scanf
是一个标准输入函数,用于从标准输入(通常是键盘)读取格式化数据并存储到指定的变量中。Scanf in Linux: A Comprehensive Guide
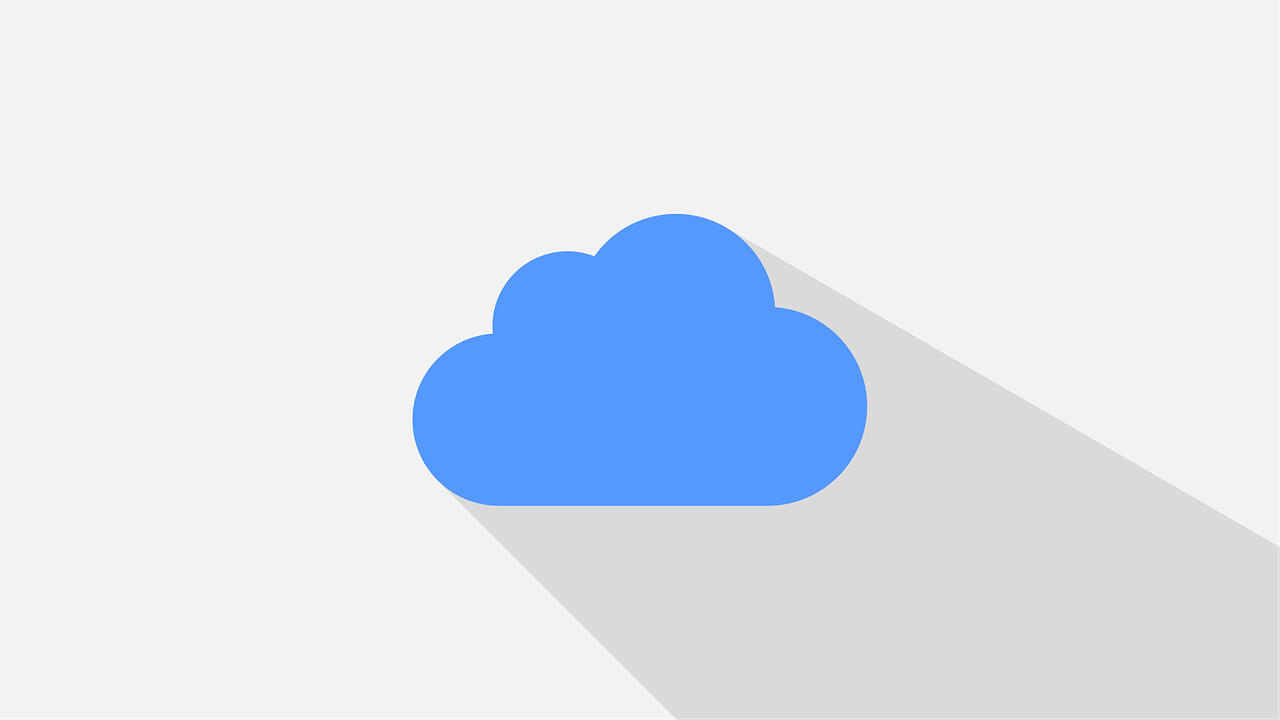
Introduction to scanf in Linux
In the world of C programming,scanf
is a fundamental function used for reading formatted input from the standard input (stdin). This can be particularly useful when developing programs on Linux systems where console-based user interaction is common. This guide will delve into the intricacies of usingscanf
, covering its syntax, usage, and potential pitfalls.
Basic Syntax and Usage
The basic syntax ofscanf
is as follows:
int scanf(const char *format, ...);
Parameters:
1、format: A string that specifies the types and number of input items to read.
2、…: A variable number of pointer arguments corresponding to the format specifiers in the format string.
Example Usage:
Here’s a simple example demonstrating how to usescanf
to read an integer and a floating-point number from the user:
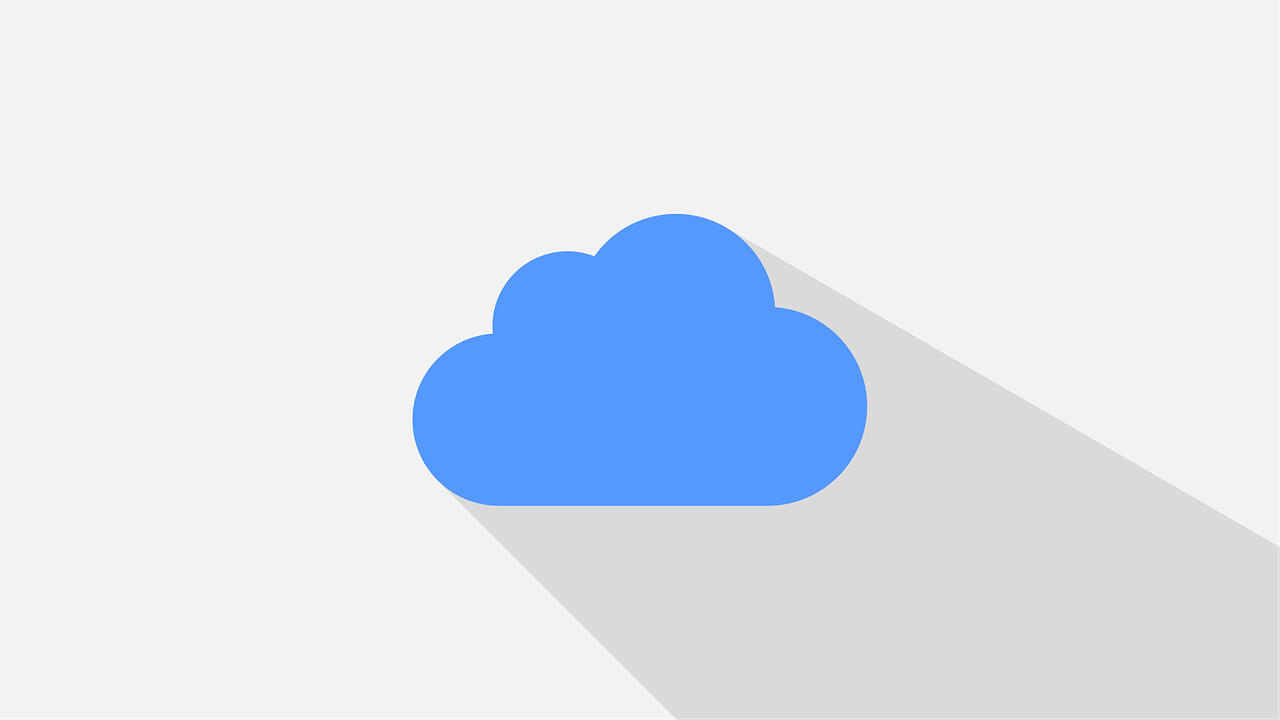
#include <stdio.h> int main() { int num; float fnum; printf("Enter an integer: "); scanf("%d", &num); printf("Enter a floating-point number: "); scanf("%f", &fnum); printf("You entered: %d and %f ", num, fnum); return 0; }
Output Explanation:
The%d
format specifier is used to read an integer.
The%f
format specifier is used to read a floating-point number.
The addresses ofnum
andfnum
are passed toscanf
to store the input values.
Common Format Specifiers
Here is a table summarizing some commonly used format specifiers inscanf
:
Format Specifier | Description | Example |
%d | Read an integer | int x; scanf("%d", &x); |
%f | Read a floating point number | float y; scanf("%f", &y); |
%c | Read a single character | char z; scanf(" %c", &z); |
%s | Read a string (until whitespace) | char str[100]; scanf("%s", str); |
%lf | Read a double precision float | double d; scanf("%lf", &d); |
%x | Read an integer in hexadecimal | int h; scanf("%x", &h); |
%o | Read an integer in octal | int o; scanf("%o", &o); |
%u | Read an unsigned integer | unsigned int u; scanf("%u", &u); |
Handling Whitespace and Newlines
One of the challenges withscanf
is handling whitespace and newline characters effectively. Here are some tips:
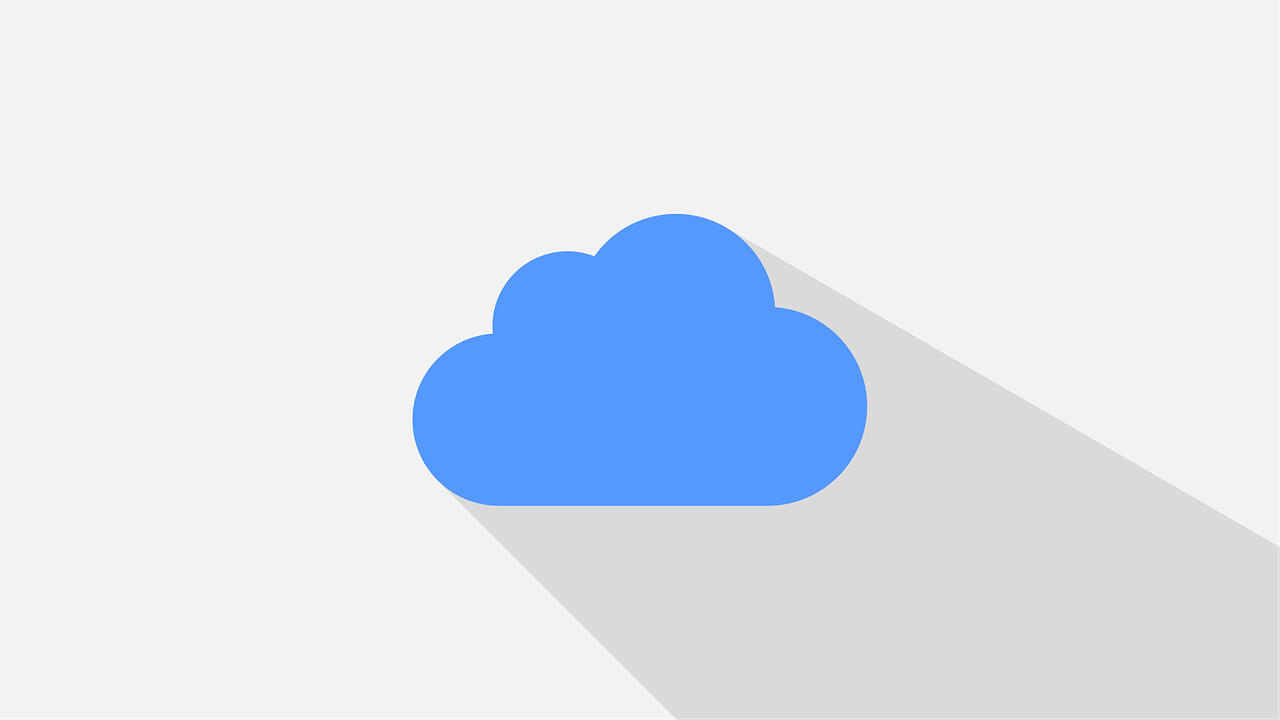
Use the space character () in the format string to skip any whitespace characters (including spaces, tabs, and newlines) before the actual input.
Use the newline character (`
) to consume the remaining characters in the input buffer after a
scanf` call.
For strings, consider usingfgets
instead ofscanf
to avoid issues with whitespace.
Example to handle whitespace:
#include <stdio.h> int main() { char str[100]; printf("Enter a string: "); scanf(" %99[^ ]", str); // Space before % ensures leading whitespace is ignored printf("You entered: '%s' ", str); return 0; }
Important Note:
The `%99[^
]` format specifier reads up to 99 characters until a newline is encountered, ensuring no buffer overflow.
Error Handling with scanf
scanf
returns the number of input items successfully matched and assigned, which can be used to check if the input operation was successful. Here’s an example:
#include <stdio.h> int main() { int num; printf("Enter an integer: "); if (scanf("%d", &num) != 1) { printf("Invalid input! "); return 1; // Exit with error code } printf("You entered: %d ", num); return 0; }
Tips for Robust Error Handling:
Always check the return value ofscanf
.
Clear the input buffer if necessary using functions like `while (getchar() != ‘
‘);` to discard unwanted characters.
Advanced Usage: Limiting Input Length and Using Negative Format Specifiers
To limit the number of characters read byscanf
, you can use a width specifier before the format specifier. For instance, to read at most 5 characters into a string:
char str[6]; // Extra space for null terminator scanf("%5s", str); // Reads at most 5 characters
Negative format specifiers can be used to skip input. For example,%*d
skips an integer input without storing it:
int main() { int num1, num2; printf("Enter two integers: "); scanf("%d %*d %d", &num1, &num2); // First integer stored in num1, second skipped, third stored in num2 printf("First: %d, Second: %d ", num1, num2); return 0; }
Conclusion
Masteringscanf
in Linux is essential for effective console input handling in C programs. By understanding its syntax, format specifiers, and best practices for error handling, you can create more robust and user-friendly applications. Remember to always validate user inputs and handle errors gracefully to ensure the reliability and security of your programs.
以上就是关于“scanf linux”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1278944.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复