ObjectAnimator
和RecyclerView
实现商品添加到购物车的动画效果。通过监听添加按钮的点击事件,使用动画将商品从列表滑动到购物车图标的位置,并更新购物车的数量显示。在Android应用开发中,实现添加商品到购物车的动画效果可以极大地提升用户体验,本文将详细介绍如何在Android中实现这一功能,包括使用RecyclerView展示购物车商品列表、自定义动画效果以及相关代码示例。
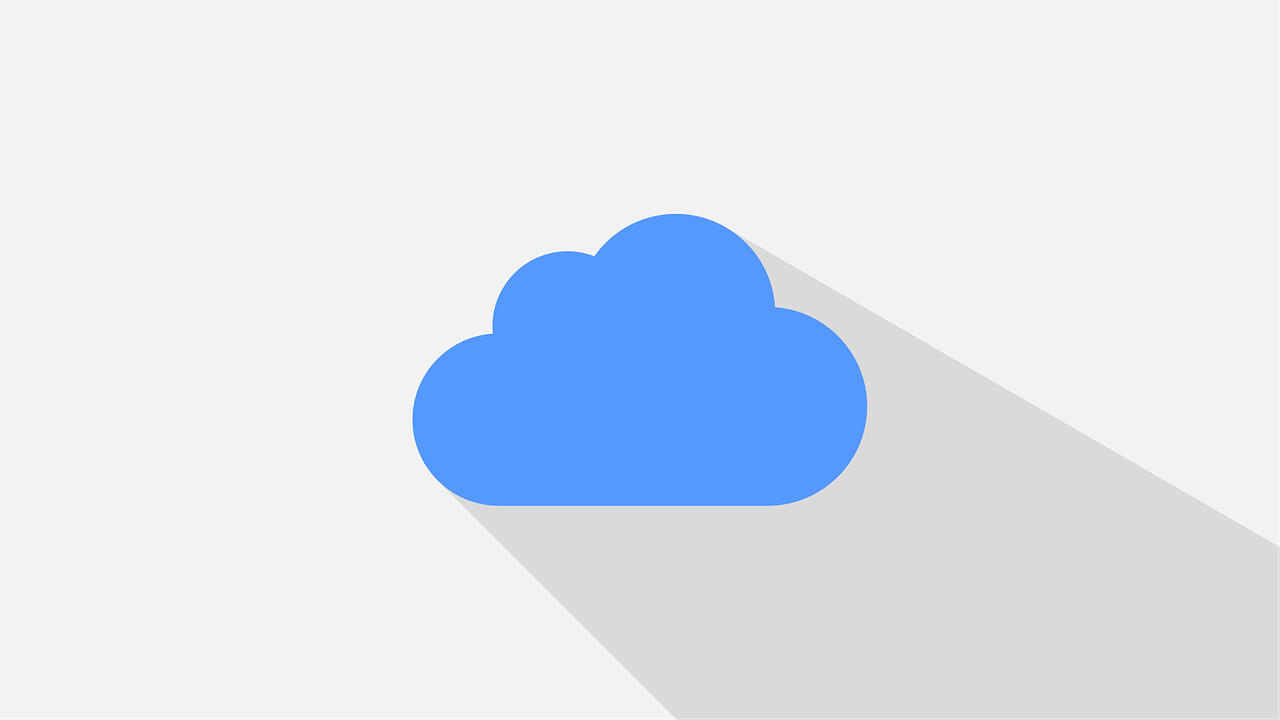
一、
1 项目背景
随着移动互联网的发展,电商应用已经成为人们日常生活中不可或缺的一部分,为了提高用户的购物体验,添加商品到购物车时的动画效果显得尤为重要,通过动画效果,用户可以直观地看到商品被添加到购物车的过程,从而增强交互性和趣味性。
2 目标
本文的目标是在Android应用中实现一个添加商品到购物车的动画效果,具体包括以下功能:
用户点击“添加到购物车”按钮后,商品从当前位置飞入购物车图标。
购物车图标显示商品数量的变化。
购物车内的商品列表实时更新。
二、准备工作
1 创建项目
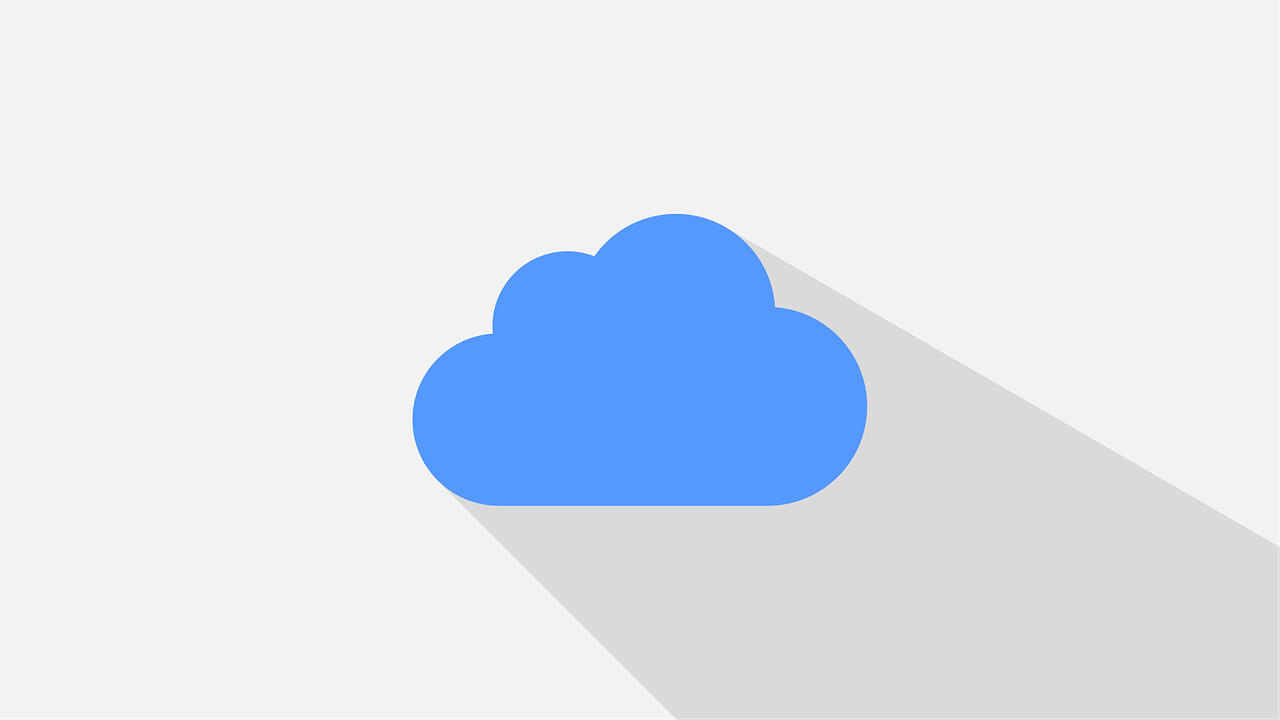
我们需要创建一个新的Android项目,在Android Studio中,选择“Start a new Android Studio project”,然后按照向导完成项目的创建。
2 添加依赖
在build.gradle
文件中添加必要的依赖,例如RecyclerView和CardView:
dependencies { implementation 'androidx.recyclerview:recyclerview:1.2.1' implementation 'androidx.cardview:cardview:1.0.0' }
三、布局文件
3.1 activity_main.xml
在res/layout/activity_main.xml
中定义主界面布局,包括一个RecyclerView用于展示商品列表和一个FloatingActionButton用于触发添加商品到购物车的动画效果。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <androidx.recyclerview.widget.RecyclerView android:id="@+id/recyclerView" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_above="@id/floatingActionButton" /> <com.google.android.material.floatingactionbutton.FloatingActionButton android:id="@+id/floatingActionButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_alignParentEnd="true" android:layout_margin="@dimen/fab_margin" android:src="@drawable/ic_add_shopping_cart_black_24dp" android:tint="@android:color/white" /> </RelativeLayout>
2 item_product.xml
在res/layout/item_product.xml
中定义商品项的布局,包括商品图片、名称和价格。
<?xml version="1.0" encoding="utf-8"?> <androidx.cardview.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="8dp"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="16dp"> <ImageView android:id="@+id/imageView" android:layout_width="match_parent" android:layout_height="200dp" android:scaleType="centerCrop" /> <TextView android:id="@+id/textViewName" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="18sp" android:textStyle="bold" /> <TextView android:id="@+id/textViewPrice" android:layout_width="match_parent" android:layout_height="wrap_content" /> </LinearLayout> </androidx.cardview.widget.CardView>
四、Adapter类
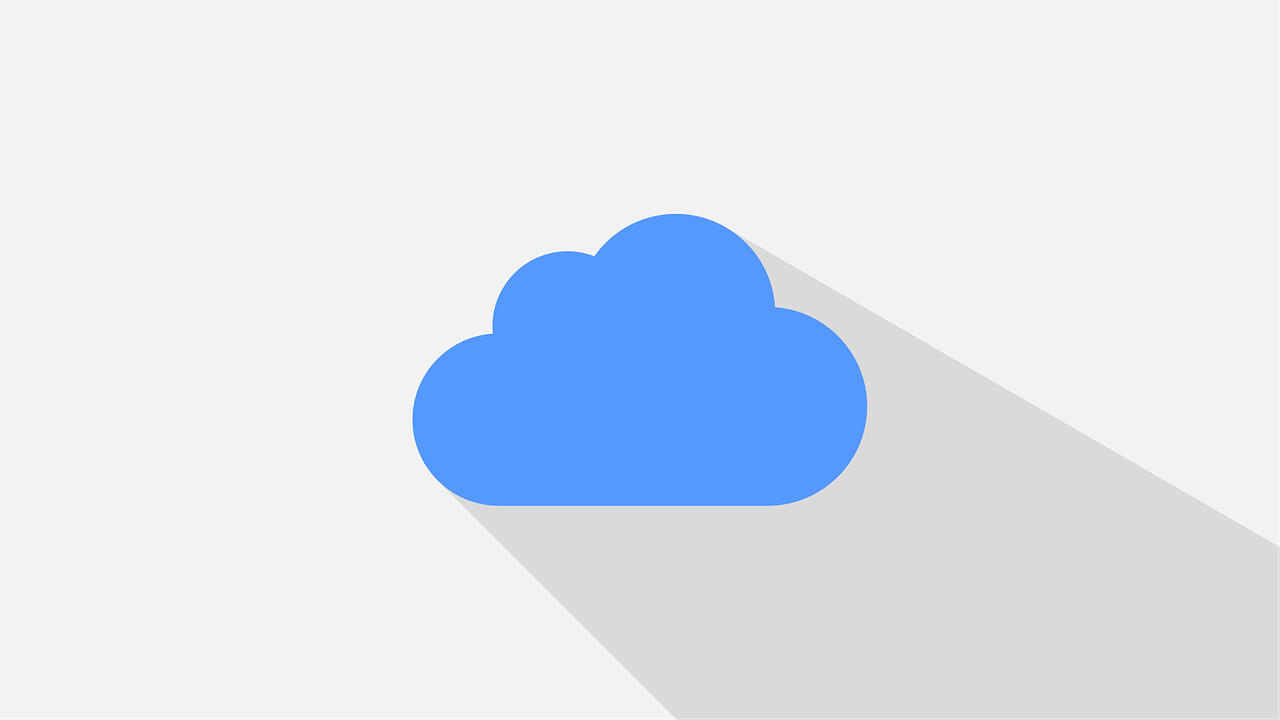
创建一个ProductAdapter
类来管理商品列表的数据和视图。
public class ProductAdapter extends RecyclerView.Adapter<ProductAdapter.ProductViewHolder> { private List<Product> productList; private OnItemClickListener onItemClickListener; public ProductAdapter(List<Product> productList) { this.productList = productList; } @NonNull @Override public ProductViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) { View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_product, parent, false); return new ProductViewHolder(view); } @Override public void onBindViewHolder(@NonNull ProductViewHolder holder, int position) { Product product = productList.get(position); holder.imageView.setImageResource(product.getImage()); holder.textViewName.setText(product.getName()); holder.textViewPrice.setText("$" + product.getPrice()); } @Override public int getItemCount() { return productList.size(); } public void setOnItemClickListener(OnItemClickListener onItemClickListener) { this.onItemClickListener = onItemClickListener; } public interface OnItemClickListener { void onItemClick(int position); } static class ProductViewHolder extends RecyclerView.ViewHolder { ImageView imageView; TextView textViewName; TextView textViewPrice; public ProductViewHolder(@NonNull View itemView) { super(itemView); imageView = itemView.findViewById(R.id.imageView); textViewName = itemView.findViewById(R.id.textViewName); textViewPrice = itemView.findViewById(R.id.textViewPrice); } } }
五、MainActivity类
在MainActivity
中设置RecyclerView的数据源,并为FloatingActionButton添加点击事件监听器以触发添加商品到购物车的动画效果。
public class MainActivity extends AppCompatActivity { private RecyclerView recyclerView; private ProductAdapter productAdapter; private FloatingActionButton floatingActionButton; private List<Product> productList; private int cartItemCount = 0; // 购物车商品数量 @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // 初始化数据源 productList = new ArrayList<>(); for (int i = 1; i <= 20; i++) { productList.add(new Product("商品" + i, "这是商品" + i, R.drawable.sample)); } // 设置RecyclerView recyclerView = findViewById(R.id.recyclerView); recyclerView.setLayoutManager(new LinearLayoutManager(this)); productAdapter = new ProductAdapter(productList); recyclerView.setAdapter(productAdapter); // 为FloatingActionButton设置点击事件监听器 floatingActionButton = findViewById(R.id.floatingActionButton); floatingActionButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { addProductToCart(); } }); } private void addProductToCart() { if (!productList.isEmpty()) { // 获取最后一个商品作为添加的商品 Product addedProduct = productList.get(productList.size() 1); // 创建并启动动画效果 startAnimation(addedProduct); // 从列表中移除该商品(模拟添加到购物车) productList.remove(addedProduct); // 通知适配器数据已更改 productAdapter.notifyDataSetChanged(); // 更新购物车商品数量 cartItemCount++; // 更新购物车图标上的商品数量(如果需要) updateCartIcon(); } else { Toast.makeText(this, "没有更多商品可添加", Toast.LENGTH_SHORT).show(); } } private void startAnimation(Product product) { // 创建动画效果的具体实现(略) // 这里可以使用ObjectAnimator或其他动画类来实现商品从当前位置飞入购物车图标的效果 } private void updateCartIcon() { // 更新购物车图标上的商品数量(如果需要) // 可以通过BadgeDrawable等方式实现 } }
六、运行效果
至此,我们已经完成了一个简单的Android应用,实现了添加商品到购物车的动画效果,当用户点击FloatingActionButton时,最后一个商品将从列表中移除,并通过动画效果飞入购物车图标的位置,购物车的商品数量也会相应增加,并且购物车内的商品列表会实时更新。
以上就是关于“Android实现添加商品到购物车动画效果”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1282294.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复