SpeechRecognizer
类来处理语音输入。以下是一个简单的示例代码:,,“java,import android.content.Intent;,import android.os.Bundle;,import android.speech.RecognitionListener;,import android.speech.RecognizerIntent;,import android.speech.SpeechRecognizer;,import androidx.appcompat.app.AppCompatActivity;,import java.util.ArrayList;,,public class VoiceActivity extends AppCompatActivity {, private SpeechRecognizer speechRecognizer;,, @Override, protected void onCreate(Bundle savedInstanceState) {, super.onCreate(savedInstanceState);, setContentView(R.layout.activity_voice);,, speechRecognizer = SpeechRecognizer.createSpeechRecognizer(this);, speechRecognizer.setRecognitionListener(new RecognitionListener() {, @Override, public void onReadyForSpeech(Bundle params) {},, @Override, public void onBeginningOfSpeech() {},, @Override, public void onRmsChanged(float rmsdB) {},, @Override, public void onBufferReceived(byte[] buffer) {},, @Override, public void onEndOfSpeech() {},, @Override, public void onError(int error) {},, @Override, public void onResults(Bundle results) {, ArrayList matches = results.getStringArrayList(SpeechRecognizer.RESULTS_RECOGNITION);, if (matches != null && !matches.isEmpty()) {, String text = matches.get(0);, // 处理识别到的文本, }, },, @Override, public void onPartialResults(Bundle partialResults) {},, @Override, public void onEvent(int eventType, Bundle params) {}, });,, Intent intent = new Intent(RecognizerIntent.ACTION_RECOGNIZE_SPEECH);, intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE_MODEL, RecognizerIntent.LANGUAGE_MODEL_FREE_FORM);, intent.putExtra(RecognizerIntent.EXTRA_MAX_RESULTS, 1);, speechRecognizer.startListening(intent);, },, @Override, protected void onDestroy() {, super.onDestroy();, if (speechRecognizer != null) {, speechRecognizer.destroy();, }, },},
“,,这段代码创建了一个基本的语音识别界面,当用户说话时,会将识别到的文本进行处理。在Android应用中实现客户端语音动弹界面,可以通过使用Android的SpeechRecognizer类来实现语音识别功能,以下是一个简单的实例代码,展示如何创建一个基本的语音输入界面,并处理用户的语音输入。
添加权限和依赖
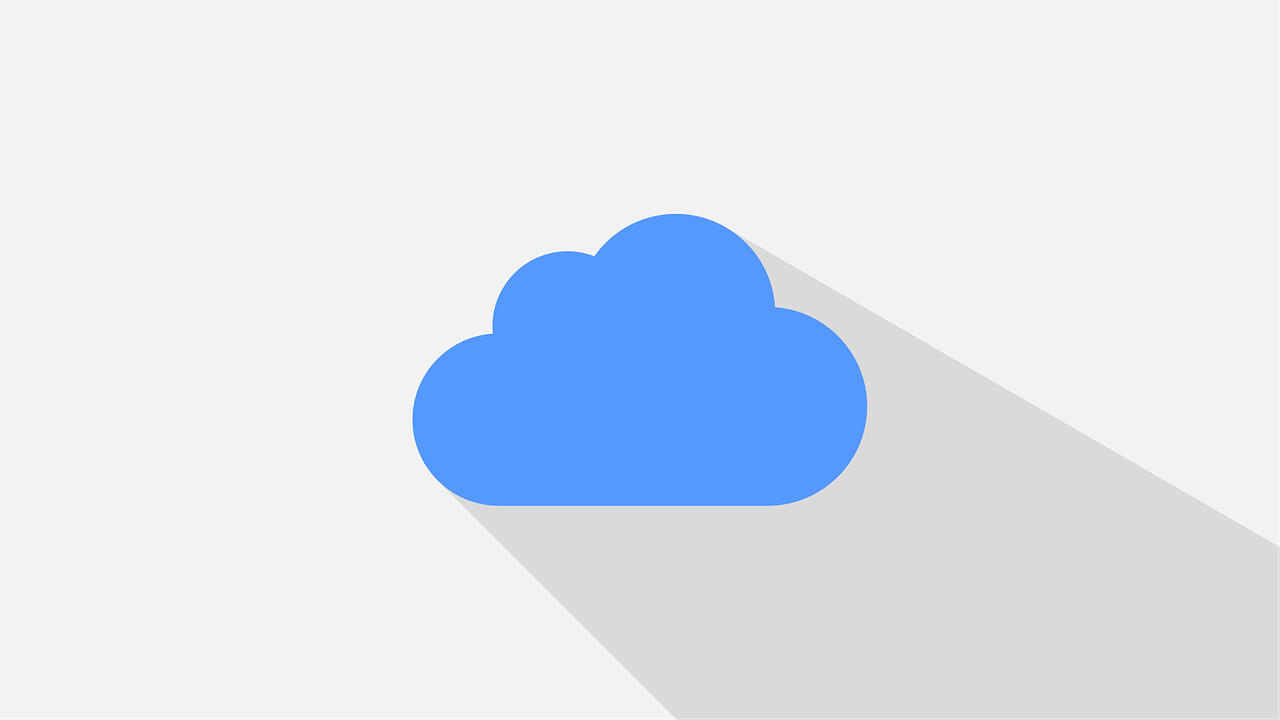
需要在AndroidManifest.xml
文件中添加必要的权限:
<uses-permission android:name="android.permission.RECORD_AUDIO"/> <uses-permission android:name="android.permission.INTERNET"/>
确保你的项目已经包含了Google Play服务库,因为SpeechRecognizer依赖于它,在你的build.gradle
文件中添加以下依赖:
implementation 'com.google.android.gms:play-services-base:17.6.0' implementation 'com.google.android.gms:play-services-location:18.0.0'
创建布局文件
在res/layout
目录下创建一个新的XML布局文件,例如activity_main.xml
,用于定义用户界面:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/btn_start_listening" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Start Listening" android:layout_centerInParent="true"/> <TextView android:id="@+id/tv_result" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Result will be shown here" android:layout_below="@id/btn_start_listening" android:layout_centerHorizontal="true" android:layout_marginTop="20dp"/> </RelativeLayout>
编写MainActivity代码
在MainActivity.java
或MainActivity.kt
中编写代码来处理语音识别逻辑:
package com.example.voicerecognition; import androidx.annotation.Nullable; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.speech.RecognizerIntent; import android.speech.SpeechRecognizer; import android.view.View; import android.widget.Button; import android.widget.TextView; import java.util.ArrayList; import java.util.Locale; public class MainActivity extends AppCompatActivity { private static final int REQUEST_CODE_SPEECH_INPUT = 1000; private Button btnStartListening; private TextView tvResult; private SpeechRecognizer speechRecognizer; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btnStartListening = findViewById(R.id.btn_start_listening); tvResult = findViewById(R.id.tv_result); speechRecognizer = SpeechRecognizer.createSpeechRecognizer(this); btnStartListening.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { startListening(); } }); } private void startListening() { Intent intent = new Intent(RecognizerIntent.ACTION_RECOGNIZE_SPEECH); intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE_MODEL, RecognizerIntent.LANGUAGE_MODEL_FREE_FORM); intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE, Locale.getDefault()); intent.putExtra(RecognizerIntent.EXTRA_PROMPT, "Speak something..."); try { speechRecognizer.startListening(intent); } catch (Exception e) { e.printStackTrace(); } } @Override protected void onDestroy() { super.onDestroy(); if (speechRecognizer != null) { speechRecognizer.destroy(); } } }
处理语音识别结果
为了处理语音识别的结果,需要重写onActivityResult
方法:
@Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == REQUEST_CODE_SPEECH_INPUT && resultCode == RESULT_OK && data != null) { ArrayList<String> result = data.getStringArrayListExtra(RecognizerIntent.EXTRA_RESULTS); if (result != null && !result.isEmpty()) { tvResult.setText(result.get(0)); } else { tvResult.setText("No voice input detected"); } } }
运行应用
现在你可以运行你的应用,点击“Start Listening”按钮,然后说出一些话,应用会显示你说的话。
是一个简单的Android客户端语音动弹界面的实现示例,通过使用Android的SpeechRecognizer类,我们可以轻松地将语音识别功能集成到我们的应用中,这个示例展示了如何启动语音识别、处理结果以及更新UI,根据实际需求,你可以进一步扩展和优化这个基础实现。
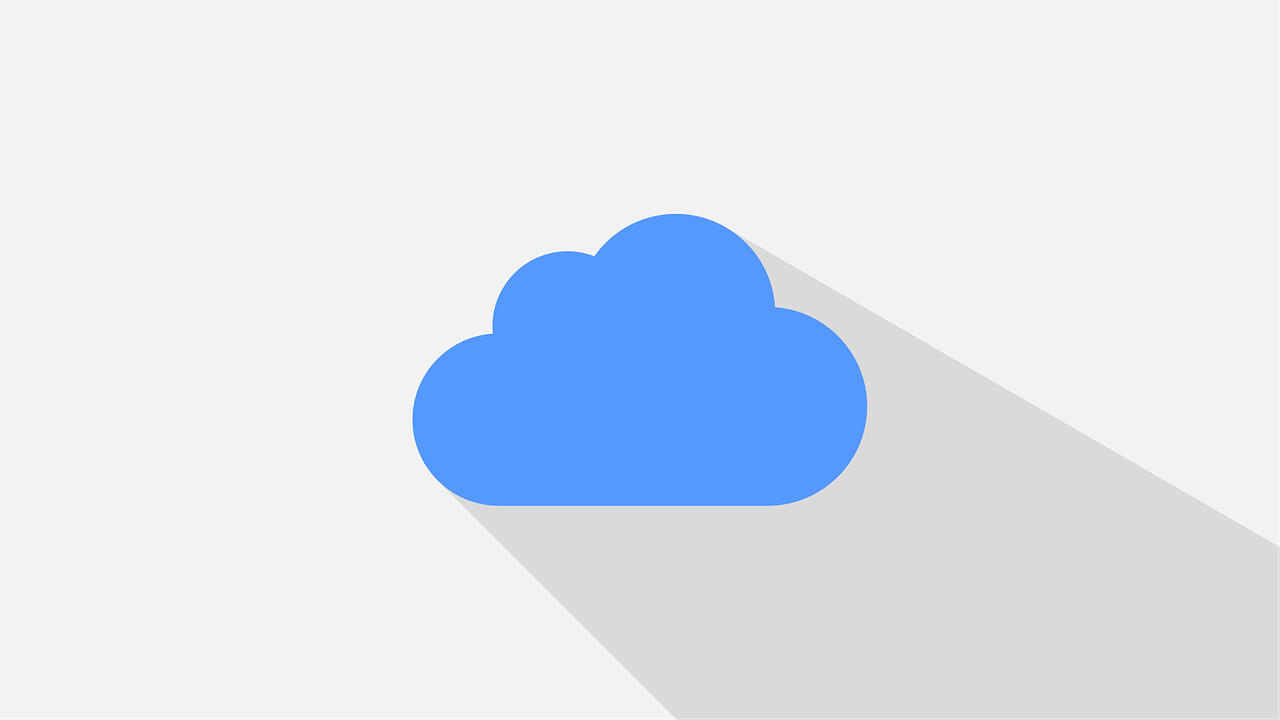
到此,以上就是小编对于“Android实现客户端语音动弹界面实例代码”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1277380.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复