Linux驱动中的ioctl函数是一个非常重要的系统调用接口,它允许用户空间程序与设备驱动程序进行交互,本文将详细介绍ioctl的构成、实现以及常见问题解答。
ioctl简介
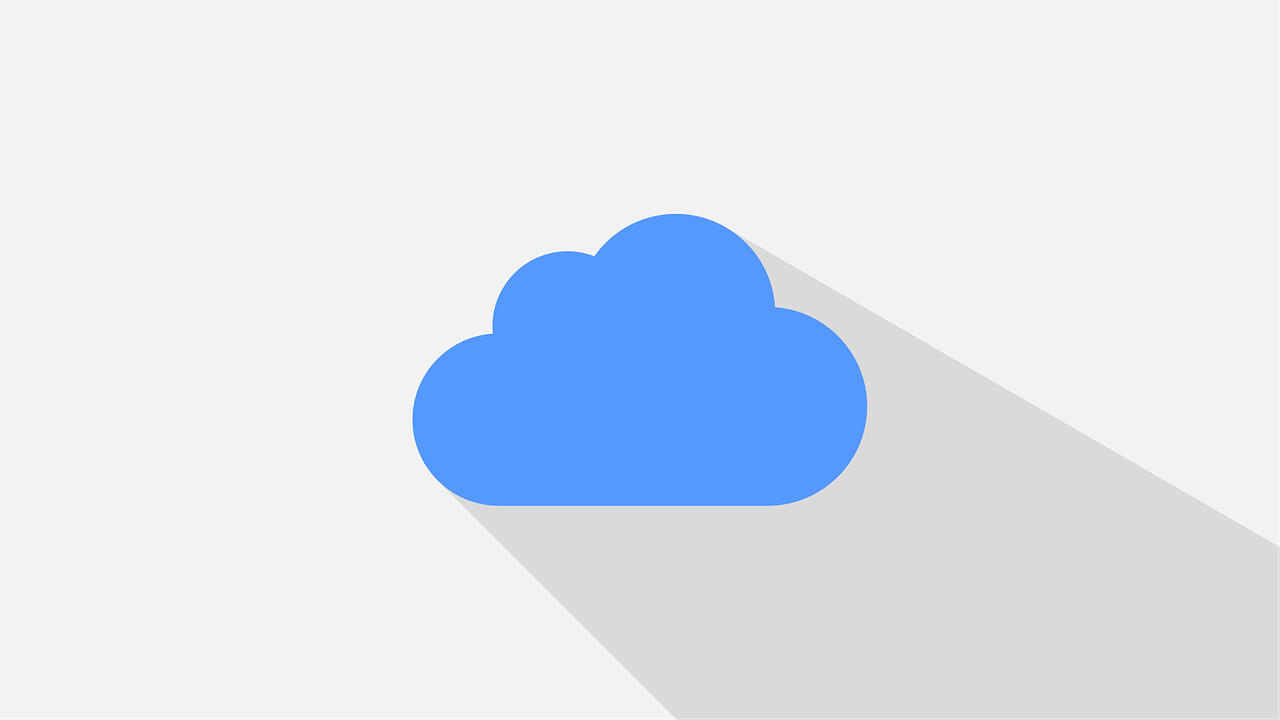
ioctl(输入/输出控制)是Linux专门为用户层控制设备设计的系统调用接口,通过这个接口,用户可以向设备发送各种命令,从而实现对设备的控制和数据交换,这种机制极大地提高了设备控制的灵活性。
ioctl命令的构成
一个ioctl命令由32位整数表示,其构成如下:
设备类型 | 序列号 | 方向 | 数据尺寸 |
8 bit | 8 bit | 2 bit | 13/14 bit |
具体的宏定义如下:
_IO(type, nr)
:没有参数的命令。
_IOR(type, nr, size)
:从设备读取数据的命令。
_IOW(type, nr, size)
:向设备写入数据的命令。
_IOWR(type, nr, size)
:双向数据传输的命令。
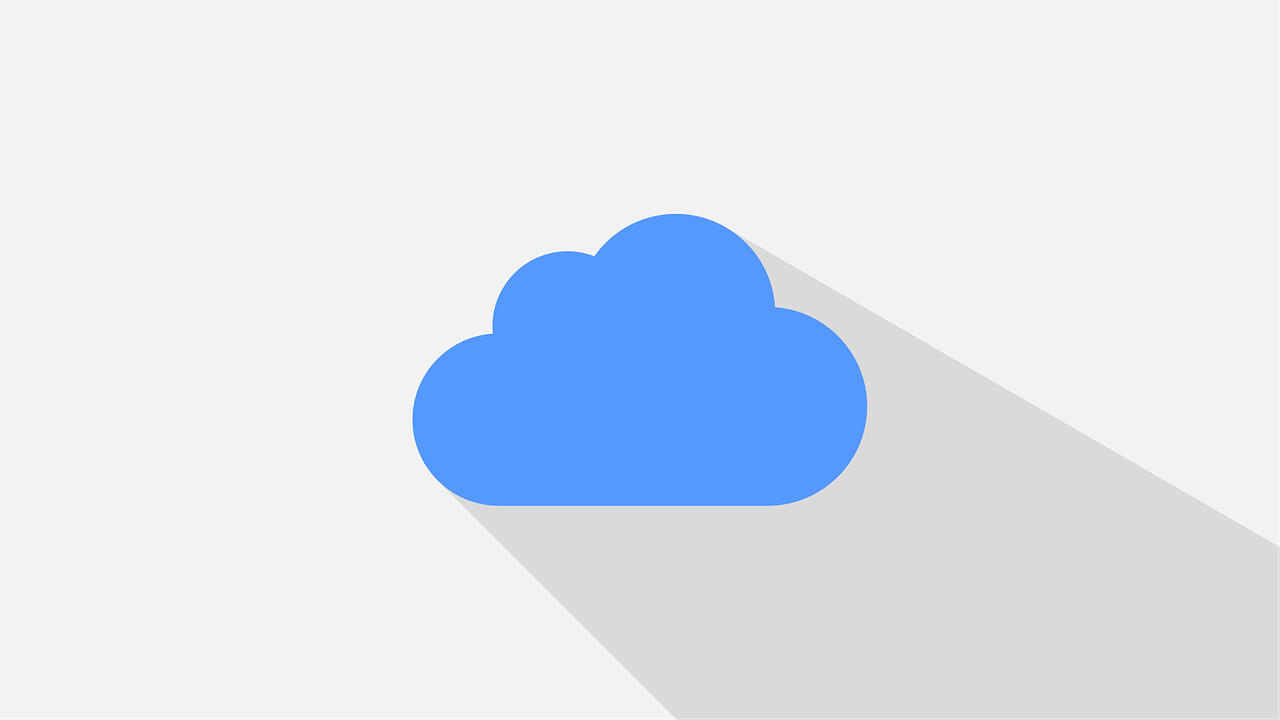
这些宏的具体定义在系统头文件<linux/ioctl.h>
中。
#define MY_IOCTL_SET_PARAM _IOW('M', 1, int)
ioctl命令的分解
内核提供了一些宏来分解ioctl命令号,以便提取其中的各部分信息:
_IOC_DIR(cmd)
:获得传输方向位段的值。
_IOC_TYPE(cmd)
:获得类型的值。
_IOC_NR(cmd)
:获得编号的值。
_IOC_SIZE(cmd)
:获得大小的值。
对于命令MY_IOCTL_SET_PARAM
,可以使用以下宏进行分解:
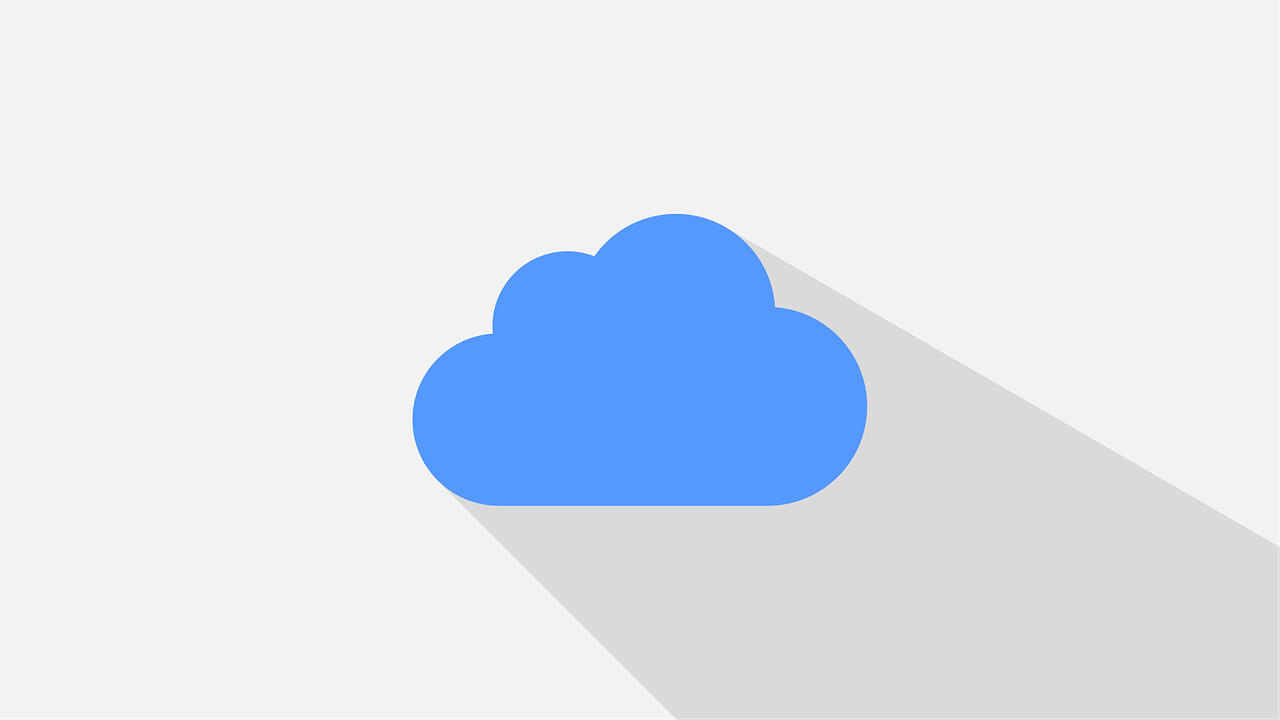
int direction = _IOC_DIR(MY_IOCTL_SET_PARAM); unsigned char type = _IOC_TYPE(MY_IOCTL_SET_PARAM); int nr = _IOC_NR(MY_IOCTL_SET_PARAM); size_t size = _IOC_SIZE(MY_IOCTL_SET_PARAM);
用户空间ioctl调用
用户空间应用程序通过文件描述符调用ioctl系统调用,传递命令和参数。
int fd = open("/dev/my_device", O_RDWR); int param = 42; ioctl(fd, MY_IOCTL_SET_PARAM, ¶m); close(fd);
驱动层ioctl实现
在驱动层,我们需要在文件操作结构体中实现ioctl处理函数。
static long my_device_ioctl(struct file *filp, unsigned int cmd, unsigned long arg) { switch (cmd) { case MY_IOCTL_SET_PARAM: { int param; if (copy_from_user(¶m, (int __user *)arg, sizeof(param))) return -EFAULT; // 设置设备参数 break; } default: return -EINVAL; } return 0; }
实验示例
以下是一个完整的驱动和应用程序示例,展示了如何使用ioctl进行设备控制。
驱动代码(hello.c)
#include <linux/module.h> #include <linux/kernel.h> #include <linux/fs.h> #include <linux/init.h> #include <linux/delay.h> #include <linux/irq.h> #include <linux/poll.h> #include <linux/cdev.h> #include <linux/device.h> #include "command.h" #define HELLO_CNT 1 //主设备号为0,表示动态分配设备号 dev_t dev = 0;static int major = 0; static int minor = 0;static struct cdev *hello_cdev[HELLO_CNT];static struct class *hello_class = NULL;static struct class_device * hello_class_dev[HELLO_CNT];static int s_val = 0;int hello_open(struct inode * pnode, struct file * pfile){ printk("open file.. "); int num = MINOR(pnode->i_rdev); if(num >= HELLO_CNT) { return -ENODEV; } pfile->private_data = hello_cdev[num]; return 0;}int hello_release(struct inode *pnode, struct file *pfile){ printk("release file .. "); return 0;}long hello_ioctl(struct file *pfile, unsigned int cmd, unsigned long arg){ int ret = 0; switch(cmd) { case HELLO_RESET: { printk("Rev HELLO_RESET cmd "); break; } case HELLO_READ: { printk("Rec HELLO_READ cmd "); ret = copy_to_user(arg, &s_val, sizeof(int)); break; } case HELLO_WRITE: { printk("Rec HELLO_WRITE cmd ");
应用程序代码(test.c)
#include <stdio.h> #include <fcntl.h> #include <unistd.h> #include <sys/ioctl.h> #include <linux/ioctl.h> #include "command.h" int main() { int fd = open("/dev/hello", O_RDWR); if (fd < 0) { perror("open"); return -1; } int val = 100; if (ioctl(fd, HELLO_WRITE, &val) == -1) { perror("ioctl write"); close(fd); return -1; } if (ioctl(fd, HELLO_READ, &val) == -1) { perror("ioctl read"); close(fd); return -1; } printf("Read value: %d ", val); close(fd); return 0; }
ioctl与write/read的区别
ioctl与write和read的主要区别在于用途和灵活性,write和read主要用于基本的数据传输,而ioctl则用于执行设备特定的控制操作,调整设备的配置参数或执行特定硬件操作,这使得ioctl在处理复杂设备控制时更加灵活和强大。
常见问题解答
Q1: ioctl命令的方向有哪些?如何选择合适的方向?
A1: ioctl命令的方向有四种:无数据传输(_IOC_NONE)、只读(_IOC_READ)、只写(_IOC_WRITE)和读写(_IOC_READ|_IOC_WRITE),选择合适的方向取决于具体操作的需求,如果只是发送命令而不涉及数据传输,选择无数据传输;如果需要从设备读取数据,选择只读;如果需要向设备写入数据,选择只写;如果需要同时读写数据,选择读写。
Q2: 如何在驱动中正确处理ioctl命令?
A2: 在驱动中正确处理ioctl命令的步骤如下:
1、在文件操作结构体中实现ioctl处理函数。
2、根据传入的命令号,使用switch语句区分不同的命令。
3、对于每个命令,根据需要执行相应的操作,如从用户空间复制数据、修改设备状态等。
4、确保所有操作成功完成后返回0,否则返回相应的错误码。
ioctl是一种强大的工具,通过合理的设计和实现,可以有效地控制系统中的设备,提高系统的灵活性和可维护性。
以上就是关于“linux驱动 ioctl”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1281688.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复