java.util.zip
包来实现文件或文件夹的压缩。首先创建ZipOutputStream
对象,然后遍历文件或文件夹,将每个文件添加到压缩包中。在Android应用开发中,实现文件或文件夹的压缩是一个常见需求,本文将详细介绍如何在Android平台上实现这一功能,包括使用Java和第三方库进行文件压缩。
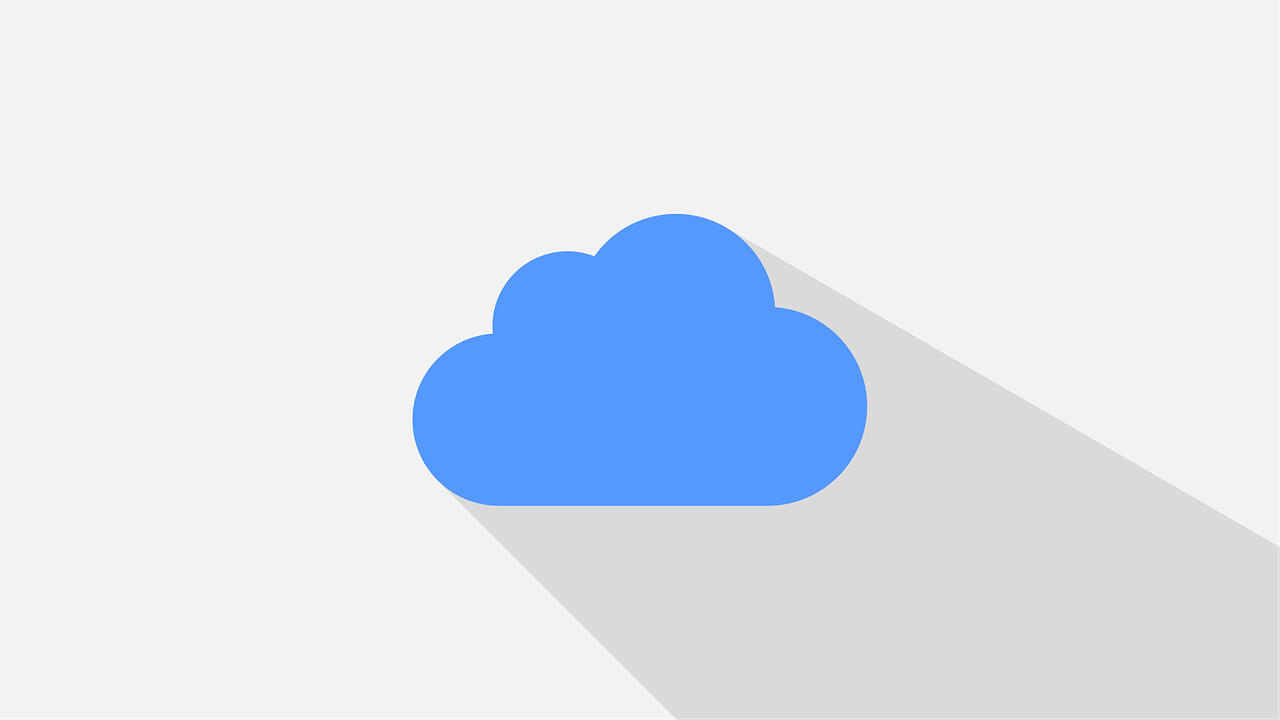
文件压缩是数据存储和传输过程中常用的技术,可以有效减少文件大小,节省存储空间和提高传输效率,在Android开发中,我们可以通过Java自带的压缩工具类或者引入第三方库来实现文件或文件夹的压缩,下面将分别介绍这两种方法。
二、使用Java内置工具类进行文件压缩
1. 准备工作
确保你的Android项目中已经添加了必要的权限,如读写存储权限:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
并在AndroidManifest.xml
中声明这些权限。
2. 压缩单个文件
Java提供了java.util.zip
包来处理ZIP格式的压缩和解压缩,以下是一个简单的示例,演示如何将一个文件压缩成ZIP格式:
import java.io.*; import java.util.zip.*; public class FileCompressor { public static void compressFile(String filePath, String zipFilePath) { byte[] buffer = new byte[1024]; try { FileOutputStream fos = new FileOutputStream(zipFilePath); ZipOutputStream zos = new ZipOutputStream(fos); ZipEntry ze = new ZipEntry(new File(filePath).getName()); zos.putNextEntry(ze); FileInputStream in = new FileInputStream(filePath); int len; while ((len = in.read(buffer)) > 0) { zos.write(buffer, 0, len); } in.close(); zos.closeEntry(); zos.close(); } catch (IOException e) { e.printStackTrace(); } } }
3. 压缩文件夹
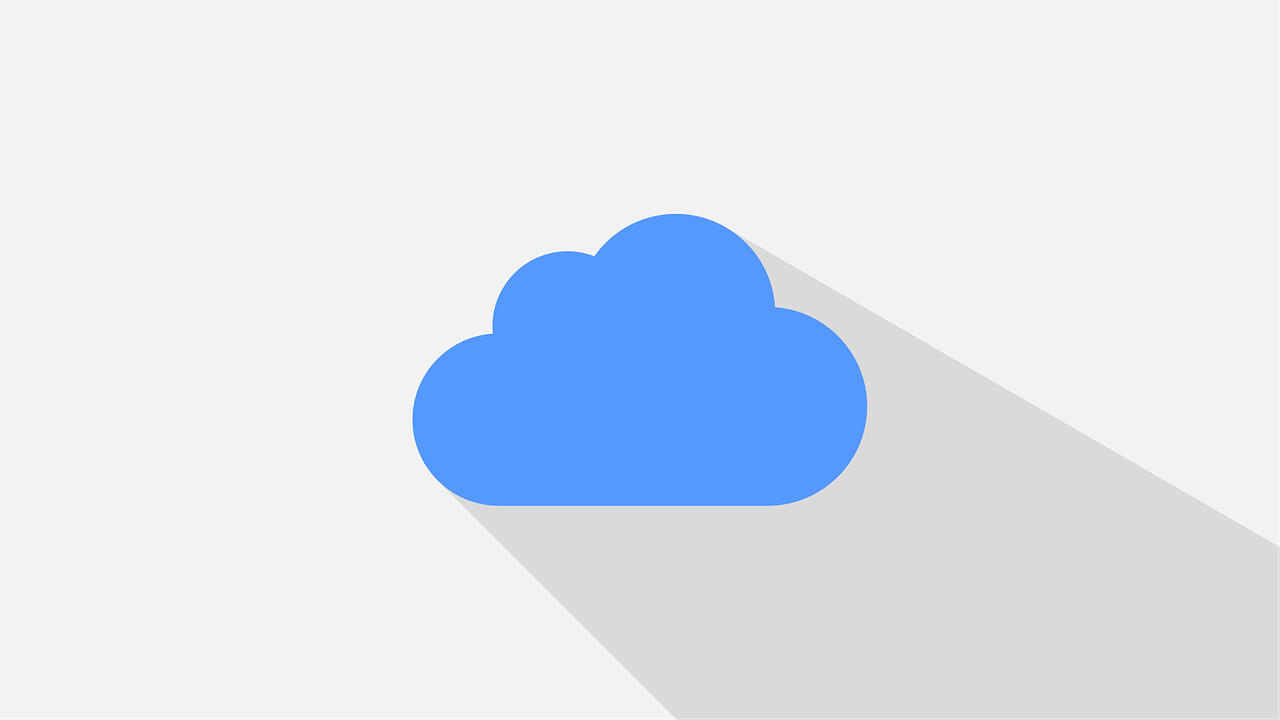
压缩文件夹比压缩单个文件稍微复杂一些,因为需要递归遍历文件夹中的所有文件,以下是一个示例代码:
import java.io.*; import java.util.zip.*; public class FolderCompressor extends FileCompressor { public static void compressFolder(String sourceFolder, String zipFilePath) { byte[] buffer = new byte[1024]; try { FileOutputStream fos = new FileOutputStream(zipFilePath); ZipOutputStream zos = new ZipOutputStream(fos); File folderToCompress = new File(sourceFolder); compressFolderToZipFile(folderToCompress, folderToCompress.getName(), zos, buffer); zos.close(); } catch (IOException e) { e.printStackTrace(); } } private static void compressFolderToZipFile(File folder, String parentFolder, ZipOutputStream zos, byte[] buffer) throws IOException { File[] files = folder.listFiles(); for (File file : files) { if (file.isDirectory()) { compressFolderToZipFile(file, parentFolder + "/" + file.getName(), zos, buffer); } else { ZipEntry zipEntry = new ZipEntry(parentFolder + "/" + file.getName()); zos.putNextEntry(zipEntry); FileInputStream fis = new FileInputStream(file); int len; while ((len = fis.read(buffer)) > 0) { zos.write(buffer, 0, len); } fis.close(); zos.closeEntry(); } } } }
三、使用第三方库进行文件压缩
除了Java自带的工具类外,还可以使用第三方库来实现更强大的文件压缩功能,常用的第三方库有ZIP4J和TrueVFS等,下面以ZIP4J为例介绍如何使用第三方库进行文件压缩。
1. 添加依赖
在项目的build.gradle
文件中添加ZIP4J的依赖:
dependencies { implementation 'net.lingala.zip4j:zip4j:2.9.1' }
2. 压缩文件或文件夹
使用ZIP4J进行文件或文件夹的压缩非常简单,以下是一个简单的示例:
import net.lingala.zip4j.ZipFile; import net.lingala.zip4j.model.ZipParameters; import net.lingala.zip4j.model.enums.CompressionLevel; import net.lingala.zip4j.model.enums.CompressionMethod; import net.lingala.zip4j.model.enums.EncryptionMethod; import java.io.File; public class Zip4jExample { public static void main(String[] args) { try { // 创建ZIP文件参数 ZipParameters parameters = new ZipParameters(); parameters.setCompressionMethod(CompressionMethod.DEFLATE); // 设置压缩方法为DEFLATE parameters.setCompressionLevel(CompressionLevel.NORMAL); // 设置压缩级别为正常 parameters.setEncryptFiles(true); // 启用加密 parameters.setEncryptionMethod(EncryptionMethod.ZIP_STANDARD); // 设置加密方法为标准ZIP加密 // 创建ZIP文件对象 ZipFile zipFile = new ZipFile("output.zip", "password".toCharArray()); // 设置密码保护 zipFile.addFolder(new File("path/to/your/folder"), parameters); // 添加文件夹到ZIP文件 zipFile.addFile(new File("path/to/your/file"), parameters); // 添加文件到ZIP文件 } catch (Exception e) { e.printStackTrace(); } } }
本文介绍了在Android平台上实现文件或文件夹压缩的两种主要方法:使用Java内置的工具类和使用第三方库ZIP4J,根据实际需求选择合适的方法,可以有效地实现文件的压缩和解压缩功能,希望本文对你有所帮助!
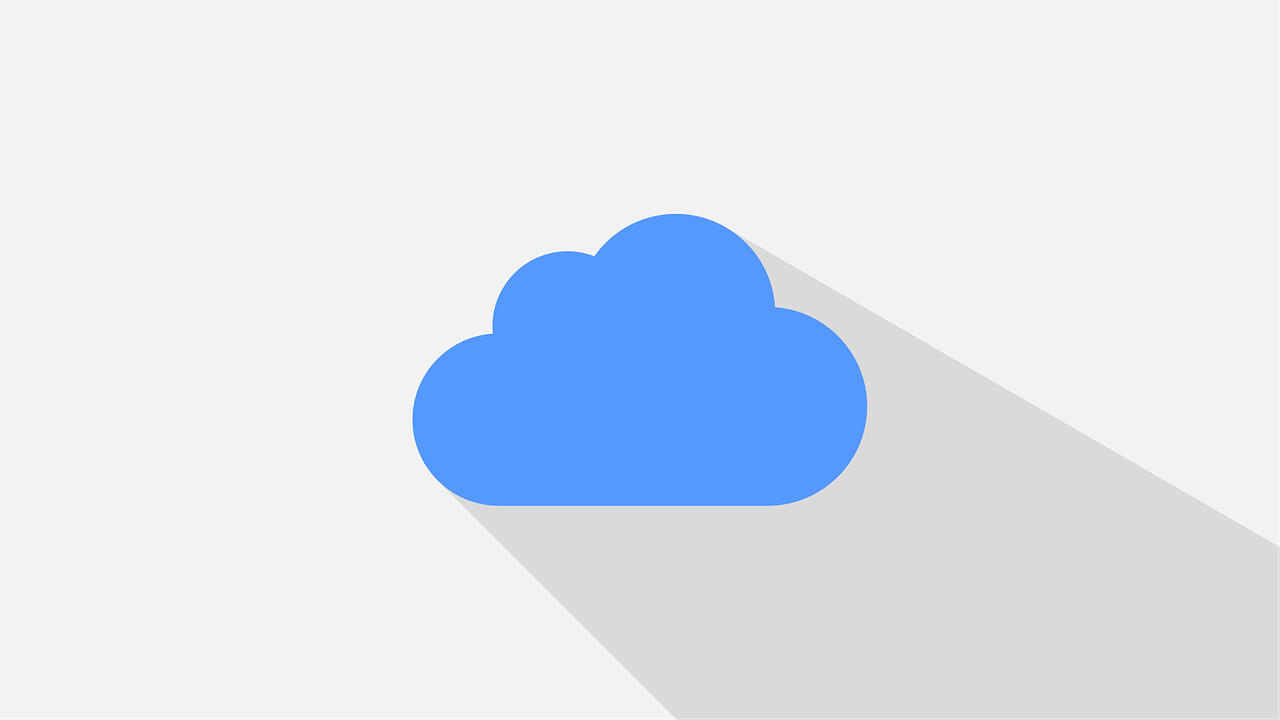
以上就是关于“Android实现文件或文件夹压缩”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1278324.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复