Android实现快递单号查询快递状态信息
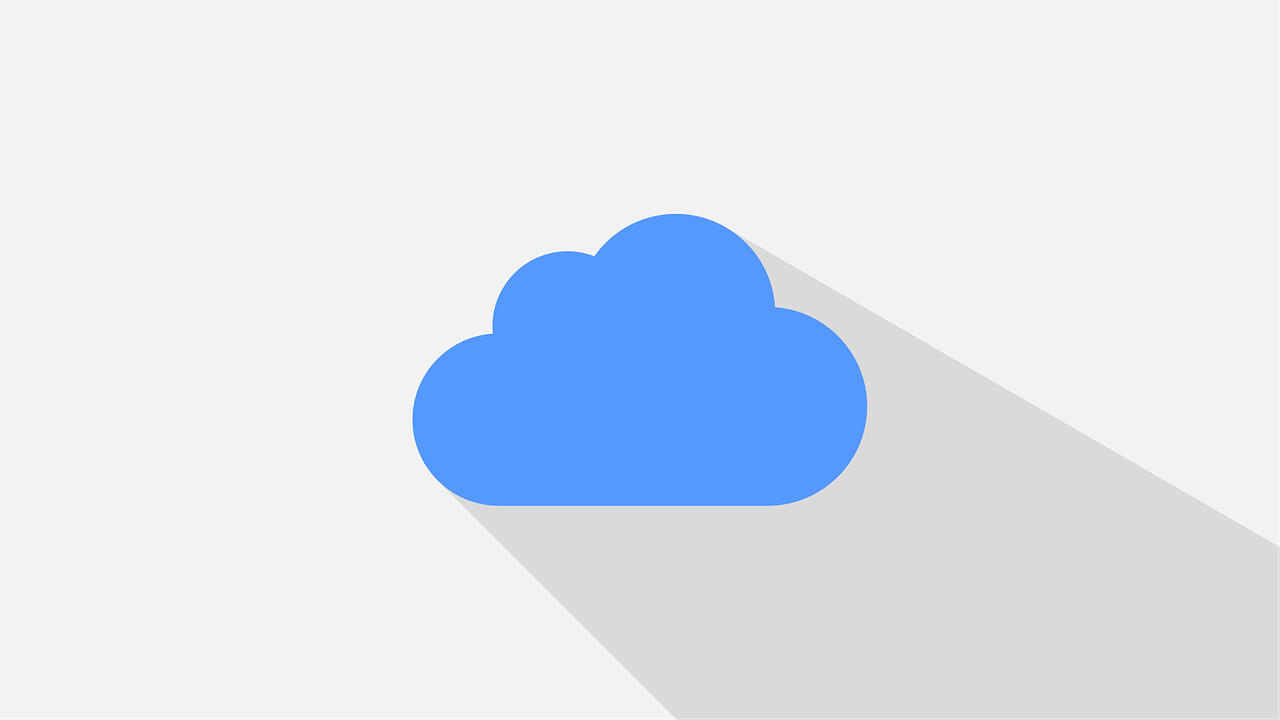
一、背景和目标
随着电子商务的迅猛发展,用户对物流信息的即时性和准确性需求日益增加,基于Android平台的快递单号查询应用可以方便用户随时随地获取快递状态信息,提高用户体验,本文将详细介绍如何在Android平台上实现一个快递单号查询功能,包括前端界面设计、后端接口调用、数据解析与展示等步骤。
二、开发环境设置
工具及依赖库
IDE:Android Studio
语言:Java/Kotlin
网络请求库:OkHttp
JSON解析库:Gson
配置build.gradle文件
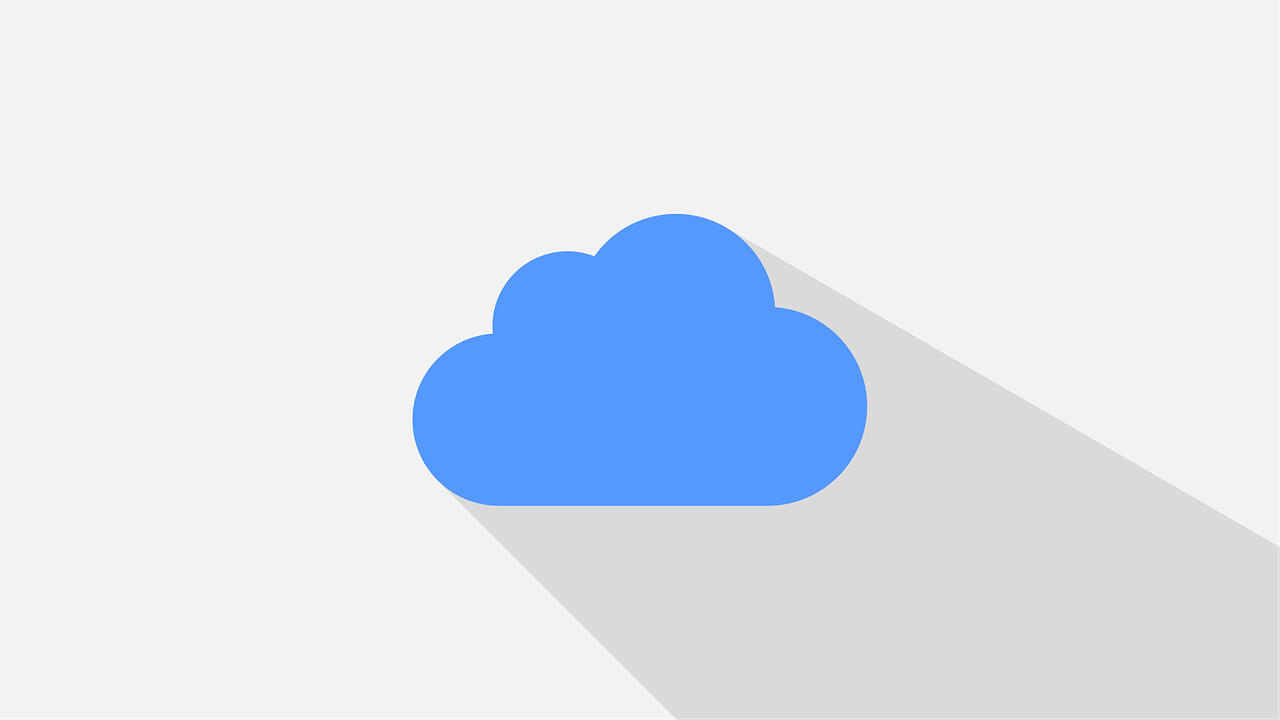
在项目的build.gradle
文件中添加OkHttp和Gson依赖:
dependencies { implementation 'com.squareup.okhttp3:okhttp:4.9.0' implementation 'com.google.code.gson:gson:2.8.6' }
三、前端界面设计
1. 布局文件activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:padding="16dp"> <Spinner android:id="@+id/delivery_company_spinner" android:layout_width="match_parent" android:layout_height="wrap_content" android:entries="@array/delivery_company" /> <EditText android:id="@+id/delivery_no_edit_text" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="请输入快递单号" android:inputType="number" /> <Button android:id="@+id/query_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="查询" /> <ListView android:id="@+id/messages_list_view" android:layout_width="match_parent" android:layout_height="wrap_content" /> </LinearLayout>
2. ListView的item布局文件list_item.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:padding="8dp"> <TextView android:id="@+id/time_text_view" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textStyle="bold" /> <TextView android:id="@+id/context_text_view" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout>
四、后端接口调用与数据解析
1. 创建HTTP请求工具类HttpUtil.java
package com.example.httputil; import okhttp3.Call; import okhttp3.Callback; import okhttp3.MediaType; import okhttp3.RequestBody; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response; import java.io.IOException; public class HttpUtil { private static final MediaType JSON = MediaType.parse("application/json; charset=utf-8"); private static final OkHttpClient client = new OkHttpClient(); public static void sendGetRequest(String url, Callback callback) { Request request = new Request.Builder().url(url).build(); Call call = client.newCall(request); call.enqueue(callback); } }
2. 创建主活动MainActivity.java
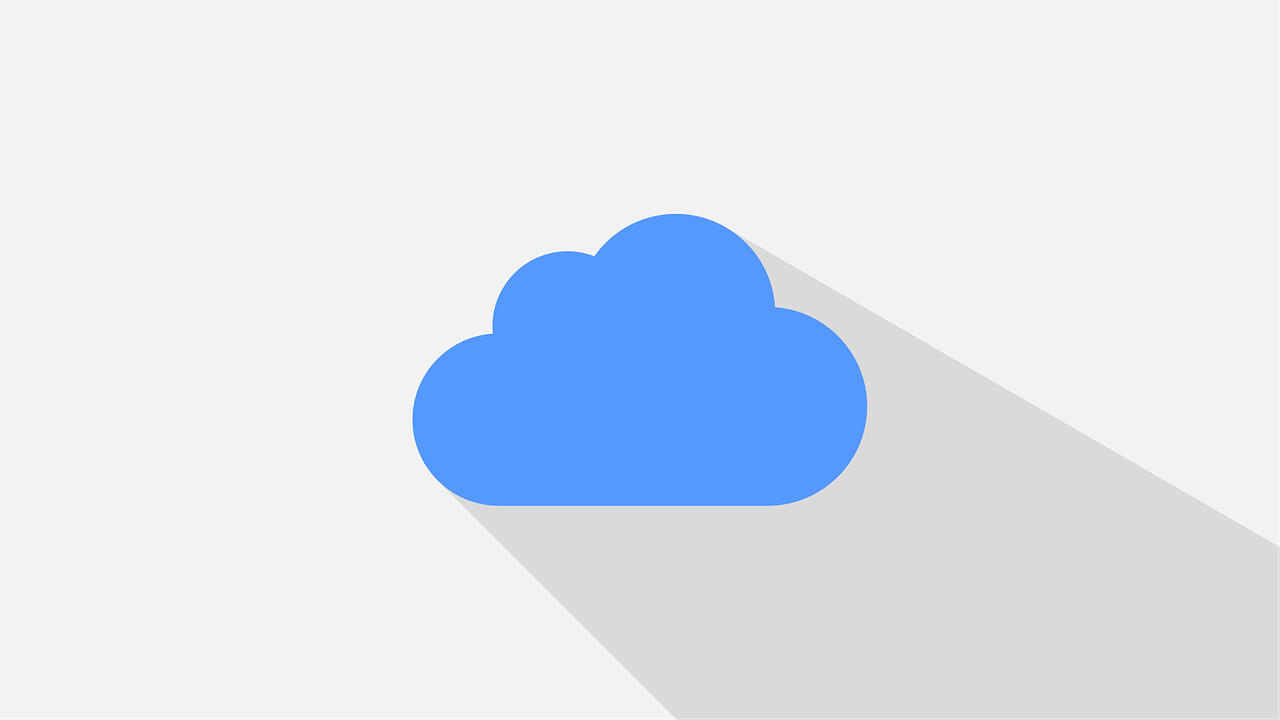
package com.example.logistics; import android.os.Bundle; import android.widget.ArrayAdapter; import android.widget.Button; import android.widget.EditText; import android.widget.ListView; import android.widget.Spinner; import androidx.appcompat.app.AppCompatActivity; import com.example.httputil.HttpUtil; import com.google.gson.Gson; import org.json.JSONArray; import org.json.JSONObject; import java.io.IOException; import java.util.ArrayList; import java.util.List; import okhttp3.Call; import okhttp3.Callback; import okhttp3.Response; public class MainActivity extends AppCompatActivity { private Spinner deliveryCompanySpinner; private EditText deliveryNoEditText; private Button queryButton; private ListView messagesListView; private ArrayAdapter<String> adapter; private List<String> messageList = new ArrayList<>(); private Gson gson = new Gson(); @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); deliveryCompanySpinner = findViewById(R.id.delivery_company_spinner); deliveryNoEditText = findViewById(R.id.delivery_no_edit_text); queryButton = findViewById(R.id.query_button); messagesListView = findViewById(R.id.messages_list_view); adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, messageList); messagesListView.setAdapter(adapter); queryButton.setOnClickListener(v -> { String companyName = deliveryCompanySpinner.getSelectedItem().toString(); String deliveryNo = deliveryNoEditText.getText().toString().trim(); String url = "https://api.kuaidi100.com/autonumber/autoComNum/?text=" + deliveryNo + "&key=YOUR_KEY"; // 替换为实际的API key HttpUtil.sendGetRequest(url, new Callback() { @Override public void onFailure(Call call, IOException e) { e.printStackTrace(); } @Override public void onResponse(Call call, Response response) throws IOException { if (response.isSuccessful()) { String jsonData = response.body().string(); Log.d("MainActivity", jsonData); // 打印JSON数据以便调试 try { JSONObject jsonObject = new JSONObject(jsonData); JSONArray dataArray = jsonObject.getJSONArray("data"); for (int i = 0; i < dataArray.length(); i++) { JSONObject itemObject = dataArray.getJSONObject(i); String time = itemObject.getString("time"); String context = itemObject.getString("context"); messageList.add(time + " " + context); } adapter.notifyDataSetChanged(); } catch (Exception e) { e.printStackTrace(); } } else { Log.d("MainActivity", "Failed to fetch data"); } } }); }); } }
五、测试与优化
测试步骤:
确保Android设备或模拟器已连接并运行。
编译并运行应用程序。
选择快递公司,输入快递单号,点击“查询”按钮。
查看ListView中是否显示了正确的快递状态信息。
常见问题与解决方案:
网络请求失败:检查网络连接,确保API地址正确。
JSON解析错误:确保返回的JSON格式正确,可以使用在线工具验证。
UI卡顿:考虑使用异步任务或Retrofit进行网络请求优化。
性能优化:
使用RecyclerView替代ListView以提高列表渲染效率。
在子线程中执行网络请求操作,避免阻塞主线程。
到此,以上就是小编对于“Android实现快递单号查询快递状态信息”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1279985.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复