Android实现社交应用中评论与回复功能
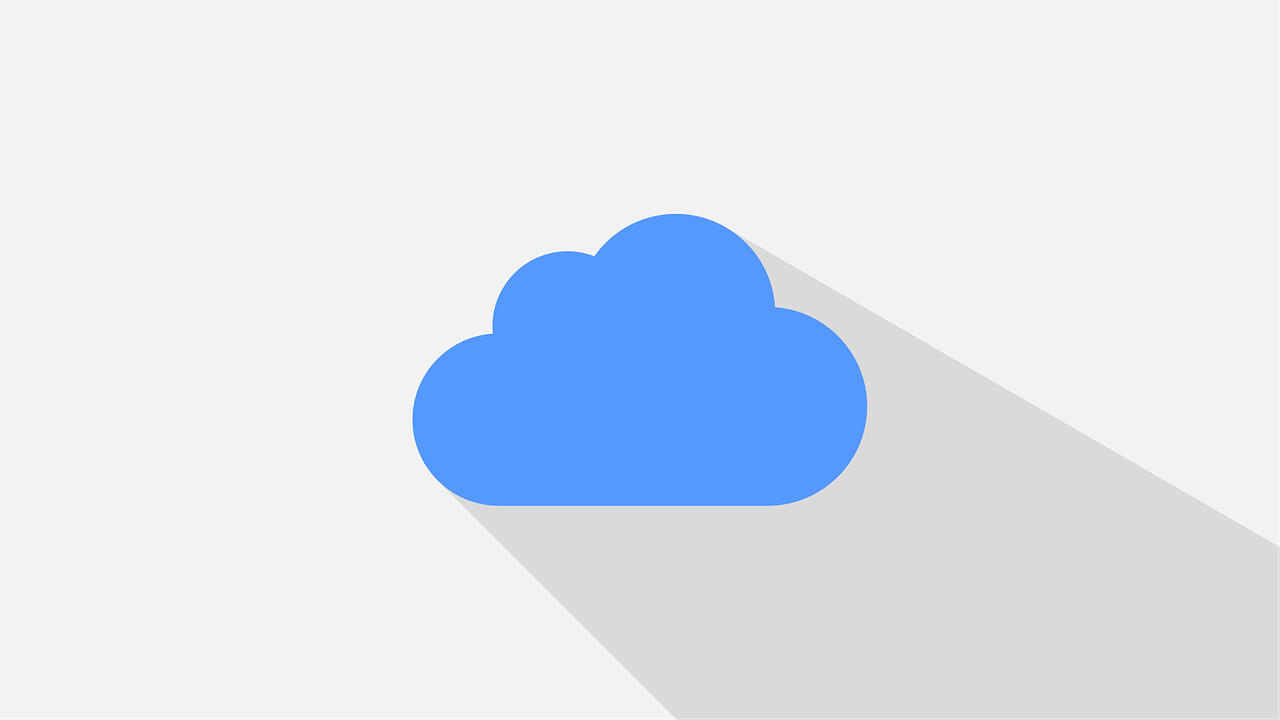
在Android平台上开发一个具备评论和回复功能的社交应用程序,需要结合UI设计、数据存储、网络通信等多方面的技术,本文将详细阐述如何实现这一功能,包括界面设计、数据模型、业务逻辑以及网络请求等关键部分。
一、项目
目标
创建一个能够发布评论,并针对特定评论进行回复的社交应用模块,用户可以通过该模块查看他人的评论,并对感兴趣的评论做出回应。
技术栈
编程语言:Java/Kotlin
数据库:Room(本地缓存)+ Firebase Firestore/Realtime Database(云端同步)
网络库:Retrofit或OkHttp
UI框架:Jetpack Compose或XML布局+View Binding
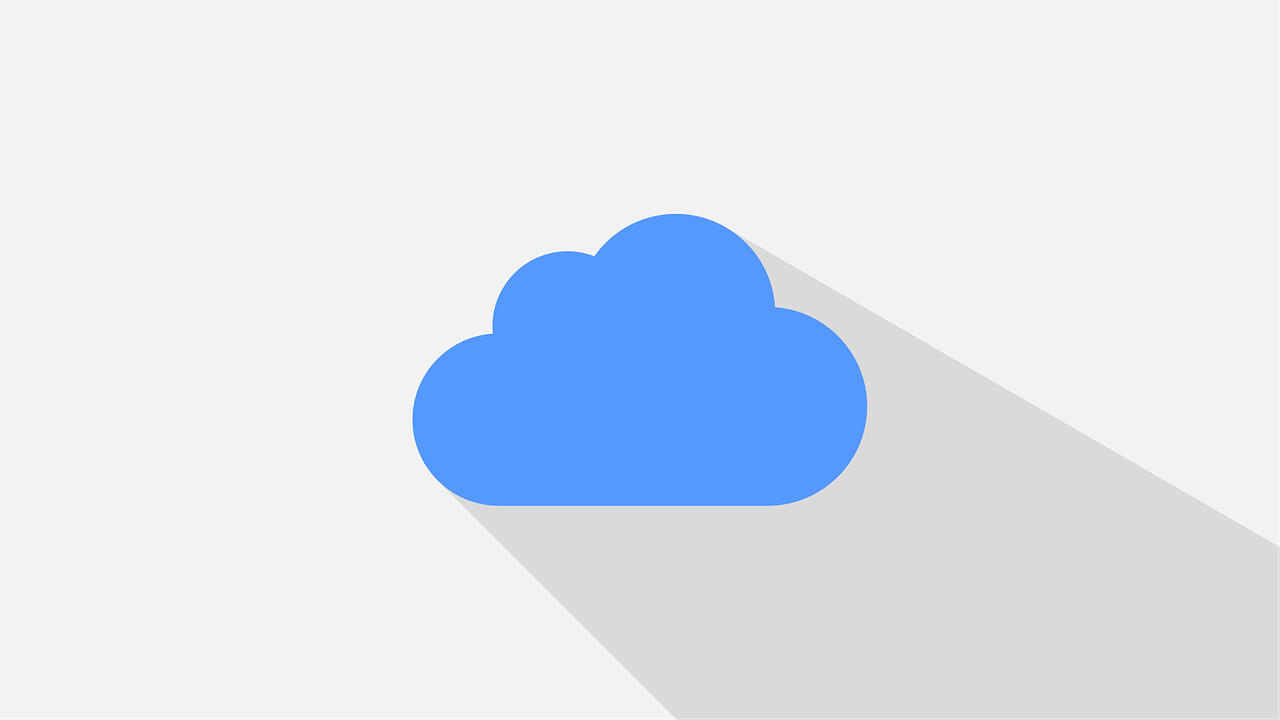
二、数据模型设计
首先定义两个实体类:Comment
和Reply
,每个评论可以有多个子回复,但子回复只能属于一个父级评论。
@Entity(tableName = "comments") public class Comment { @PrimaryKey(autoGenerate = true) private int id; private String userId; private String postId; // 关联到某个帖子 private String content; private long timestamp; // getters and setters... } @Entity(tableName = "replies") public class Reply { @PrimaryKey(autoGenerate = true) private int id; private int commentId; // 关联到某条评论 private String userId; private String content; private long timestamp; // getters and setters... }
三、数据库操作
使用Room持久化层来管理本地数据库的操作,为上述两个实体创建DAO接口,并提供相应的Repository类封装CRUD方法。
DAO接口示例
@Dao public interface CommentDao { @Insert void insertComment(Comment comment); @Query("SELECT * FROM comments WHERE postId = :postId ORDER BY timestamp DESC") List<Comment> getCommentsByPostId(String postId); } @Dao public interface ReplyDao { @Insert void insertReply(Reply reply); @Query("SELECT * FROM replies WHERE commentId = :commentId ORDER BY timestamp DESC") List<Reply> getRepliesByCommentId(int commentId); }
Repository类示例
public class CommentRepository { private CommentDao commentDao; private LiveData<List<Comment>> allComments; public CommentRepository(Application application) { CommentRoomDatabase db = CommentRoomDatabase.getDatabase(application); commentDao = db.commentDao(); allComments = commentDao.getAllComments(); } public LiveData<List<Comment>> getAllComments() { return allComments; } public void insert(Comment comment) { new InsertCommentAsyncTask(commentDao).execute(comment); } private static class InsertCommentAsyncTask extends AsyncTask<Comment, Void, Void> { private CommentDao commentDao; InsertCommentAsyncTask(CommentDao commentDao) { this.commentDao = commentDao; } @Override protected Void doInBackground(Comment... comments) { commentDao.insertComment(comments[0]); return null; } } }
四、UI设计与交互逻辑
1. 主界面布局 (activity_main.xml)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <RecyclerView android:id="@+id/recyclerView" android:layout_width="match_parent" android:layout_height="wrap_content"/> </LinearLayout>
2. RecyclerView适配器 (CommentAdapter.java)
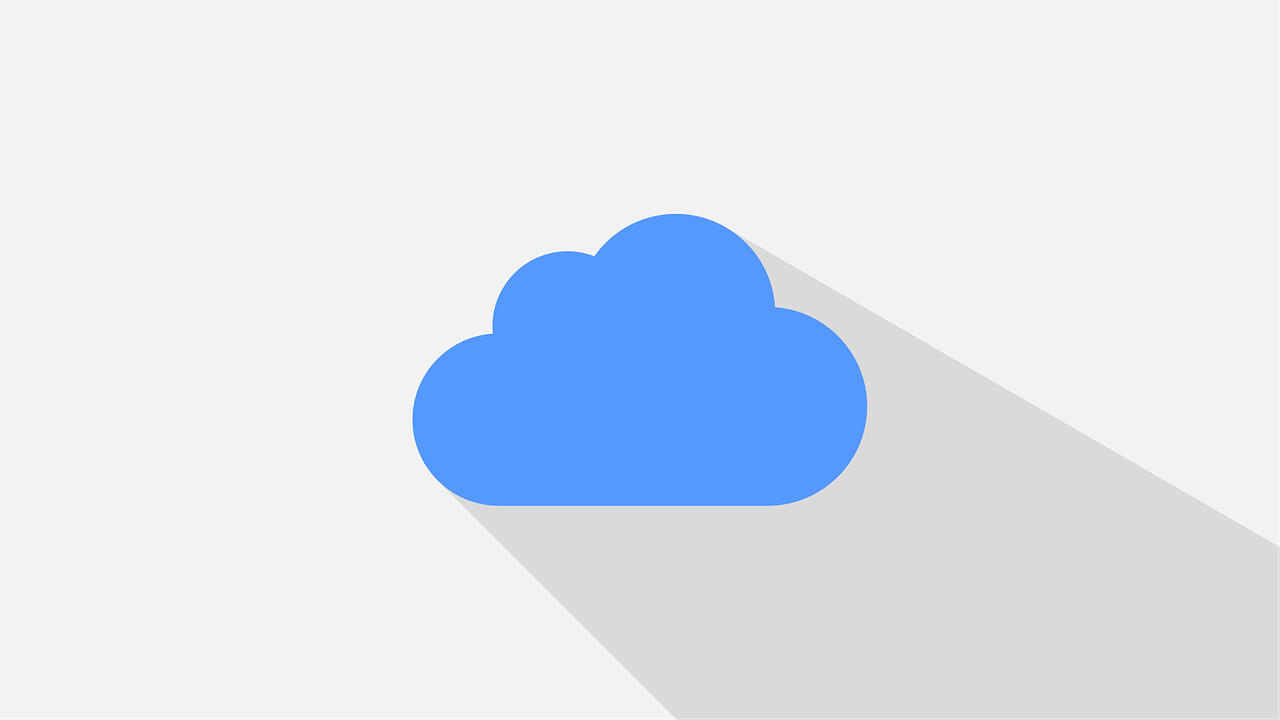
public class CommentAdapter extends RecyclerView.Adapter<CommentAdapter.CommentViewHolder> { private List<Comment> comments; private OnItemClickListener listener; public interface OnItemClickListener { void onReplyClick(int position); } public void setOnItemClickListener(OnItemClickListener listener) { this.listener = listener; } public static class CommentViewHolder extends RecyclerView.ViewHolder { public TextView commentTextView; public ImageButton replyButton; public CommentViewHolder(View itemView) { super(itemView); commentTextView = itemView.findViewById(R.id.textView); replyButton = itemView.findViewById(R.id.replyButton); } } @NonNull @Override public CommentViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) { View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_comment, parent, false); return new CommentViewHolder(view); } @Override public void onBindViewHolder(@NonNull final CommentViewHolder holder, int position) { final Comment comment = comments.get(position); holder.commentTextView.setText(comment.getContent()); holder.replyButton.setOnClickListener(v -> { if (listener != null) { listener.onReplyClick(holder.getAdapterPosition()); } }); } @Override public int getItemCount() { return comments.size(); } public void setComments(List<Comment> comments) { this.comments = comments; notifyDataSetChanged(); } }
3. 评论项布局 (item_comment.xml)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:padding="8dp"> <TextView android:id="@+id/textView" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" /> <ImageButton android:id="@+id/replyButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/ic_reply" /> </LinearLayout>
五、业务逻辑实现
添加评论功能
当用户点击“发表”按钮时,获取输入框内容并调用Repository保存新评论。
public void addComment(String content) { Comment comment = new Comment(); comment.setUserId("currentUserId"); // 替换为实际用户ID comment.setPostId("postId"); // 替换为实际帖子ID comment.setContent(content); comment.setTimestamp(System.currentTimeMillis()); commentRepository.insert(comment); }
回复评论功能
当用户点击某条评论旁边的“回复”按钮时,弹出对话框让用户输入回复内容,然后调用Repository保存新回复。
public void replyToComment(int commentId, String replyContent) { Reply reply = new Reply(); reply.setCommentId(commentId); reply.setUserId("currentUserId"); // 替换为实际用户ID reply.setContent(replyContent); reply.setTimestamp(System.currentTimeMillis()); replyRepository.insert(reply); }
显示评论及回复列表
通过RecyclerView展示所有评论及其对应的回复,可以使用嵌套的RecyclerView或者ExpandableListView来实现这一点,这里以嵌套RecyclerView为例:
public void displayComments(String postId) { commentRepository.getCommentsByPostId(postId).observe(this, new Observer<List<Comment>>() { @Override public void onChanged(@Nullable List<Comment> comments) { for (Comment comment : comments) { displayRepliesForComment(comment); } } }); } private void displayRepliesForComment(Comment comment) { // 假设已经有一个方法可以获取某条评论的所有回复 List<Reply> replies = replyRepository.getRepliesByCommentId(comment.getId()); // 更新UI,例如添加到RecyclerView中... }
六、网络同步与离线支持
为了确保数据一致性,并且能够在没有网络连接的情况下也能正常使用应用,我们需要将本地数据库与云端数据库进行同步,这里以Firebase Firestore为例:
public class SyncManager { private FirebaseFirestore db; private CommentRepository commentRepository; private ReplyRepository replyRepository; public SyncManager(Application application) { db = FirebaseFirestore.getInstance(); commentRepository = new CommentRepository(application); replyRepository = new ReplyRepository(application); } public void syncComments() { commentRepository.getAllComments().observe(new LifecycleOwner() { ... }, new Observer<List<Comment>>() { @Override public void onChanged(@Nullable List<Comment> comments) { if (comments != null) { for (Comment comment : comments) { Map<String, Object> data = new HashMap<>(); data.put("userId", comment.getUserId()); data.put("postId", comment.getPostId()); data.put("content", comment.getContent()); data.put("timestamp", comment.getTimestamp()); db.collection("comments").add(data); } } } }); } }
还需要处理从云端下载数据并更新本地数据库的逻辑,这部分内容较为复杂,涉及到冲突解决、增量更新等多个方面,具体实现取决于项目需求和技术选型。
以上就是关于“Android实现社交应用中评论与回复功能”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1284132.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复