Android实现倒计时结束后跳转页面功能
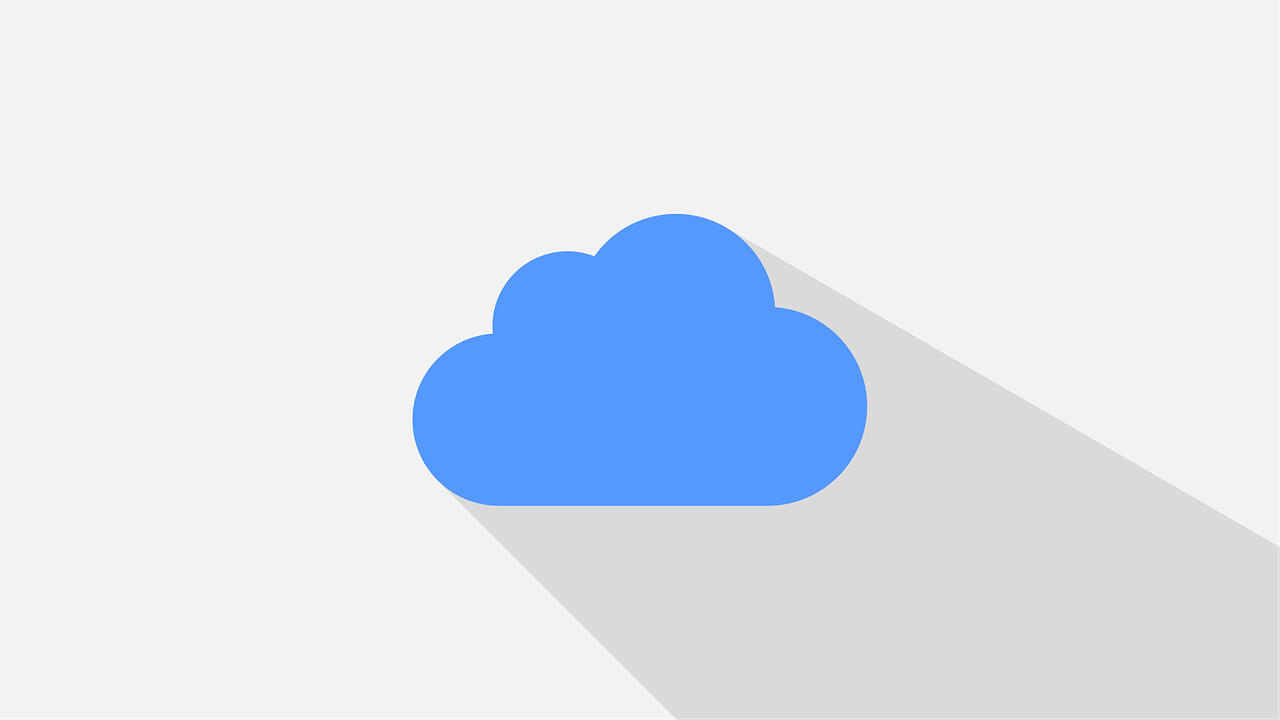
在Android应用开发中,倒计时功能是一个常见的需求,在考试应用、游戏或限时活动中,倒计时可以给用户带来紧张感和时间管理的体验,本文将介绍如何在Android中实现一个倒计时功能,并在倒计时结束后自动跳转到另一个页面。
一、创建项目
我们需要创建一个Android项目,打开Android Studio,选择“Start a new Android Studio project”,填写项目名称和保存位置,然后点击“Finish”。
二、设计界面
在res/layout目录下的activity_main.xml文件中,设计一个简单的界面,这个界面包含一个TextView用于显示倒计时时间,一个Button用于开始倒计时。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/tv_countdown" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="48sp" android:layout_centerInParent="true"/> <Button android:id="@+id/btn_start" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="开始倒计时" android:layout_below="@id/tv_countdown" android:layout_centerHorizontal="true" android:layout_marginTop="20dp"/> </RelativeLayout>
三、实现倒计时逻辑
在MainActivity.java文件中,实现倒计时的逻辑,当用户点击按钮时,开始倒计时,并在倒计时结束后跳转到另一个页面。
package com.example.countdown; import android.content.Intent; import android.os.Bundle; import android.os.Handler; import android.view.View; import android.widget.Button; import android.widget.TextView; import androidx.appcompat.app.AppCompatActivity; public class MainActivity extends AppCompatActivity { private static final long COUNTDOWN_TIME = 10000; // 倒计时时间(毫秒) private TextView tvCountdown; private Button btnStart; private Handler handler = new Handler(); @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); tvCountdown = findViewById(R.id.tv_countdown); btnStart = findViewById(R.id.btn_start); btnStart.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { startCountdown(); } }); } private void startCountdown() { long timeLeft = COUNTDOWN_TIME; tvCountdown.setText(formatTime(timeLeft)); handler.postDelayed(new Runnable() { @Override public void run() { if (timeLeft > 0) { timeLeft -= 1000; tvCountdown.setText(formatTime(timeLeft)); handler.postDelayed(this, 1000); } else { // 倒计时结束,跳转到另一个页面 Intent intent = new Intent(MainActivity.this, AnotherActivity.class); startActivity(intent); } } }, 1000); } private String formatTime(long millis) { long seconds = millis / 1000; long minutes = seconds / 60; seconds %= 60; long hours = minutes / 60; minutes %= 60; return String.format("%02d:%02d:%02d", hours, minutes, seconds); } }
在这个示例中,我们使用了一个Handler来每隔一秒更新一次TextView的文本,以显示剩余的倒计时时间,当倒计时结束时,我们使用Intent跳转到另一个页面。
四、创建目标页面
为了测试跳转功能,我们需要创建一个简单的目标页面,在com.example.countdown包下创建一个新的Activity,命名为AnotherActivity。
package com.example.countdown; import android.os.Bundle; import androidx.appcompat.app.AppCompatActivity; public class AnotherActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_another); } }
在res/layout目录下创建一个新的布局文件activity_another.xml:
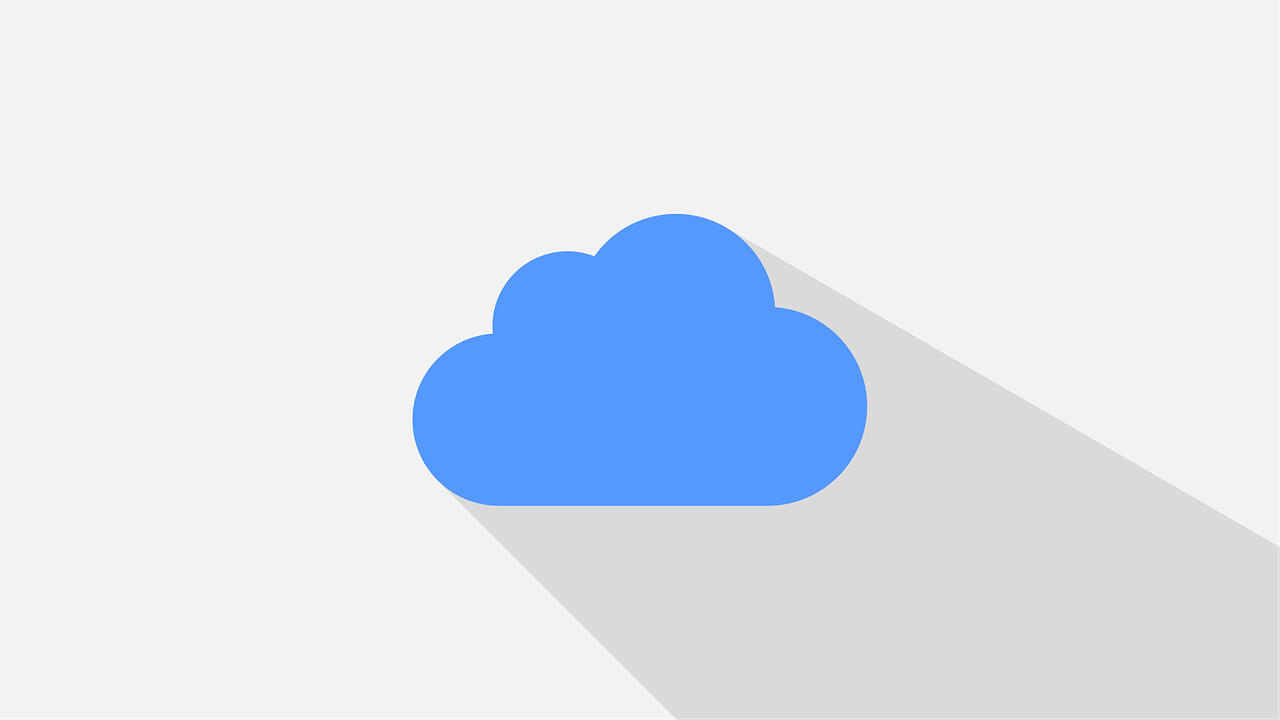
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="倒计时结束,跳转到这个页面!" android:textSize="24sp" android:layout_centerInParent="true"/> </RelativeLayout>
五、配置AndroidManifest.xml
我们需要在AndroidManifest.xml文件中注册AnotherActivity:
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.countdown"> <application ...> <activity android:name=".AnotherActivity"></activity> </application> </manifest>
至此,我们已经完成了一个简单的Android倒计时功能,并在倒计时结束后跳转到另一个页面,这个示例展示了如何使用Handler来实现定时任务,以及如何使用Intent进行页面跳转,你可以根据实际需求对这个示例进行扩展和优化。
小伙伴们,上文介绍了“Android实现倒计时结束后跳转页面功能”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1277867.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复