javascript, fetch('/api/comments/count'), .then(response => response.json()), .then(data => {, // 处理返回的数据, }), .catch(error => {, console.error('Error:', error);, });,
`,,2. **处理响应**:,
`javascript, function updateCommentCount(count) {, document.getElementById('commentcount').innerText = count;, },
`,,3. **绑定到页面元素**:,
`html,0,
`,,4. **完整示例**:,
`javascript, fetch('/api/comments/count'), .then(response => response.json()), .then(data => {, updateCommentCount(data.count);, }), .catch(error => {, console.error('Error:', error);, });,, function updateCommentCount(count) {, document.getElementById('commentcount').innerText = count;, },
“,,这种方式可以动态地更新评论总数,而无需重新加载整个页面。在现代Web开发中,JavaScript(JS)已经成为了不可或缺的一部分,通过JavaScript,我们可以实现各种动态效果和功能,包括调用评论总数这样的数据,本文将详细介绍如何使用JavaScript来获取评论总数,并提供一些实用的技巧和示例代码。
理解评论总数的意义
在大多数网站和应用中,评论总数是一个非常重要的指标,它不仅可以帮助用户了解某个内容的受欢迎程度,还可以为网站管理员提供有价值的数据,以便进行内容优化和用户体验改进,准确地获取和显示评论总数是非常重要的。
使用AJAX获取评论总数
AJAX(Asynchronous JavaScript and XML)是一种在无需重新加载整个网页的情况下,能够更新部分网页的技术,通过AJAX,我们可以从服务器请求数据并更新网页,而不影响用户体验,以下是一个简单的例子,展示如何使用AJAX获取评论总数:
1、HTML部分:
<div id="commentscount">正在加载评论数...</div>
2、JavaScript部分:
function getCommentsCount() { var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { document.getElementById("commentscount").innerHTML = this.responseText; } }; xhr.open("GET", "/api/comments/count", true); xhr.send(); } getCommentsCount();
3、服务器端部分(以Node.js为例):
const express = require('express'); const app = express(); app.get('/api/comments/count', (req, res) => { // 假设我们有一个函数getCommentCount()可以获取评论总数 const count = getCommentCount(); res.send(String(count)); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
使用Fetch API获取评论总数
Fetch API提供了一个更加现代化和灵活的方式来进行网络请求,以下是使用Fetch API的示例:
1、HTML部分:
<div id="commentscount">正在加载评论数...</div>
2、JavaScript部分:
async function getCommentsCount() { try { const response = await fetch('/api/comments/count'); const count = await response.text(); document.getElementById("commentscount").innerHTML = count; } catch (error) { console.error('Error:', error); } } getCommentsCount();
使用jQuery获取评论总数
如果你已经在项目中使用了jQuery,那么可以使用它的$.ajax
方法来简化AJAX请求:
1、HTML部分:
<div id="commentscount">正在加载评论数...</div>
2、JavaScript部分:
function getCommentsCount() { $.ajax({ url: '/api/comments/count', method: 'GET', success: function(data) { $('#commentscount').html(data); }, error: function(error) { console.error('Error:', error); } }); } getCommentsCount();
使用React获取评论总数
在React应用中,我们可以结合组件状态来动态显示评论总数:
1、组件定义:
import React, { useEffect, useState } from 'react'; import axios from 'axios'; function CommentsCount() { const [count, setCount] = useState(0); useEffect(() => { async function fetchCommentsCount() { try { const response = await axios.get('/api/comments/count'); setCount(response.data); } catch (error) { console.error('Error:', error); } } fetchCommentsCount(); }, []); return <div>评论总数: {count}</div>; } export default CommentsCount;
常见问题解答(FAQs)
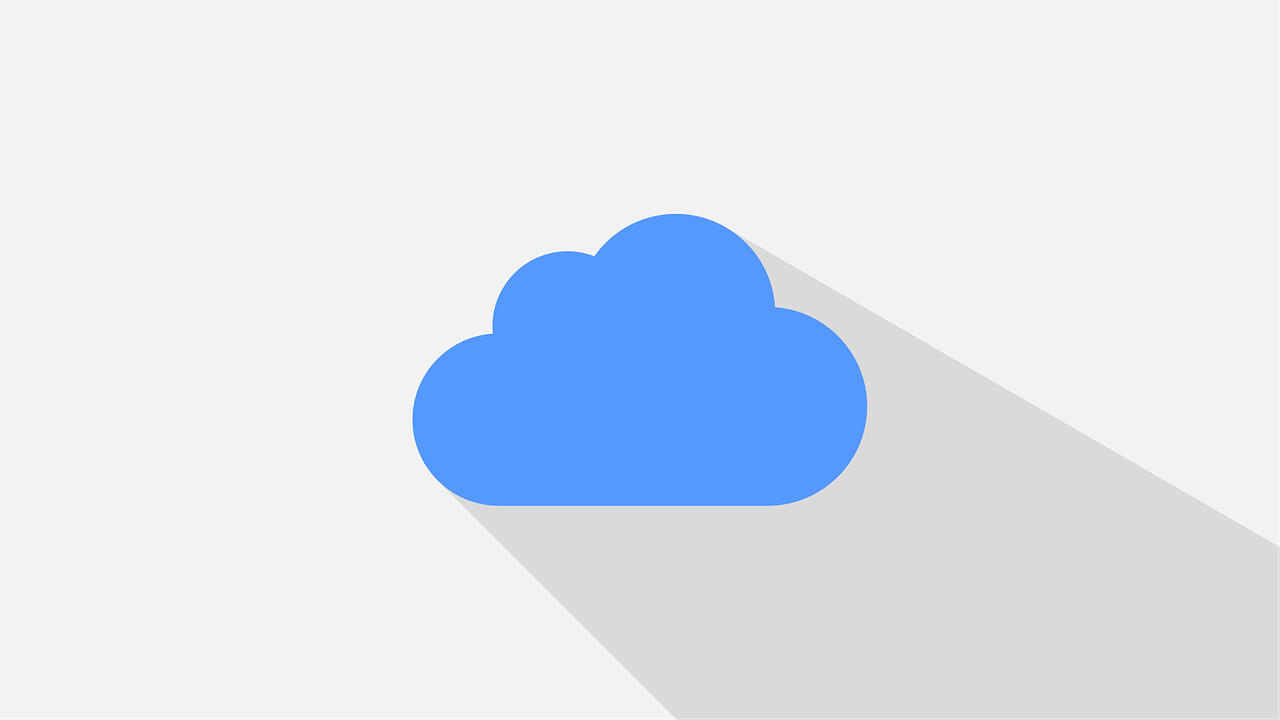
问题1:如何应对跨域请求?
答:如果前端和后端不在同一个域名下,可能会遇到跨域请求的问题,可以通过以下几种方式解决:
1、CORS(CrossOrigin Resource Sharing):在服务器端设置响应头,允许特定的域名访问资源,在Node.js中:
app.use((req, res, next) => { res.header("AccessControlAllowOrigin", "http://yourfrontenddomain.com"); res.header("AccessControlAllowHeaders", "Origin, XRequestedWith, ContentType, Accept"); next(); });
2、JSONP(JSON with Padding):只支持GET请求,且已经逐渐被CORS取代。
3、代理服务器:在开发环境中,可以使用代理服务器来解决跨域问题,在React应用中配置proxy
字段:
{ "proxy": "http://yourbackenddomain.com" }
问题2:如何提高数据加载性能?
答:提高数据加载性能可以从以下几个方面入手:
1、缓存:在客户端或服务器端对数据进行缓存,减少重复请求。
2、数据压缩:对传输的数据进行压缩,减少传输时间和带宽消耗。
3、懒加载:只有在需要显示数据时才进行请求,避免不必要的数据加载。
4、使用CDN分发网络(CDN)加速静态资源的加载速度。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1227042.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复