Android实现调用摄像头和相册的方法
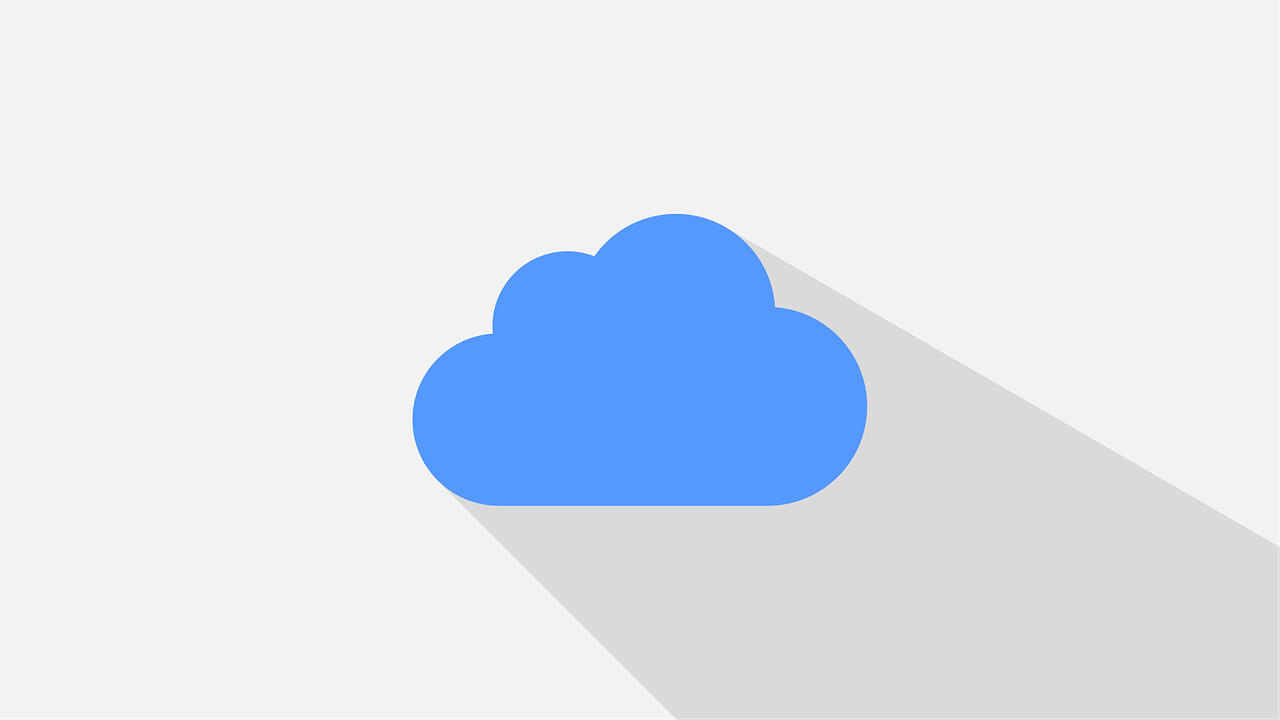
在现代移动应用开发中,集成摄像头和相册功能已成为许多应用程序的标配,Android提供了丰富的API来支持这些功能,使得开发者可以方便地实现拍照和选择图片的需求,本文将详细介绍如何在Android项目中调用摄像头和相册,并展示相关代码示例及注意事项。
一、前期准备
1. 添加权限
为了访问摄像头和存储设备,需要在AndroidManifest.xml
文件中声明以下权限:
<uses-permission android:name="android.permission.CAMERA"/> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
2. 添加依赖
在项目的build.gradle
文件中添加Glide库的依赖,用于加载和显示图像:
implementation 'com.github.bumptech.glide:glide:4.9.0' annotationProcessor 'com.github.bumptech.glide:compiler:4.9.0'
二、布局文件(activity_main.xml)
创建一个基本的布局文件,包含启动摄像头和打开相册的按钮以及一个用于显示图片的ImageView控件。
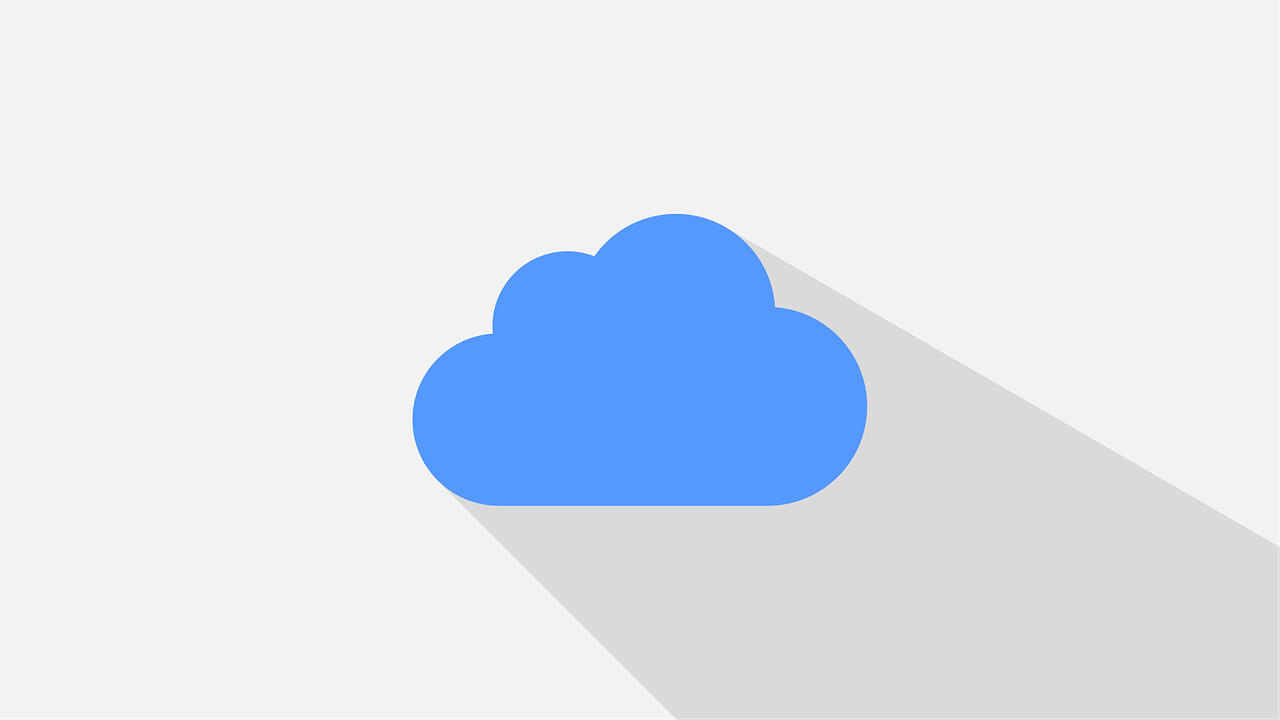
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <Button android:id="@+id/take_photo" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="启动相机"/> <Button android:id="@+id/choose_from_album" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="从相册中选择图片"/> <ImageView android:id="@+id/picture" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal"/> </LinearLayout>
三、MainActivity.java
在MainActivity
中实现启动摄像头和打开相册的功能,并处理回调以显示拍摄或选择的图片。
package com.example.luoxn28.activity; import android.Manifest; import android.app.Activity; import android.content.ContentUris; import android.content.Intent; import android.content.pm.PackageManager; import android.database.Cursor; import android.graphics.Bitmap; import android.net.Uri; import android.os.Build; import android.os.Bundle; import android.provider.DocumentsContract; import android.provider.MediaStore; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; import android.widget.ImageView; import android.widget.Toast; import java.io.File; import java.io.FileNotFoundException; import java.io.IOException; import java.util.Random; import androidx.core.content.ContextCompat; import androidx.core.content.FileProvider; import androidx.core.content.PermissionChecker; public class MainActivity extends AppCompatActivity { private static final String TAG = "hdu"; private static final int TAKE_PHOTO = 1; private static final int CROP_PHOTO = 2; private static final int CHOOSE_PHOTO = 3; private Button takePhoto, chooseFromAlbum; private ImageView picture; private Uri imageUri; private String currentPhotoPath; private String currentPhotoName; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); takePhoto = findViewById(R.id.take_photo); picture = findViewById(R.id.picture); chooseFromAlbum = findViewById(R.id.choose_from_album); takePhoto.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { btnCamOnclick(); } }); chooseFromAlbum.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { btnPhoOnclick(); } }); } private void btnCamOnclick() { Log.d(TAG, "onClick: 单击了按钮"); // 创建保存照片的文件 currentPhotoName = "img_" + new Random().nextInt(10000) + ".jpg"; currentPhotoPath = getExternalFilesDir(Environment.DIRECTORY_PICTURES).getAbsolutePath() + "/" + currentPhotoName; File imgfile = new File(currentPhotoPath); try { if (imgfile.exists()) { imgfile.delete(); } imgfile.createNewFile(); } catch (IOException e) { e.printStackTrace(); } // 创建Intent,启动相机程序 Intent intent = new Intent("android.media.action.IMAGE_CAPTURE"); intent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(imgfile)); startActivityForResult(intent, TAKE_PHOTO); // 请求码为TAKE_PHOTO } private void btnPhoOnclick() { Intent intent = new Intent(Intent.ACTION_PICK, MediaStore.Images.Media.EXTERNAL_CONTENT_URI); intent.setType("image/*"); startActivityForResult(intent, CHOOSE_PHOTO); // 请求码为CHOOSE_PHOTO } @Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { super.onActivityResult(requestCode, resultCode, data); switch (requestCode) { case TAKE_PHOTO: if (resultCode == RESULT_OK) { // 根据真实路径显示照片 showImage(currentPhotoPath); } else { Toast.makeText(this, "拍照失败", Toast.LENGTH_SHORT).show(); } break; case CHOOSE_PHOTO: if (resultCode == RESULT_OK) { // 获取选择的照片路径,并显示照片 Uri imageuri = data.getData(); // 这是缩略图路径 String[] filePathColumn = { MediaStore.Images.Media.DATA }; Cursor cursor = getContentResolver().query(imageuri, filePathColumn, null, null, null); if (cursor != null) { cursor.moveToFirst(); int columnIndex = cursor.getColumnIndex(filePathColumn[0]); String picturePath = cursor.getString(columnIndex); cursor.close(); showImage(picturePath); // 显示原图路径 } else { Toast.makeText(this, "获取图片路径失败", Toast.LENGTH_SHORT).show(); } } else { Toast.makeText(this, "取消选择", Toast.LENGTH_SHORT).show(); } break; default: break; } } private void showImage(String path) { File imgFile = new File(path); if (imgFile.exists()) { Bitmap myBitmap = BitmapFactory.decodeFile(imgFile.getAbsolutePath()); picture.setImageBitmap(myBitmap); } } }
1、权限处理:确保在运行时申请必要的权限,特别是在Android 6.0及以上版本,可以使用ActivityCompat.requestPermissions
方法动态申请权限。
2、文件管理:注意管理拍摄和选择的图片文件,避免内存泄漏和冗余文件,使用合适的命名规则以防止文件名冲突。
3、用户体验:在启动相机或相册时,可以通过进度条或对话框提示用户操作正在进行中,提升用户体验。
小伙伴们,上文介绍了“Android实现调用摄像头和相册的方法”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1284067.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复