Notification
类和 NotificationManager
来实现状态栏添加图标。以下是一个简单的函数实例:,,“java,public void addStatusBarIcon(Context context) {, Notification notification = new Notification.Builder(context), .setSmallIcon(R.drawable.icon), .setContentTitle("状态栏通知"), .setContentText("这是一个示例通知"), .build();, , NotificationManager notificationManager = (NotificationManager) context.getSystemService(Context.NOTIFICATION_SERVICE);, notificationManager.notify(1, notification);,},
“,,这个函数会在设备的状态栏上显示一个带有指定图标的通知。Android 实现状态栏添加图标的函数实例
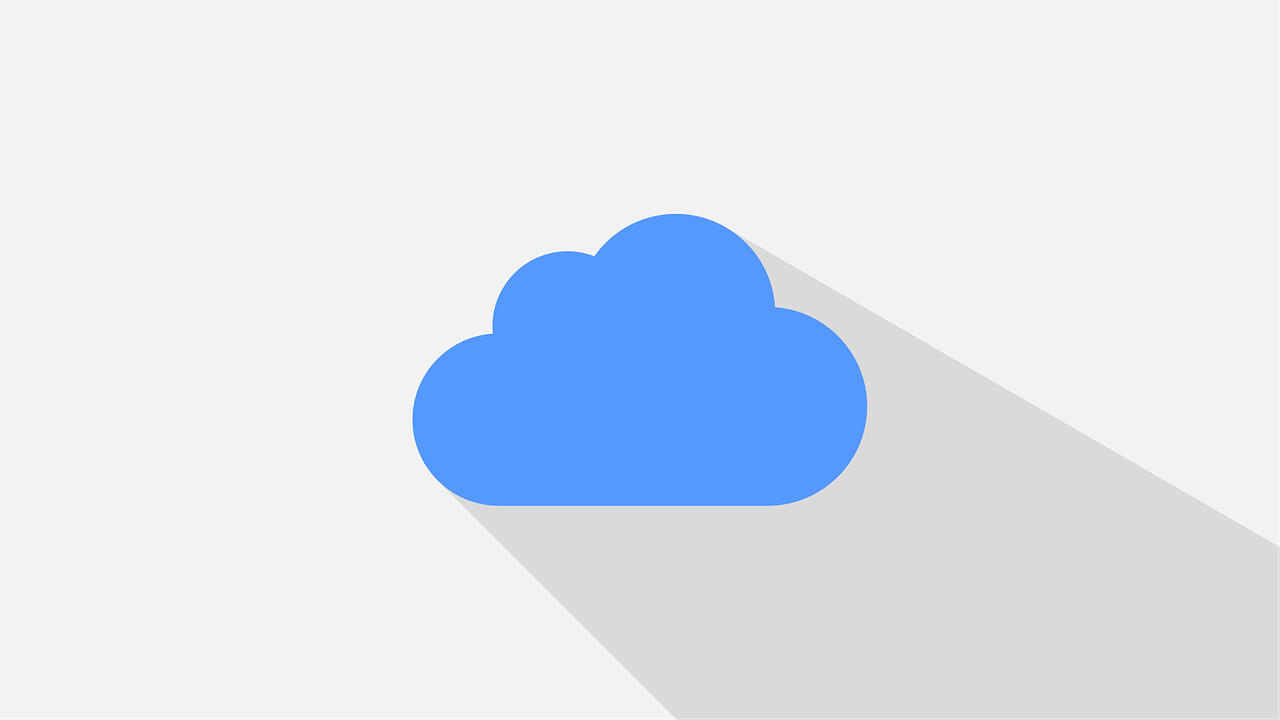
在Android开发中,向状态栏添加图标是一项常见的需求,特别是在通知和系统托盘中显示应用的状态或信息,本文将详细介绍如何在Android应用中实现这一功能,包括代码示例、步骤说明以及相关注意事项。
准备工作
1.1 创建项目
确保你已经安装了Android Studio,并创建一个新的Android项目。
1.2 配置权限
在你的AndroidManifest.xml
文件中,添加必要的权限:
<uses-permission android:name="android.permission.POST_NOTIFICATIONS" />
注意:POST_NOTIFICATIONS
权限仅适用于Android 13及更高版本,对于较低版本的Android,你不需要此权限。
1.3 定义图标资源
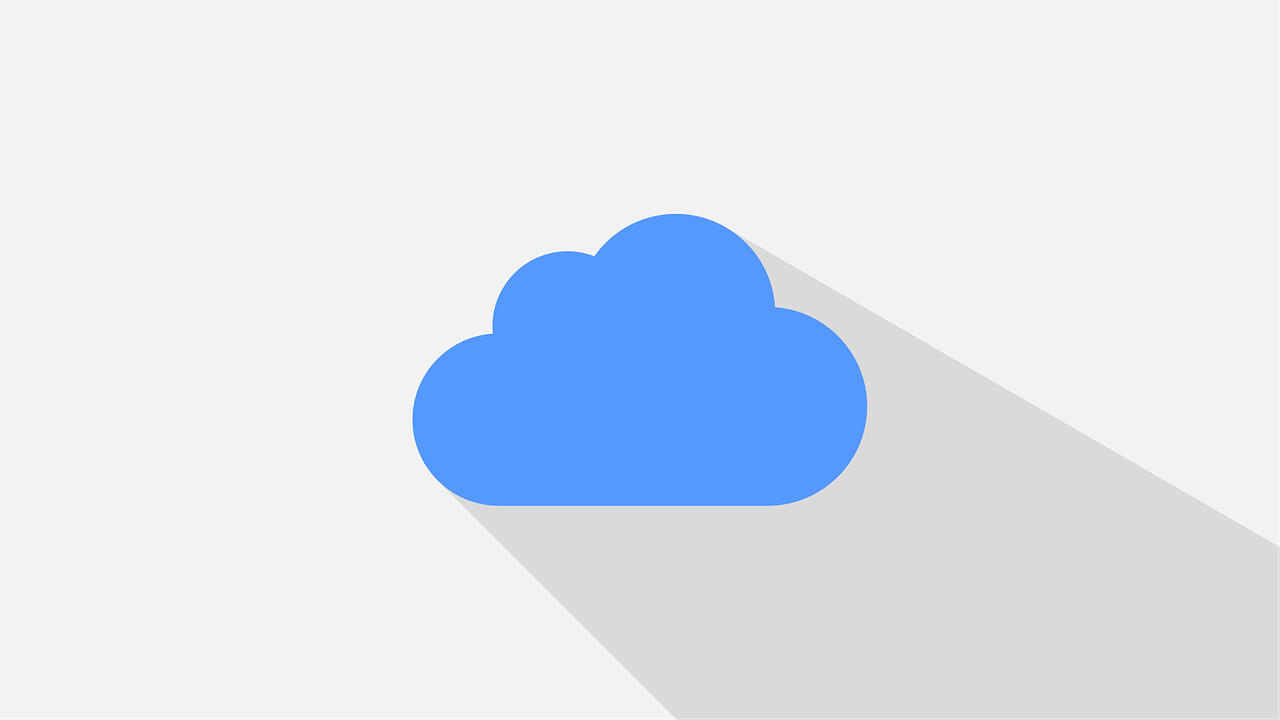
在res/drawable
目录下添加你的图标文件(例如ic_notification.png
),并在res/values/strings.xml
中定义通知标题和内容:
<resources> <string name="app_name">MyApp</string> <string name="notification_title">My Notification</string> <string name="notification_content">This is a sample notification content.</string> </resources>
2. 创建通知通道(适用于Android 8.0及以上)
从Android 8.0(API级别26)开始,所有通知都必须分配到一个频道,创建一个通知频道的代码如下:
import android.app.NotificationChannel; import android.app.NotificationManager; import android.content.Context; import android.os.Build; public class NotificationHelper { private static final String CHANNEL_ID = "my_channel_id"; private static final String CHANNEL_NAME = "My Channel"; private static final String CHANNEL_DESC = "This is my channel description"; public static void createNotificationChannel(Context context) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { NotificationChannel channel = new NotificationChannel(CHANNEL_ID, CHANNEL_NAME, NotificationManager.IMPORTANCE_DEFAULT); channel.setDescription(CHANNEL_DESC); NotificationManager manager = context.getSystemService(NotificationManager.class); if (manager != null) { manager.createNotificationChannel(channel); } } } }
在应用程序启动时调用此方法:
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); NotificationHelper.createNotificationChannel(this); }
创建通知并显示
使用NotificationCompat.Builder
类来构建通知,并使用NotificationManager
来显示通知,以下是一个完整的示例:
import android.app.Notification; import android.app.NotificationChannel; import android.app.NotificationManager; import android.content.Context; import android.graphics.BitmapFactory; import android.graphics.Color; import android.os.Build; import androidx.annotation.RequiresApi; import androidx.core.app.NotificationCompat; import androidx.core.app.NotificationManagerCompat; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // 创建通知通道 createNotificationChannel(); // 创建并显示通知 showNotification(); } private void createNotificationChannel() { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { String channelId = "my_channel_id"; CharSequence channelName = "My Channel"; String channelDescription = "This is my channel description"; int importance = NotificationManager.IMPORTANCE_DEFAULT; NotificationChannel channel = new NotificationChannel(channelId, channelName, importance); channel.setDescription(channelDescription); NotificationManager notificationManager = getSystemService(NotificationManager.class); notificationManager.createNotificationChannel(channel); } } private void showNotification() { // 创建通知内容 NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "my_channel_id") .setSmallIcon(R.drawable.ic_notification) // 设置小图标 .setContentTitle("My Notification") // 设置通知标题 .setContentText("This is a sample notification content.") // 设置通知内容 .setPriority(NotificationCompat.PRIORITY_DEFAULT) // 设置优先级 .setAutoCancel(true) // 点击通知后自动取消 .setShowWhen(true) // 显示时间戳 .setColor(Color.BLUE) // 设置通知颜色 .setLargeIcon(BitmapFactory.decodeResource(getResources(), R.drawable.ic_notification)); // 设置大图标 // 显示通知 NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(1, builder.build()); // 通知ID为1 } }
自定义通知布局(可选)
如果默认的通知布局不能满足你的需求,你可以自定义通知布局,在res/layout
目录下创建一个XML布局文件(例如notification_custom.xml
):
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp"> <ImageView android:id="@+id/image" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/ic_notification" /> <TextView android:id="@+id/title" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_toEndOf="@id/image" android:layout_marginStart="10dp" android:text="Custom Title" android:textSize="16sp" /> <TextView android:id="@+id/text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/title" android:layout_toEndOf="@id/image" android:layout_marginTop="5dp" android:text="Custom Content" android:textSize="14sp" /> </RelativeLayout>
在代码中使用自定义布局:
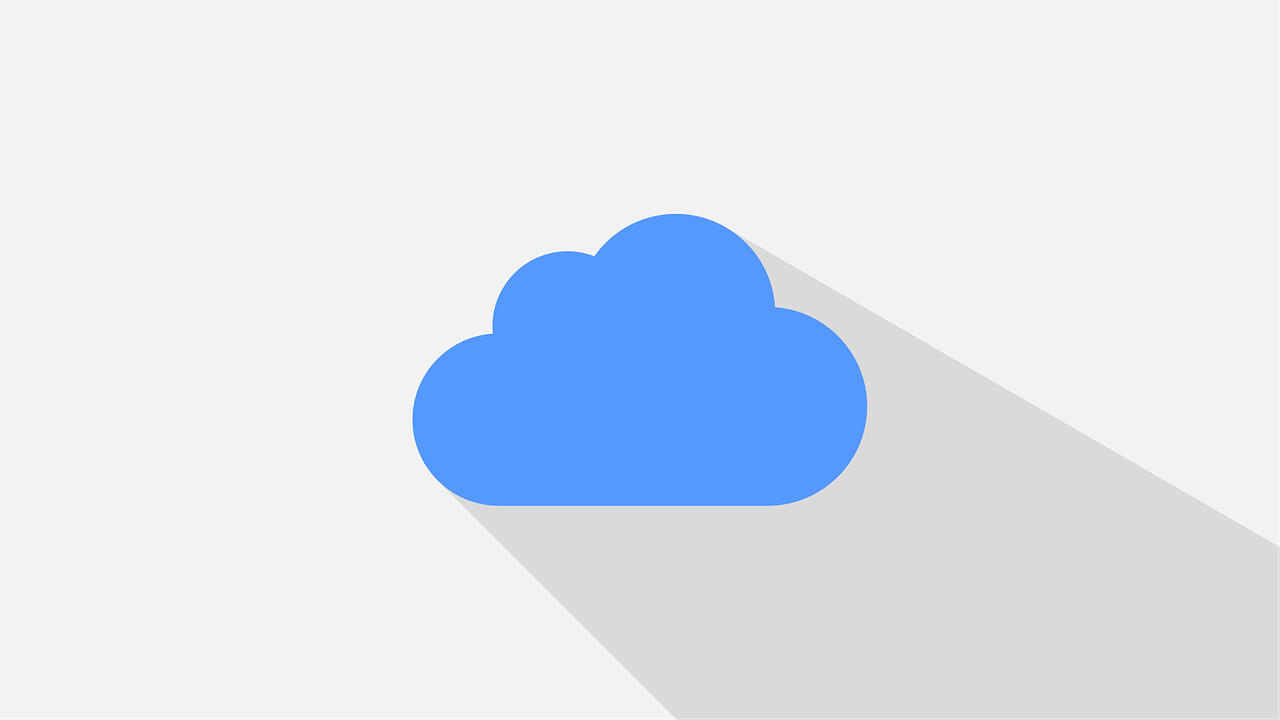
private void showCustomNotification() { // 获取自定义布局的RemoteViews对象 RemoteViews remoteViews = new RemoteViews(this.getPackageName(), R.layout.notification_custom); remoteViews.setImageViewResource(R.id.image, R.drawable.ic_notification); remoteViews.setTextViewText(R.id.title, "Custom Title"); remoteViews.setTextViewText(R.id.text, "Custom Content"); NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "my_channel_id") .setSmallIcon(R.drawable.ic_notification) // 小图标仍然需要设置 .setStyle(new NotificationCompat.DecoratedCustomViewStyle()) // 设置自定义样式 .setCustomContentView(remoteViews) // 设置自定义视图 .setPriority(NotificationCompat.PRIORITY_DEFAULT) // 设置优先级 .setAutoCancel(true) // 点击通知后自动取消 .setShowWhen(true); // 显示时间戳 // 显示通知 NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(2, builder.build()); // 通知ID为2 }
更新通知(可选)
有时你可能需要更新已经显示的通知,可以使用相同的通知ID来更新通知:
private void updateNotification() { NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "my_channel_id") .setSmallIcon(R.drawable.ic_notification) .setContentTitle("Updated Title") .setContentText("This is the updated content of the notification.") .setPriority(NotificationCompat.PRIORITY_DEFAULT) .setAutoCancel(true) .setShowWhen(true) .setLargeIcon(BitmapFactory.decodeResource(getResources(), R.drawable.ic_notification)); // 更新通知 NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(1, builder.build()); // 使用相同的通知ID(1)进行更新 }
删除通知(可选)
当你不再需要显示某个通知时,可以将其删除:
private void cancelNotification() { NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.cancel(1); // 使用通知ID(1)进行删除 }
处理通知点击事件(可选)
可以为通知设置点击事件,当用户点击通知时执行特定的操作:
private void showNotificationWithPendingIntent() { Intent intent = new Intent(this, TargetActivity.class); // 目标活动 PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT | PendingIntent.FLAG_IMMUTABLE); NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "my_channel_id") .setSmallIcon(R.drawable.ic_notification) .setContentTitle("Notification with PendingIntent") .setContentText("Tap to open TargetActivity") .setPriority(NotificationCompat.PRIORITY_DEFAULT) .setAutoCancel(true) .setShowWhen(true) .setContentIntent(pendingIntent) // 设置PendingIntent .setLargeIcon(BitmapFactory.decodeResource(getResources(), R.drawable.ic_notification)); // 显示通知 NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(3, builder.build()); // 通知ID为3 }
保持简洁:尽量保持通知内容的简洁明了,避免过多的文字。
使用适当的图标:选择合适的图标可以提高用户的识别度。
考虑用户体验:确保通知不会频繁打扰用户,合理设置通知的频率和条件。
测试不同设备:在不同设备和Android版本上测试通知的显示效果,以确保兼容性。
遵循Material Design指南:遵循Google的Material Design指南,提高用户界面的一致性和美观性。
优化性能:在后台任务中创建和管理通知,避免在主线程中进行耗时操作。
处理异常情况:在创建和管理通知时,处理可能出现的异常情况,如权限不足或API不兼容等。
以上内容就是解答有关“android实现状态栏添加图标的函数实例”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1283473.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复