Android实现底部导航栏
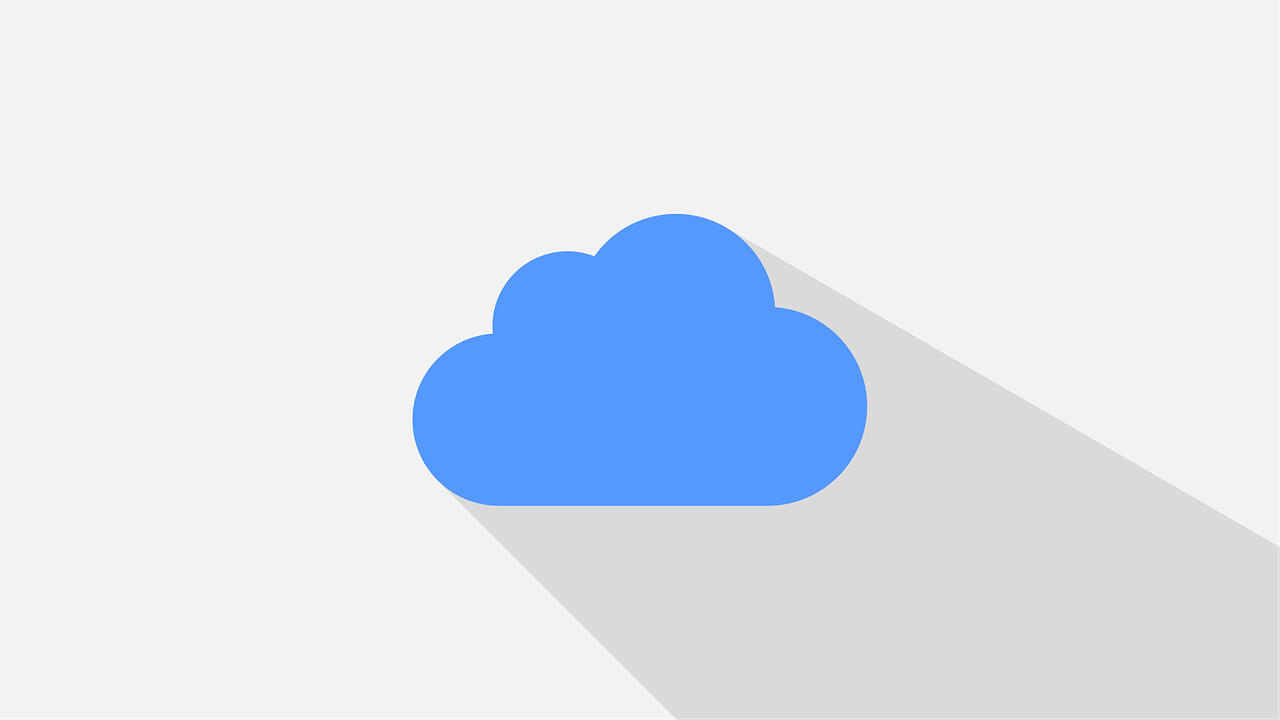
底部导航栏(Bottom Navigation Bar)是Android应用程序中常见的一种导航模式,它通过在屏幕底部显示多个操作选项,让用户可以快速切换不同的功能模块,本文将详细介绍如何在Android应用中实现底部导航栏,包括创建项目、设计布局、编写逻辑代码以及测试和优化。
一、准备工作
创建新项目
我们需要在Android Studio中创建一个新的项目,打开Android Studio,选择“Start a new Android Studio project”,然后按照以下步骤进行配置:
Project Name: 输入你的项目名称,例如BottomNavigationDemo
Package name: 输入包名,例如com.example.bottomnavigationdemo
Save location: 选择项目的保存位置
Language: 选择Java或Kotlin作为编程语言
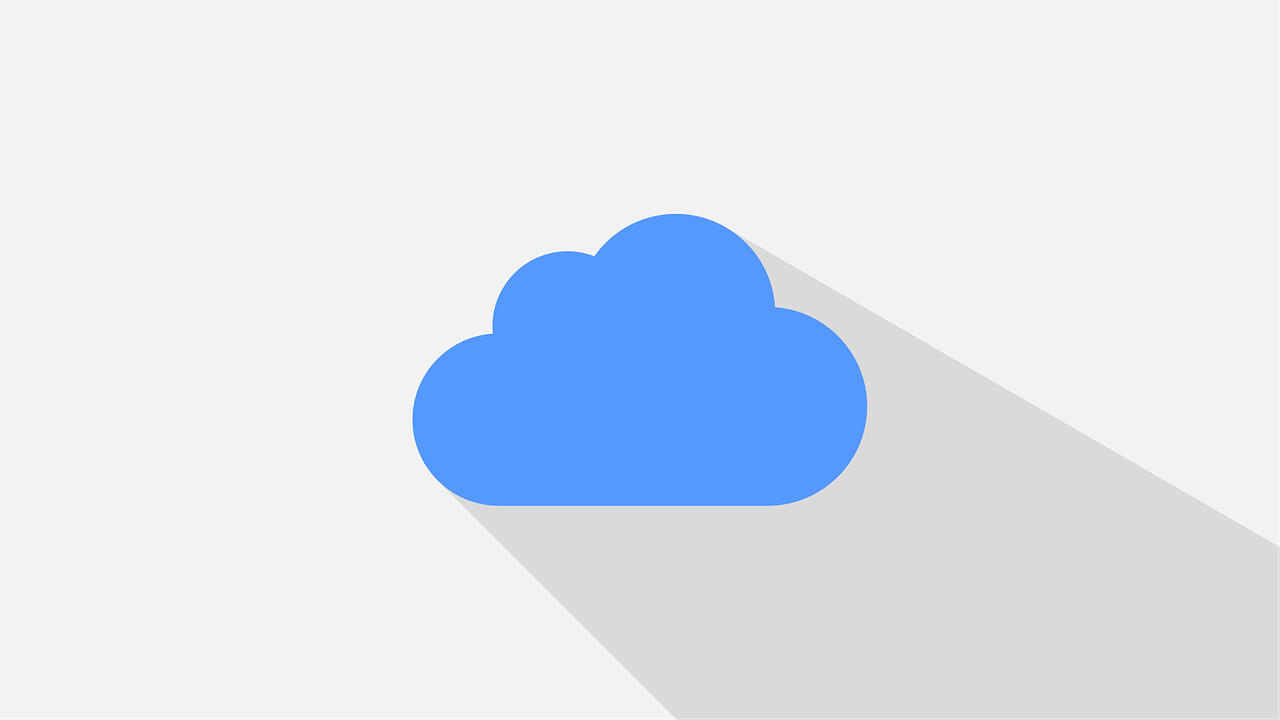
Minimum API level: 选择API 21(Lollipop)或更高版本
点击“Finish”完成项目创建。
添加依赖项
我们需要在项目的build.gradle
文件中添加必要的依赖项,打开app/build.gradle
文件,并添加以下内容到dependencies
部分:
implementation 'androidx.appcompat:appcompat:1.3.0' implementation 'com.google.android.material:material:1.4.0'
这些依赖项提供了AppCompat库和Material Design组件,后者包含了底部导航栏所需的BottomNavigationView
控件。
二、设计布局
主布局文件
在res/layout/activity_main.xml
中定义主布局文件,我们将使用BottomNavigationView
作为底部导航栏,并通过一个FrameLayout
容器来加载不同的Fragment。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <FrameLayout android:id="@+id/container" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_above="@id/bottom_navigation" /> <com.google.android.material.bottomnavigation.BottomNavigationView android:id="@+id/bottom_navigation" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" app:menu="@menu/bottom_navigation_menu" /> </RelativeLayout>
菜单资源文件
创建一个菜单资源文件res/menu/bottom_navigation_menu.xml
,用于定义底部导航栏的菜单项,每个菜单项对应一个Fragment。
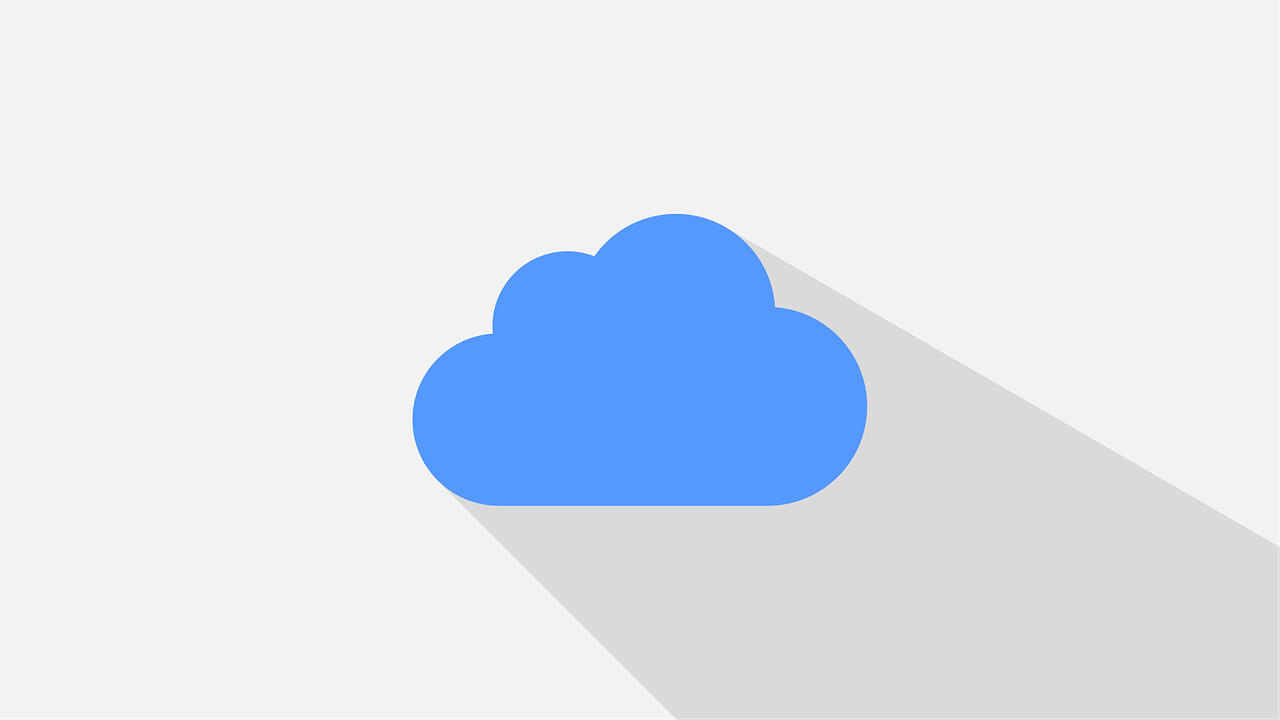
<?xml version="1.0" encoding="utf-8"?> <menu xmlns:android="http://schemas.android.com/apk/res/android"> <item android:id="@+id/navigation_home" android:icon="@drawable/ic_home" android:title="Home" /> <item android:id="@+id/navigation_dashboard" android:icon="@drawable/ic_dashboard" android:title="Dashboard" /> <item android:id="@+id/navigation_notifications" android:icon="@drawable/ic_notifications" android:title="Notifications" /> </menu>
注意:你需要在res/drawable
目录下添加对应的图标文件(ic_home.xml
,ic_dashboard.xml
,ic_notifications.xml
),或者使用其他现有的图标资源。
三、编写逻辑代码
MainActivity类
在MainActivity
中实现底部导航栏的功能,我们需要为每个菜单项设置点击事件,并在相应的Fragment之间切换。
package com.example.bottomnavigationdemo; import android.os.Bundle; import androidx.annotation.NonNull; import androidx.appcompat.app.AppCompatActivity; import androidx.fragment.app.Fragment; import androidx.fragment.app.FragmentManager; import androidx.fragment.app.FragmentTransaction; import com.google.android.material.bottomnavigation.BottomNavigationView; public class MainActivity extends AppCompatActivity { private BottomNavigationView bottomNavigationView; private FragmentManager fragmentManager; private HomeFragment homeFragment; private DashboardFragment dashboardFragment; private NotificationsFragment notificationsFragment; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); bottomNavigationView = findViewById(R.id.bottom_navigation); fragmentManager = getSupportFragmentManager(); // 初始化Fragment homeFragment = new HomeFragment(); dashboardFragment = new DashboardFragment(); notificationsFragment = new NotificationsFragment(); // 默认显示HomeFragment if (savedInstanceState == null) { switchFragment(homeFragment); } else { // 恢复状态 switchFragment(findFragmentById(R.id.container)); } bottomNavigationView.setOnNavigationItemSelectedListener(item -> { switch (item.getItemId()) { case R.id.navigation_home: switchFragment(homeFragment); return true; case R.id.navigation_dashboard: switchFragment(dashboardFragment); return true; case R.id.navigation_notifications: switchFragment(notificationsFragment); return true; } return false; }); } private void switchFragment(Fragment fragment) { FragmentTransaction transaction = fragmentManager.beginTransaction(); transaction.replace(R.id.container, fragment); transaction.commit(); } }
Fragment类
创建三个简单的Fragment类,分别代表底部导航栏中的三个选项卡,以下是HomeFragment
的示例:
package com.example.bottomnavigationdemo; import android.os.Bundle; import androidx.fragment.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; public class HomeFragment extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment return inflater.inflate(R.layout.fragment_home, container, false); } }
类似地,创建DashboardFragment
和NotificationsFragment
,并分别为它们指定不同的布局文件(如fragment_dashboard.xml
和fragment_notifications.xml
)。
四、测试和优化
运行应用
连接真机或启动模拟器,点击运行按钮,观察底部导航栏是否正常工作,你可以通过点击不同的菜单项来切换不同的Fragment。
优化用户体验
为了提升用户体验,你可以进一步优化以下几点:
选中状态:确保底部导航栏在不同的菜单项被选中时有明确的视觉反馈(如颜色变化),可以在res/color/bottom_navigation_colors.xml
中定义选中和未选中的颜色。
动画效果:为Fragment切换添加过渡动画,使用户界面更加流畅,可以使用FragmentTransaction
的setCustomAnimations
方法来实现。
响应式设计:确保底部导航栏在不同尺寸的设备上都有良好的显示效果,特别是在大屏幕设备上可能需要调整布局。
性能优化:避免在Fragment中加载过多的数据或执行耗时操作,以免影响应用的响应速度,可以考虑使用异步任务或ViewModel来管理数据。
五、归纳
通过以上步骤,你已经成功在Android应用中实现了一个基本的底部导航栏,这个底部导航栏允许用户在不同的功能模块之间快速切换,提升了应用的可用性和用户体验,根据实际需求,你可以进一步扩展和完善底部导航栏的功能,例如添加更多菜单项、支持动态更新菜单等,希望本文对你有所帮助!
各位小伙伴们,我刚刚为大家分享了有关“android实现底部导航栏”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1278587.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复