Android 实现单选按钮功能
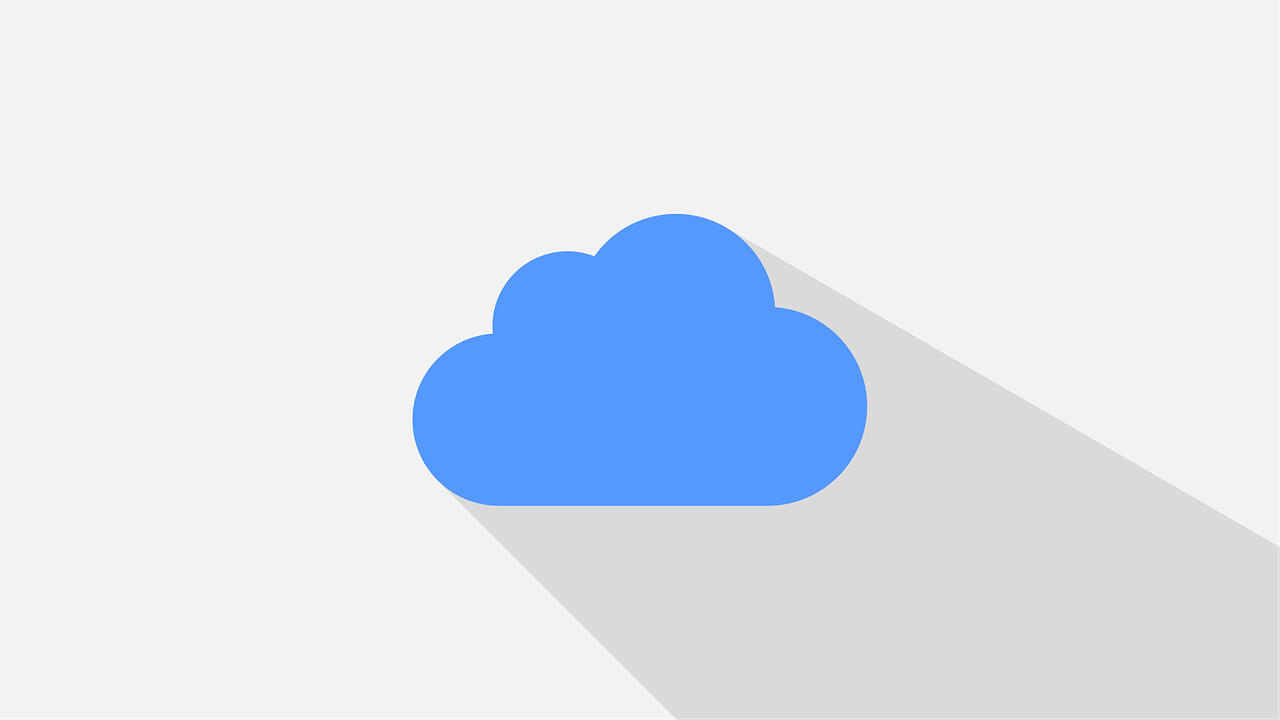
在 Android 开发中,单选按钮(RadioButton)是常用的 UI 组件之一,它允许用户从多个选项中选择一个选项,本文将详细介绍如何在 Android 应用中实现单选按钮功能,包括创建单选按钮、设置属性、处理用户交互等。
1. 创建单选按钮
1 在布局文件中添加单选按钮
我们需要在布局文件(例如activity_main.xml
)中添加单选按钮,我们可以使用RadioGroup
来包含多个RadioButton
,以便用户只能选择其中一个选项。
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选择一个选项:" android:padding="16dp"/> <RadioGroup android:id="@+id/radioGroup" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="16dp"> <RadioButton android:id="@+id/radioButton1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选项1"/> <RadioButton android:id="@+id/radioButton2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选项2"/> <RadioButton android:id="@+id/radioButton3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选项3"/> </RadioGroup> </LinearLayout>
2 在活动中初始化单选按钮
在MainActivity
中初始化RadioGroup
和RadioButton
,并设置默认选中的选项。
package com.example.radiobuttondemo; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.widget.RadioButton; import android.widget.RadioGroup; import android.widget.Toast; public class MainActivity extends AppCompatActivity { private RadioGroup radioGroup; private RadioButton radioButton1; private RadioButton radioButton2; private RadioButton radioButton3; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); radioGroup = findViewById(R.id.radioGroup); radioButton1 = findViewById(R.id.radioButton1); radioButton2 = findViewById(R.id.radioButton2); radioButton3 = findViewById(R.id.radioButton3); // 设置默认选中的选项 radioButton1.setChecked(true); // 为 RadioGroup 设置监听器 radioGroup.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() { @Override public void onCheckedChanged(RadioGroup group, int checkedId) { switch (checkedId) { case R.id.radioButton1: Toast.makeText(MainActivity.this, "选择了选项1", Toast.LENGTH_SHORT).show(); break; case R.id.radioButton2: Toast.makeText(MainActivity.this, "选择了选项2", Toast.LENGTH_SHORT).show(); break; case R.id.radioButton3: Toast.makeText(MainActivity.this, "选择了选项3", Toast.LENGTH_SHORT).show(); break; } } }); } }
2. 设置单选按钮的属性
1 更改文本颜色和大小
可以通过在布局文件中设置属性来更改单选按钮的文本颜色和大小。
<RadioButton android:id="@+id/radioButton1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选项1" android:textColor="#FF0000" android:textSize="18sp"/>
2 禁用或启用单选按钮
可以使用setEnabled(boolean enabled)
方法来启用或禁用单选按钮。
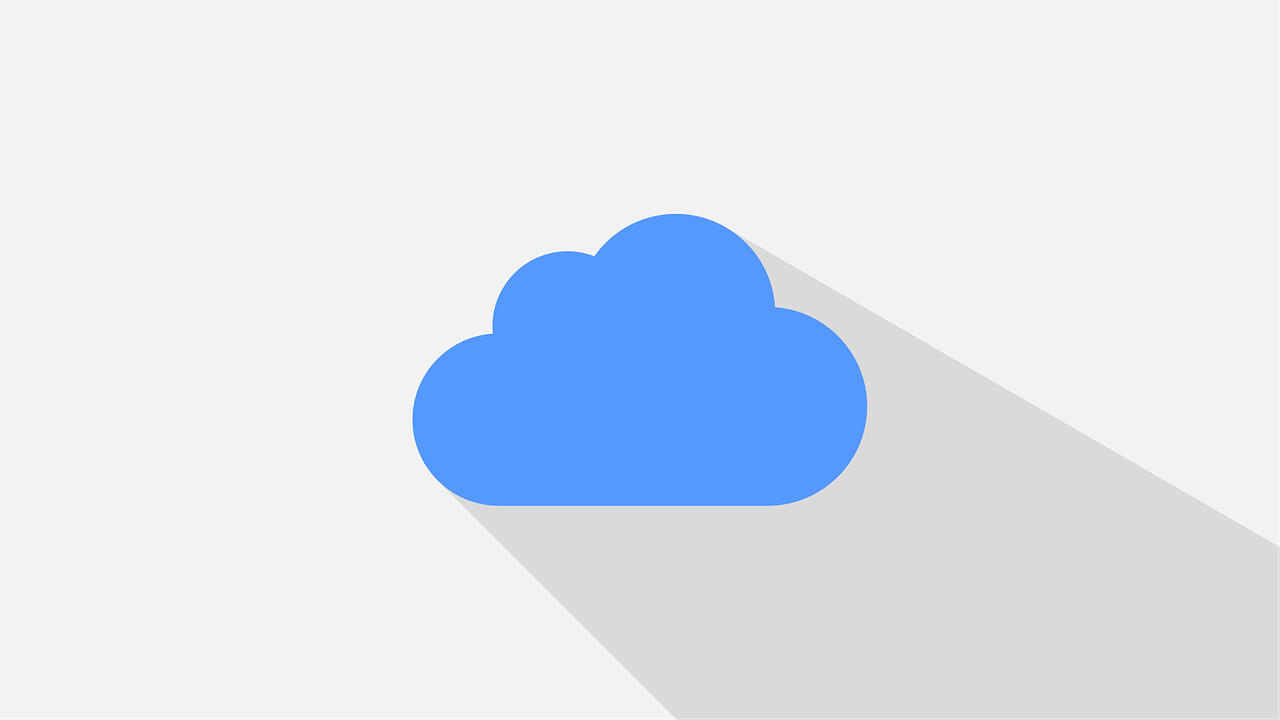
radioButton1.setEnabled(false); // 禁用单选按钮1 radioButton2.setEnabled(true); // 启用单选按钮2
3 设置单选按钮的图标
可以为单选按钮设置图标,以增强视觉效果,可以在布局文件中使用android:button
属性指定图标资源。
<RadioButton android:id="@+id/radioButton1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选项1" android:button="@drawable/ic_radio_button"/>
3. 处理用户交互
1 获取选中的选项
可以通过调用radioGroup.getCheckedRadioButtonId()
方法获取当前选中的单选按钮的 ID。
int selectedId = radioGroup.getCheckedRadioButtonId(); switch (selectedId) { case R.id.radioButton1: // 处理选项1被选中的情况 break; case R.id.radioButton2: // 处理选项2被选中的情况 break; case R.id.radioButton3: // 处理选项3被选中的情况 break; }
2 动态添加或删除单选按钮
可以在运行时动态添加或删除单选按钮,要动态添加一个单选按钮,可以执行以下操作:
RadioButton newRadioButton = new RadioButton(this); newRadioButton.setText("新选项"); radioGroup.addView(newRadioButton);
要删除一个单选按钮,可以执行以下操作:
radioGroup.removeView(radioButton2);
4. 示例代码汇总
以下是一个完整的示例代码,展示了如何在 Android 应用中实现单选按钮功能:
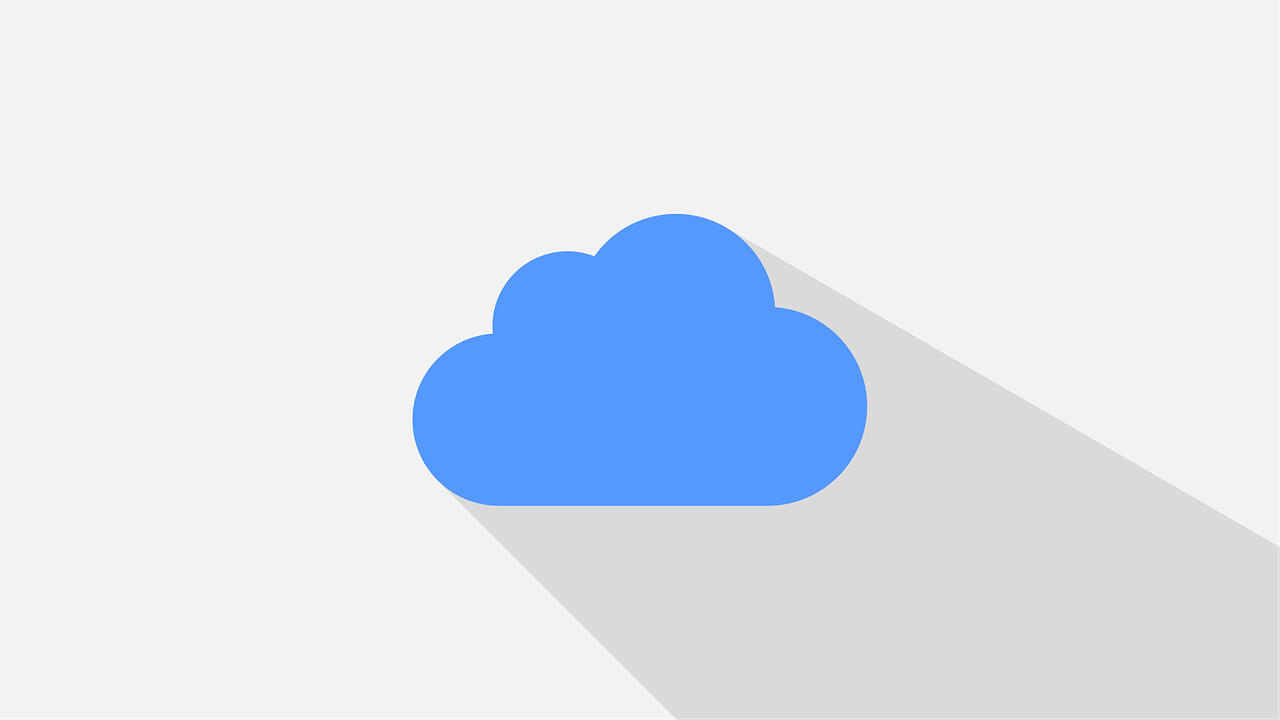
<!-activity_main.xml --> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选择一个选项:" android:padding="16dp"/> <RadioGroup android:id="@+id/radioGroup" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="16dp"> <RadioButton android:id="@+id/radioButton1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选项1"/> <RadioButton android:id="@+id/radioButton2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选项2"/> <RadioButton android:id="@+id/radioButton3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选项3"/> </RadioGroup> </LinearLayout>
// MainActivity.java package com.example.radiobuttondemo; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.widget.RadioButton; import android.widget.RadioGroup; import android.widget.Toast; public class MainActivity extends AppCompatActivity { private RadioGroup radioGroup; private RadioButton radioButton1; private RadioButton radioButton2; private RadioButton radioButton3; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); radioGroup = findViewById(R.id.radioGroup); radioButton1 = findViewById(R.id.radioButton1); radioButton2 = findViewById(R.id.radioButton2); radioButton3 = findViewById(R.id.radioButton3); // 设置默认选中的选项 radioButton1.setChecked(true); // 为 RadioGroup 设置监听器 radioGroup.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() { @Override public void onCheckedChanged(RadioGroup group, int checkedId) { switch (checkedId) { case R.id.radioButton1: Toast.makeText(MainActivity.this, "选择了选项1", Toast.LENGTH_SHORT).show(); break; case R.id.radioButton2: Toast.makeText(MainActivity.this, "选择了选项2", Toast.LENGTH_SHORT).show(); break; case R.id.radioButton3: Toast.makeText(MainActivity.this, "选择了选项3", Toast.LENGTH_SHORT).show(); break; } } }); } }
涵盖了在 Android 应用中实现单选按钮功能的基本步骤和常见操作,包括创建单选按钮、设置属性、处理用户交互等,希望这些内容对你有所帮助!
各位小伙伴们,我刚刚为大家分享了有关“android实现单选按钮功能”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1279775.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复