BottomSheetDialog
来实现从底部弹出的对话框。以下是一个简单的实例代码:,,“java,import android.os.Bundle;,import androidx.appcompat.app.AppCompatActivity;,import com.google.android.material.bottomsheet.BottomSheetDialog;,,public class MainActivity extends AppCompatActivity {, @Override, protected void onCreate(Bundle savedInstanceState) {, super.onCreate(savedInstanceState);, setContentView(R.layout.activity_main);,, // 创建BottomSheetDialog实例, BottomSheetDialog bottomSheetDialog = new BottomSheetDialog(this);, , // 设置自定义布局, bottomSheetDialog.setContentView(R.layout.bottom_sheet_dialog);, , // 显示对话框, bottomSheetDialog.show();, },},
`,,在这个示例中,我们首先创建一个
BottomSheetDialog实例,然后通过
setContentView方法设置自定义的布局文件,最后调用
show`方法来显示对话框。在Android开发中,从底部弹出的Dialog是一种常见的UI交互方式,这种Dialog通常用于显示操作菜单、选择项或者提供额外的信息,下面是一个详细的实例代码,展示如何实现一个从底部弹出的Dialog。
准备工作
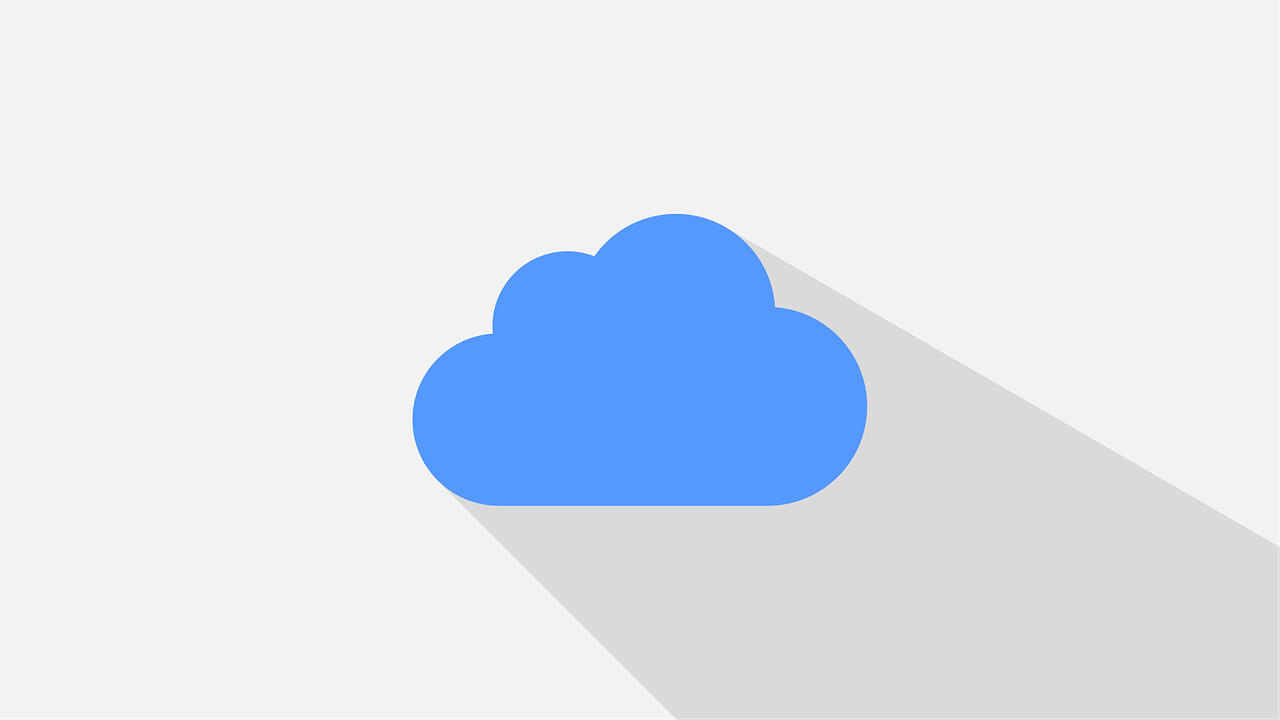
确保你的项目已经配置好基本的Android环境,并且你已经创建了一个Activity。
布局文件
创建一个布局文件dialog_bottom_sheet.xml
,这个文件将定义底部弹出的Dialog的布局。
<!-res/layout/dialog_bottom_sheet.xml --> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="16dp"> <TextView android:id="@+id/tvTitle" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Bottom Sheet Dialog" android:textSize="18sp" android:gravity="center" android:paddingBottom="16dp"/> <Button android:id="@+id/btnOption1" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Option 1"/> <Button android:id="@+id/btnOption2" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Option 2"/> <Button android:id="@+id/btnCancel" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Cancel"/> </LinearLayout>
创建Dialog类
在你的Activity中创建一个方法来显示底部弹出的Dialog。
// MainActivity.java import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.widget.Button; import androidx.annotation.Nullable; import androidx.appcompat.app.AppCompatActivity; import com.google.android.material.bottomsheet.BottomSheetDialog; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button btnShowDialog = findViewById(R.id.btnShowDialog); btnShowDialog.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { showBottomSheetDialog(); } }); } private void showBottomSheetDialog() { View view = LayoutInflater.from(this).inflate(R.layout.dialog_bottom_sheet, null); BottomSheetDialog bottomSheetDialog = new BottomSheetDialog(this); bottomSheetDialog.setContentView(view); Button btnOption1 = view.findViewById(R.id.btnOption1); Button btnOption2 = view.findViewById(R.id.btnOption2); Button btnCancel = view.findViewById(R.id.btnCancel); btnOption1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Handle option 1 click bottomSheetDialog.dismiss(); } }); btnOption2.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Handle option 2 click bottomSheetDialog.dismiss(); } }); btnCancel.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { bottomSheetDialog.dismiss(); } }); bottomSheetDialog.show(); } }
主布局文件
确保你的主布局文件中有一个按钮来触发显示底部弹出的Dialog。
<!-res/layout/activity_main.xml --> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/btnShowDialog" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Show Bottom Sheet Dialog" android:layout_centerInParent="true"/> </RelativeLayout>
依赖库
确保你在项目的build.gradle
文件中添加了Material Design组件库的依赖:
dependencies { implementation 'com.google.android.material:material:1.4.0' // 确保使用最新版本 }
运行应用
现在你可以运行你的应用,点击按钮后会显示一个从底部弹出的Dialog,包含三个选项和一个取消按钮,点击任意按钮都会关闭Dialog。
通过以上步骤,我们实现了一个简单的从底部弹出的Dialog,这个Dialog使用了Material Design组件库中的BottomSheetDialog
,使得实现更加简洁和美观,你可以根据需要进一步自定义Dialog的内容和样式。
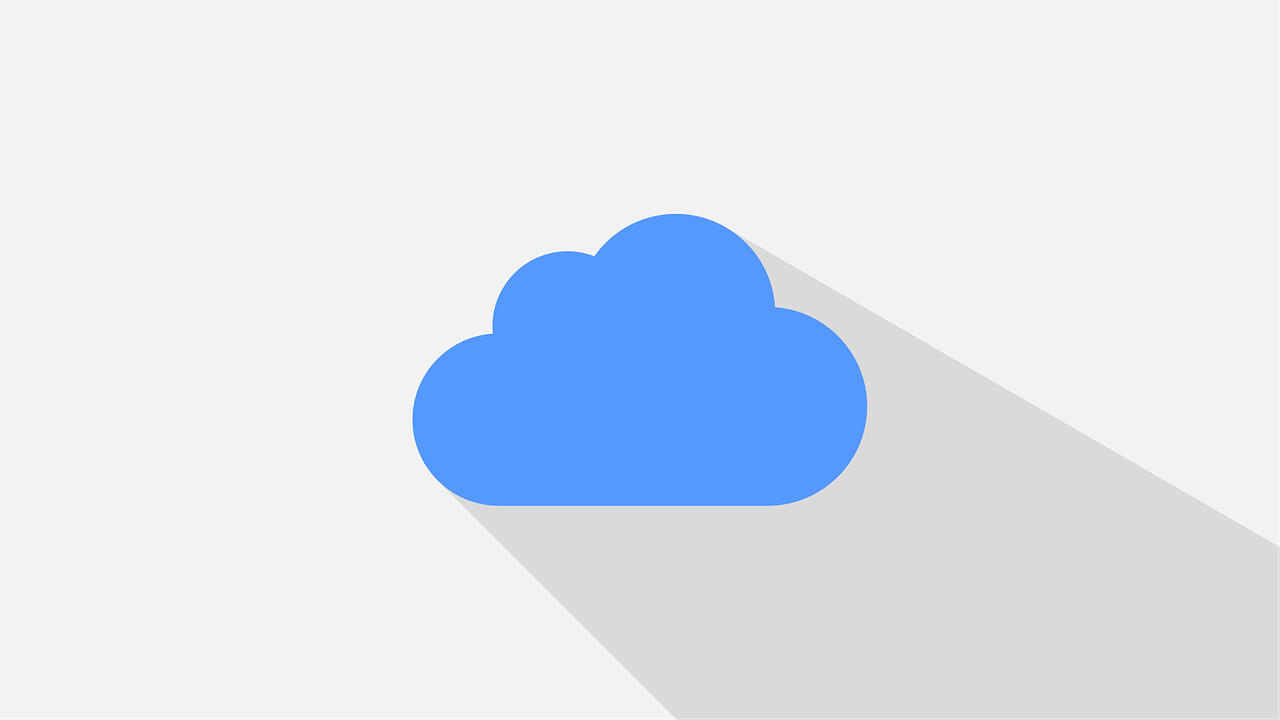
以上就是关于“Android实现从底部弹出的Dialog的实例代码”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1277459.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复