MapReduce 中文分词详解
MapReduce是一种用于处理和生成大规模数据集的编程模型,特别适用于自然语言处理(NLP)任务中的文本分析,本文将详细介绍如何在Hadoop平台上使用MapReduce进行中文分词,包括环境配置、代码实现以及自定义词典的使用。
一、准备工作
1、Hadoop集群搭建:首先需要搭建一个Hadoop集群,可以使用单节点模式进行测试开发,具体步骤可以参考Hadoop官方文档或相关教程。
2、开发环境配置:推荐使用IntelliJ IDEA作为IDE,并安装Maven以管理项目依赖。
二、添加HanLP依赖
HanLP是一个高效的Java NLP工具包,支持多种分词算法和自定义词典功能,我们可以通过Maven来引入HanLP的依赖,在项目的pom.xml
文件中添加以下内容:
<dependency> <groupId>com.hankcs</groupId> <artifactId>hanlp</artifactId> <version>portable1.7.8</version> </dependency>
这样,就可以轻松地在项目中使用HanLP提供的分词功能了。
三、编写MapReduce程序
1. Mapper类
Mapper的主要任务是对输入的每一行文本进行分词,并将每个词及其出现次数发送给Reducer,以下是一个简单的示例代码:
import com.hankcs.hanlp.HanLP; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Mapper; import java.io.IOException; public class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> { private final static IntWritable one = new IntWritable(1); private Text word = new Text(); @Override protected void map(Object key, Text value, Context context) throws IOException, InterruptedException { // 使用HanLP进行分词 String[] words = HanLP.segment(value.toString()).toStringArray(); for (String w : words) { word.set(w); context.write(word, one); } } }
2. Reducer类
Reducer的任务是接收Mapper输出的所有词汇及其出现次数,然后汇总每个词汇的总出现次数,示例代码如下:
import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Reducer; import java.io.IOException; public class WordCountReducer extends Reducer<Text, IntWritable, Text, IntWritable> { @Override protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } context.write(key, new IntWritable(sum)); } }
3. Driver类
Driver类负责设置作业配置、指定输入输出路径以及运行作业,示例代码如下:
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat; public class WordCountDriver { public static void main(String[] args) throws Exception { if (args.length != 2) { System.err.println("Usage: WordCount <input path> <output path>"); System.exit(1); } Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "word count"); job.setJarByClass(WordCountDriver.class); job.setMapperClass(TokenizerMapper.class); job.setCombinerClass(WordCountReducer.class); job.setReducerClass(WordCountReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
四、运行MapReduce作业
编译并打包上述代码后,将其提交到Hadoop集群执行,命令如下:
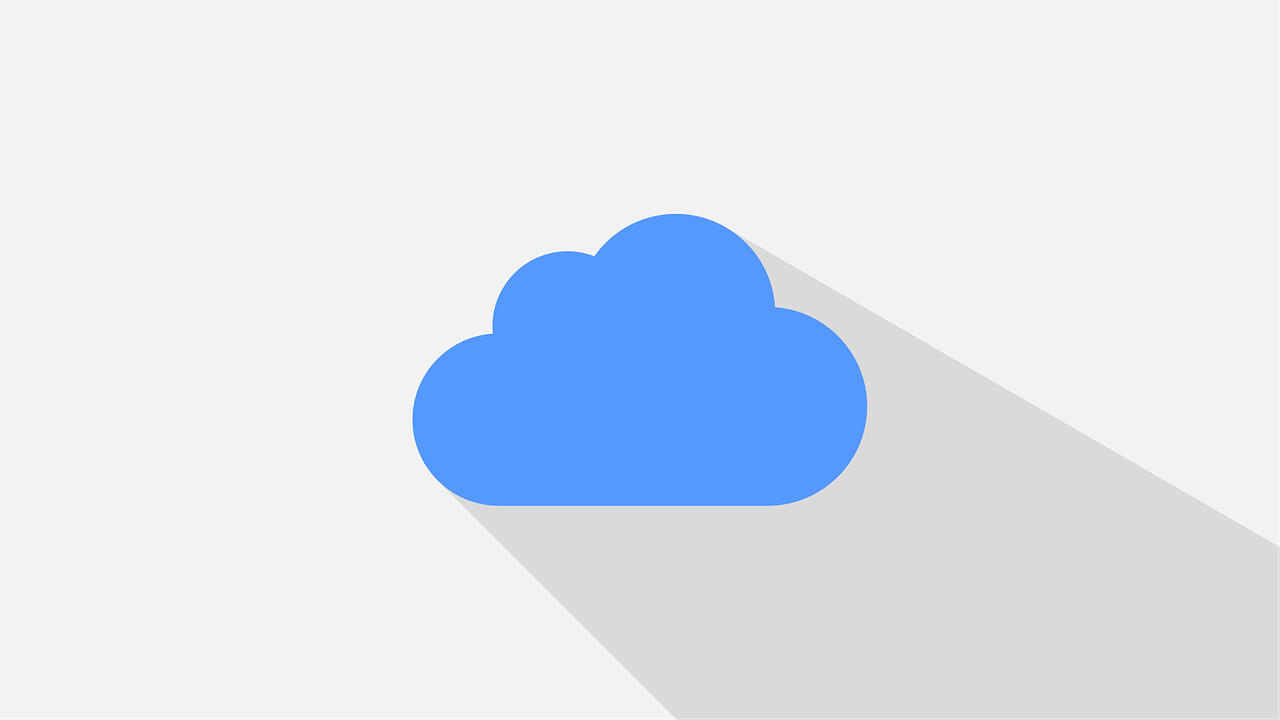
hadoop jar yourjarfile.jar input_directory output_directory
yourjarfile.jar
是你的可执行JAR文件名,input_directory
是输入数据所在的HDFS目录,output_directory
是结果存储的HDFS目录。
五、自定义词典文件的使用
有时我们需要根据业务需求添加特定的词汇到分词器中,HanLP支持通过配置文件或API动态添加自定义词典,以下是两种常见的方法:
1、通过配置文件添加自定义词典:在src/main/resources
目录下创建一个名为hanlp.properties
的文件,内容如下:
DataHome=datadictionarycustom
然后在data/dictionary/custom
目录下创建一个新的词典文件(如myDictionary.txt
),每行一个词,在代码中加载这个配置文件即可。
Properties properties = new Properties(); properties.load(new FileInputStream("src/main/resources/hanlp.properties")); CustomDictionary.add(new CustomDictionary()); // 根据实际需求调用相应的方法加载自定义词汇
2、通过API动态添加自定义词典:可以直接在代码中使用CustomDictionary
类的方法动态添加词汇:
CustomDictionary.add("新词1"); CustomDictionary.add("新词2");
这种方法更加灵活,但需要注意线程安全问题。
MapReduce结合HanLP可以实现高效的中文分词处理,通过合理的配置和编码,可以轻松应对大规模文本数据的分词需求,希望本文能够帮助读者更好地理解和应用这项技术。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1235942.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复