setOnShowListener
方法,并在其中设置一个OnDismissListener
。在OnDismissListener
中,可以调用dialog.dismiss()
来取消默认的关闭行为。Android实现点击AlertDialog上按钮时不关闭对话框的方法
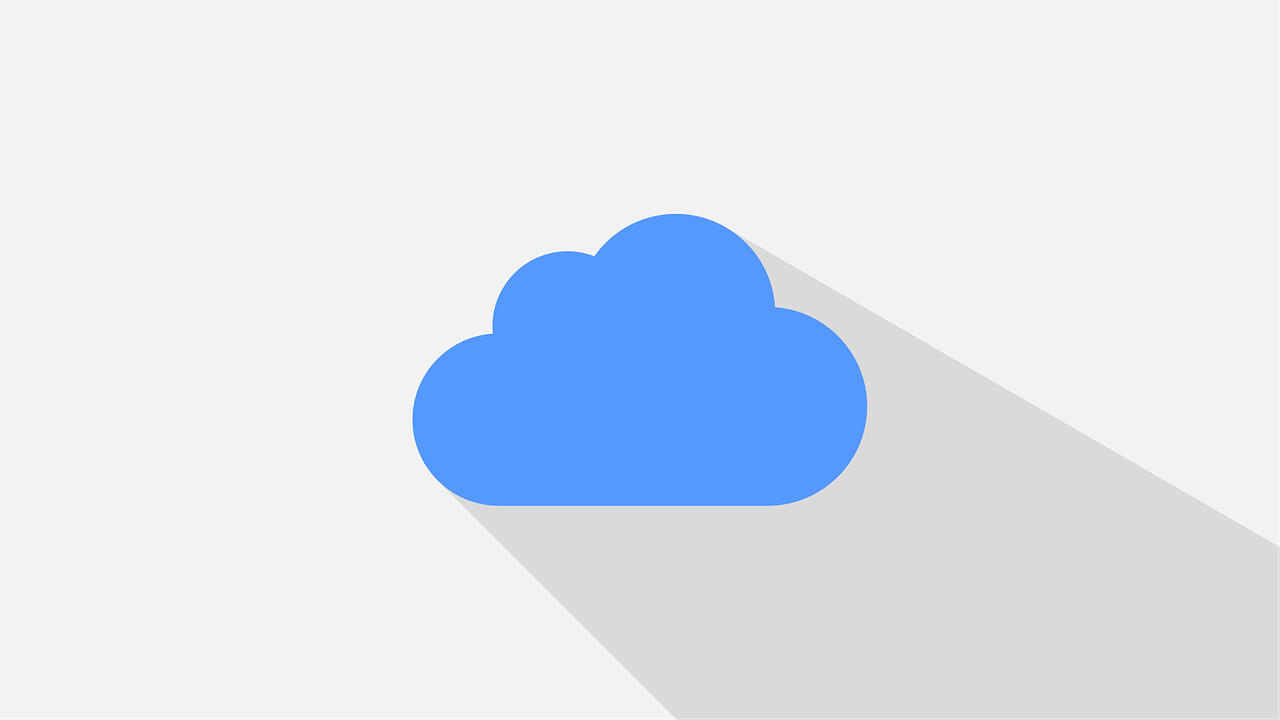
在Android开发中,AlertDialog
是用于显示消息、警告或选项的常见组件,当用户点击对话框上的按钮时,对话框会自动关闭,在某些情况下,开发者可能希望在用户点击按钮后保持对话框打开,以便执行更多操作或收集更多信息,本文将介绍如何在Android中实现这一功能。
创建AlertDialog
我们需要创建一个AlertDialog
,这可以通过使用AlertDialog.Builder
来完成,以下是创建一个简单的AlertDialog
的示例代码:
AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Title"); builder.setMessage("Message"); // Add a button builder.setPositiveButton("OK", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { // Handle the button click here } }); AlertDialog dialog = builder.create(); dialog.show();
自定义按钮点击事件
为了在点击按钮时不关闭对话框,我们需要自定义按钮的点击事件,我们可以通过设置一个OnShowListener
来实现这一点,在这个监听器中,我们可以拦截按钮的点击事件,并根据需要决定是否关闭对话框。
dialog.setOnShowListener(new DialogInterface.OnShowListener() { @Override public void onShow(DialogInterface dialog) { Button positiveButton = ((AlertDialog) dialog).getButton(AlertDialog.BUTTON_POSITIVE); positiveButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Custom logic for button click // Do not call dialog.dismiss() or dialog.cancel() here } }); } });
更新按钮状态
在某些情况下,我们可能需要在按钮点击后更新按钮的状态(禁用按钮以防止重复点击),这可以在自定义的按钮点击事件中实现。
positiveButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Custom logic for button click // Update button state if necessary positiveButton.setEnabled(false); } });
处理其他逻辑
在自定义的按钮点击事件中,我们可以添加任何其他所需的逻辑,我们可以显示另一个对话框,或者更新界面上的其他元素。
positiveButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Custom logic for button click // Show another dialog or update UI elements new AlertDialog.Builder(MainActivity.this) .setTitle("Another Dialog") .setMessage("This is an additional dialog.") .setPositiveButton("OK", null) .show(); } });
完整示例代码
以下是一个完整的示例代码,展示了如何创建一个AlertDialog
并在点击按钮时保持对话框打开:
public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Title"); builder.setMessage("Message"); // Add a button builder.setPositiveButton("OK", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { // Handle the button click here } }); AlertDialog dialog = builder.create(); dialog.show(); dialog.setOnShowListener(new DialogInterface.OnShowListener() { @Override public void onShow(DialogInterface dialog) { Button positiveButton = ((AlertDialog) dialog).getButton(AlertDialog.BUTTON_POSITIVE); positiveButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Custom logic for button click // Show another dialog or update UI elements new AlertDialog.Builder(MainActivity.this) .setTitle("Another Dialog") .setMessage("This is an additional dialog.") .setPositiveButton("OK", null) .show(); } }); } }); } }
通过以上步骤,我们可以在Android中实现点击AlertDialog
上的按钮时不关闭对话框的功能,这可以通过自定义按钮的点击事件和使用OnShowListener
来实现,这种方法允许我们在按钮点击后执行更多的逻辑,而不会自动关闭对话框。
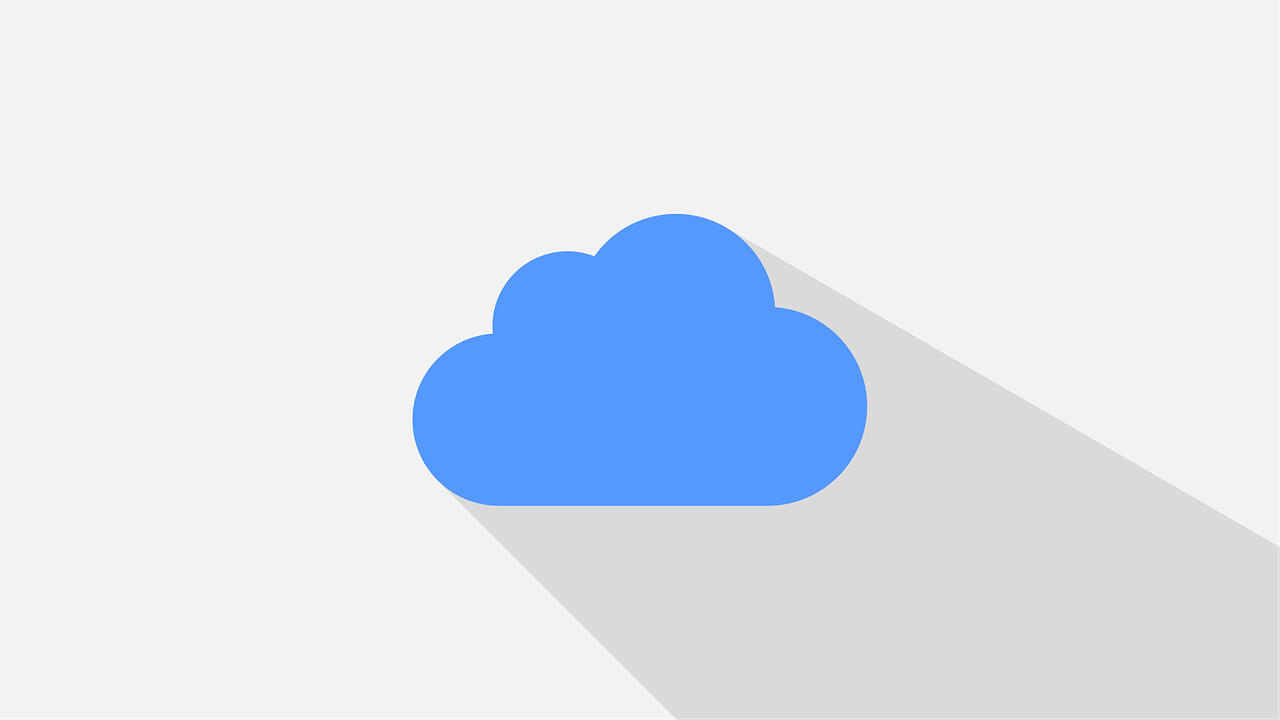
各位小伙伴们,我刚刚为大家分享了有关“Android实现点击AlertDialog上按钮时不关闭对话框的方法”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1282860.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复