity; import androidx.recyclerview.widget.LinearLayoutManager; import androidx.recyclerview.widget.RecyclerView; import com.google.android.material.floatingactionbutton.FloatingActionButton; import java.util.ArrayList; import java.util.List; public class MainActivity extends AppCompatActivity { private RecyclerView recyclerView; private ProductAdapter adapter; private List<Product> productList; private FloatingActionButton fabAddToCart; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); recyclerView = findViewById(R.id.recyclerView); fabAddToCart = findViewById(R.id.fab); // 初始化商品列表 productList = new ArrayList<>(); // 添加一些示例商品 for (int i = 1; i <= 20; i++) { productList.add(new Product("Product " + i, "Description for product " + i, R.drawable.ic_launcher_background, i * 2.99)); } adapter = new ProductAdapter(productList); recyclerView.setAdapter(adapter); recyclerView.setLayoutManager(new LinearLayoutManager(this)); // 为FAB设置点击事件监听器 fabAddToCart.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { addToCartAnimation(); } }); } private void addToCartAnimation() { // 获取当前选中的商品位置(这里简单模拟为第一个商品) int selectedPosition = 0; // 实际应用中可以通过某种方式获取用户选择的商品位置 Product selectedProduct = productList.get(selectedPosition); // 显示一个简单的Toast消息,表示商品已添加到购物车中 Toast.makeText(this, selectedProduct.getName() + " added to cart!", Toast.LENGTH_SHORT).show(); } }
5. 创建商品类
Product.java:
public class Product { private String name; private String description; private int image; private double price; public Product(String name, String description, int image, double price) { this.name = name; this.description = description; this.image = image; this.price = price; } public String getName() { return name; } public String getDescription() { return description; } public int getImage() { return image; } public double getPrice() { return price; } }
6. 添加动画效果(可选)
为了使添加商品到购物车的动画更加生动,可以使用Android的动画框架,可以使用ObjectAnimator
来实现一个简单的缩放动画。
修改MainActivity中的addToCartAnimation方法:
private void addToCartAnimation() { // 获取当前选中的商品位置(这里简单模拟为第一个商品) int selectedPosition = 0; // 实际应用中可以通过某种方式获取用户选择的商品位置 Product selectedProduct = productList.get(selectedPosition); // 显示一个简单的Toast消息,表示商品已添加到购物车中 Toast.makeText(this, selectedProduct.getName() + " added to cart!", Toast.LENGTH_SHORT).show(); // 执行缩放动画(以第一个商品为例) View firstItemView = recyclerView.getChildAt(0); // 获取第一个可见的商品视图 if (firstItemView != null) { firstItemView.animate() .scaleX(1.1f) // 放大X轴方向上的尺寸到110% .scaleY(1.1f) // 放大Y轴方向上的尺寸到110% .setDuration(200) // 动画持续时间为200毫秒 .withEndAction(new Runnable() { // 动画结束后的动作 @Override public void run() { firstItemView.animate() // 恢复到原始大小 .scaleX(1f) // X轴方向恢复到100% .scaleY(1f) // Y轴方向恢复到100% .setDuration(200); // 动画持续时间为200毫秒 } }) .start(); // 开始动画 } }
这个简单的动画会在商品被添加到购物车时放大商品视图,并在一段时间后恢复到原始大小,从而给用户带来视觉上的反馈,你可以根据需要进一步自定义动画效果,比如改变颜色、透明度等。
以上内容就是解答有关“Android实现购物车添加商品动画”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1284497.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复