在Android应用开发中,实现底部菜单和顶部导航是一种常见的设计模式,底部菜单通常用于提供快速访问主要功能或视图的入口,而顶部导航则用于展示应用的品牌标识、标题或其他重要信息,以下是关于如何在Android中实现这两种导航组件的详细步骤和小标题说明:
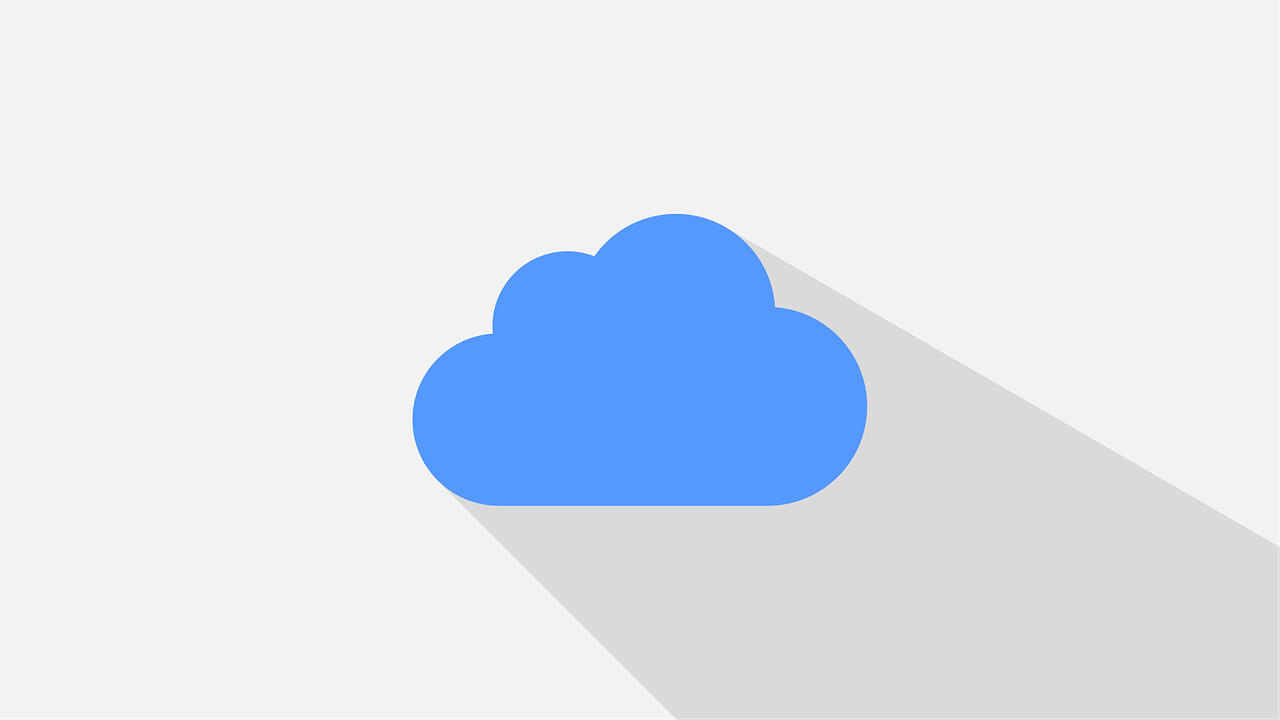
一、创建底部菜单(Bottom Navigation)
1. 添加依赖
确保在你的build.gradle
文件中添加了必要的依赖项:
dependencies { implementation 'com.google.android.material:material:1.4.0' }
2. 定义菜单资源
在res/menu
目录下创建一个名为bottom_nav_menu.xml
的文件,并定义底部菜单的结构:
<menu xmlns:android="http://schemas.android.com/apk/res/android"> <item android:id="@+id/navigation_home" android:icon="@drawable/ic_home" android:title="Home" /> <item android:id="@+id/navigation_dashboard" android:icon="@drawable/ic_dashboard" android:title="Dashboard" /> <item android:id="@+id/navigation_notifications" android:icon="@drawable/ic_notifications" android:title="Notifications" /> </menu>
3. 布局文件
在你的主布局文件(例如activity_main.xml
)中添加BottomNavigationView:
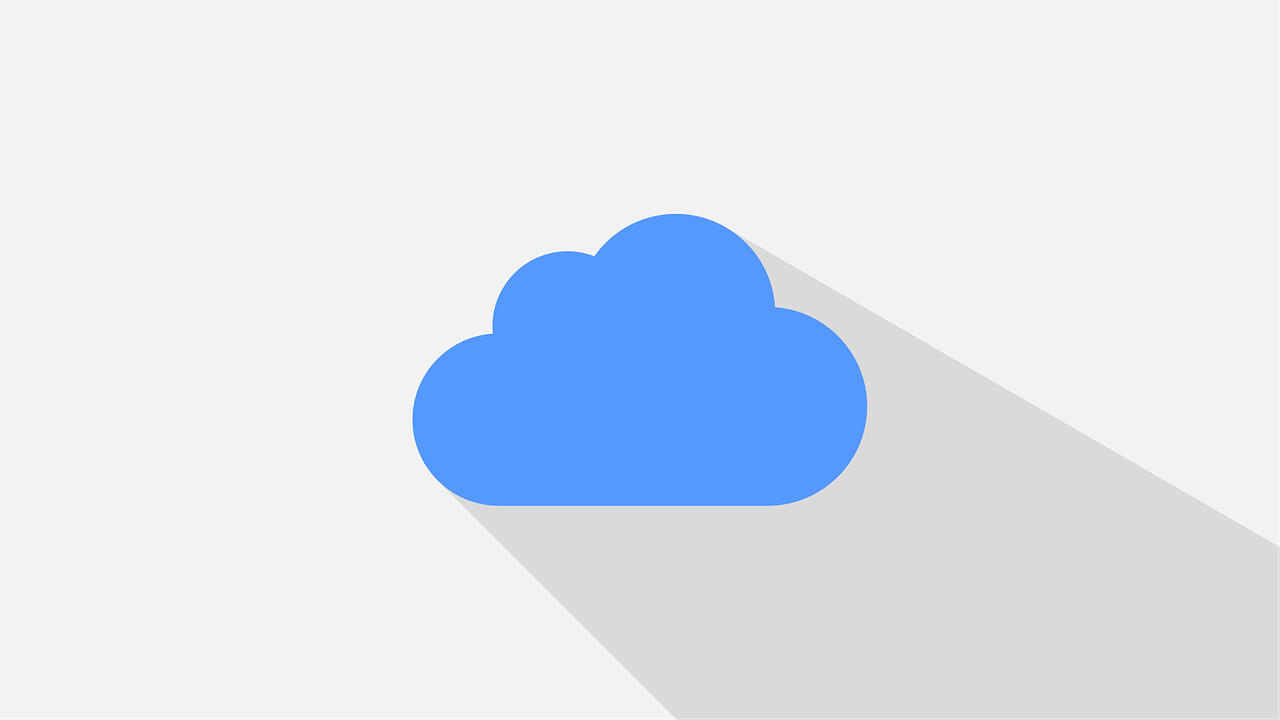
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <FrameLayout android:id="@+id/container" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_above="@id/navigation" /> <com.google.android.material.bottomnavigation.BottomNavigationView android:id="@+id/navigation" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:background="?android:attr/windowBackground" app:menu="@menu/bottom_nav_menu" /> </RelativeLayout>
4. 活动代码
在你的主活动(例如MainActivity.java
)中设置BottomNavigationView的行为:
public class MainActivity extends AppCompatActivity { private BottomNavigationView navigation; private FrameLayout container; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); navigation = findViewById(R.id.navigation); container = findViewById(R.id.container); navigation.setOnNavigationItemSelectedListener(new BottomNavigationView.OnNavigationItemSelectedListener() { @Override public boolean onNavigationItemSelected(@NonNull MenuItem item) { switch (item.getItemId()) { case R.id.navigation_home: loadFragment(new HomeFragment()); return true; case R.id.navigation_dashboard: loadFragment(new DashboardFragment()); return true; case R.id.navigation_notifications: loadFragment(new NotificationsFragment()); return true; } return false; } }); } private void loadFragment(Fragment fragment) { // 切换到新的Fragment FragmentTransaction transaction = getSupportFragmentManager().beginTransaction(); transaction.replace(R.id.container, fragment); transaction.addToBackStack(null); transaction.commit(); } }
二、创建顶部导航(Top App Bar)
1. 添加依赖
如果你还没有添加Material Components库,请参考底部菜单的步骤1。
2. 定义菜单资源
在res/menu
目录下创建一个名为top_app_bar_menu.xml
的文件,并定义顶部导航菜单的结构:
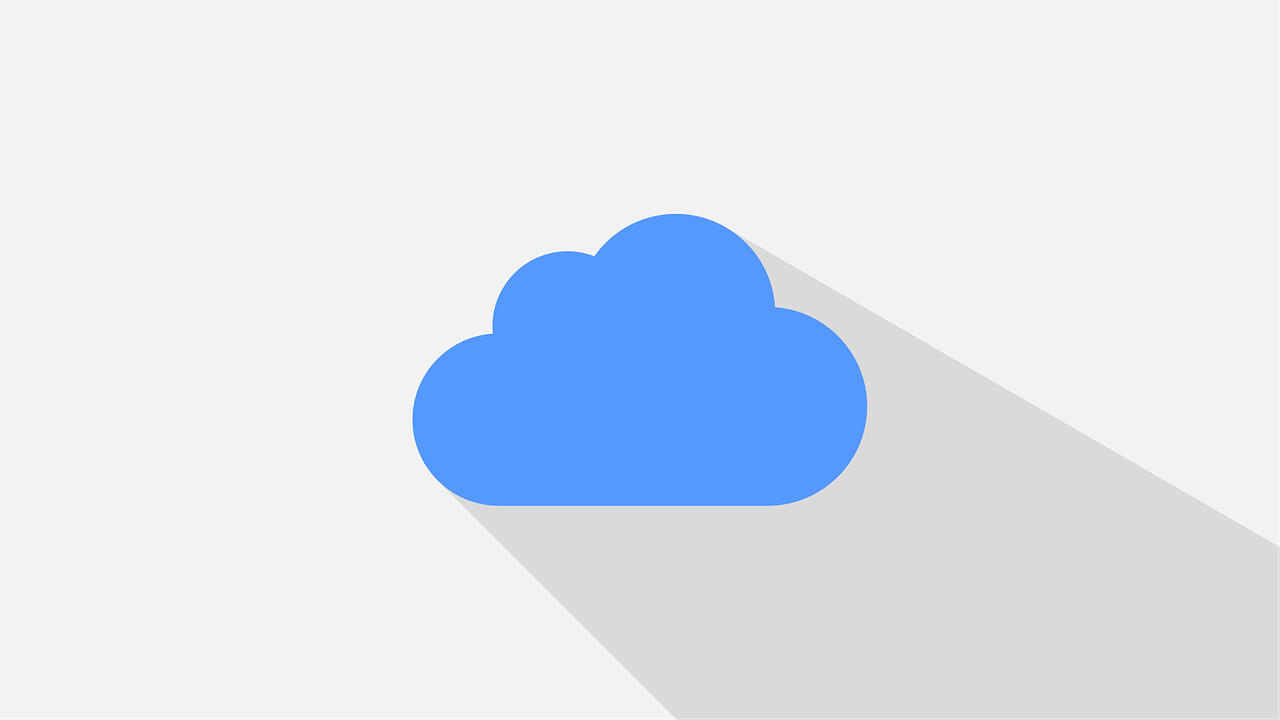
<menu xmlns:android="http://schemas.android.com/apk/res/android"> <item android:id="@+id/action_settings" android:icon="@drawable/ic_settings" android:title="Settings" /> </menu>
3. 布局文件
在你的主布局文件(例如activity_main.xml
)中添加Toolbar作为顶部导航:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <androidx.appcompat.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" android:popupTheme="@style/ThemeOverlay.AppCompat.Light" app:titleTextColor="@android:color/white" /> <!-...其他布局... --> </RelativeLayout>
4. 活动代码
在你的主活动(例如MainActivity.java
)中设置Toolbar的行为:
public class MainActivity extends AppCompatActivity { private Toolbar toolbar; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); toolbar = findViewById(R.id.toolbar); setSupportActionBar(toolbar); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.top_app_bar_menu, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } }
是一个简单的例子,展示了如何在Android应用中实现底部菜单和顶部导航,你可以根据需要自定义菜单项和布局,以及添加更多的交互逻辑来满足你的应用需求。
以上内容就是解答有关“Android实现底部菜单和顶部导航”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1278846.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复