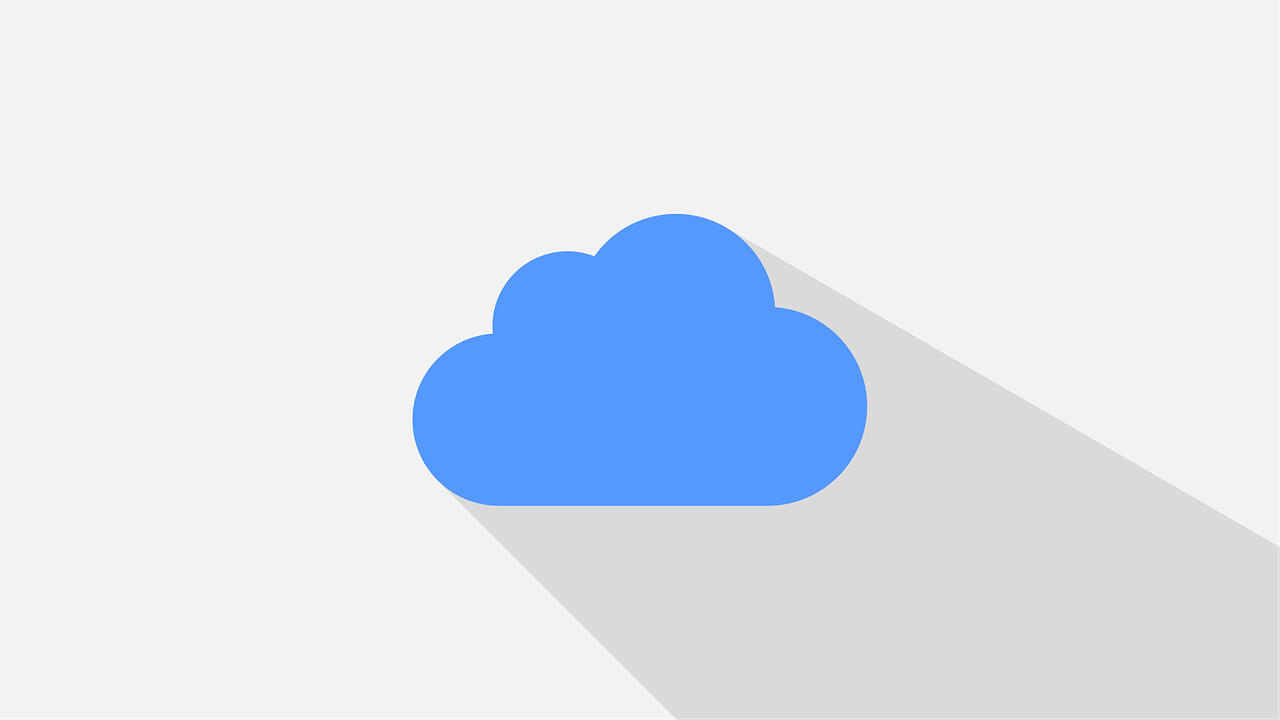
python,# 导入所需库,from mrjob.job import MRJob,,# 定义 Map 函数,def mapper(self, _, line):, for word in line.split():, yield (word, 1),,# 定义 Reduce 函数,def reducer(self, key, values):, yield (key, sum(values)),,# 创建 MapReduce 任务类,if __name__ == '__main__':, MRJobs.run(),
`,,上述代码使用 Python 编写,使用了
mrjob 库来简化 MapReduce 的实现。代码中的
mapper 函数将输入文本按空格分割成单词,并为每个单词生成一个键值对,其中键是单词本身,值是 1。
reducer 函数接收相同键(单词)的所有值,并计算它们的和。通过调用
MRJobs.run()` 运行 MapReduce 任务。,,这只是一个示例,实际的 MapReduce 任务可能需要根据具体需求进行适当的修改和扩展。MapReduce是一种编程模型,用于处理和生成大数据集,它由两个阶段组成:Map阶段和Reduce阶段,以下是一个简单的MapReduce代码示例,用于计算文本中单词的出现次数。
Map阶段
import sys from collections import defaultdict def map_function(line): words = line.split() word_count = defaultdict(int) for word in words: word_count[word] += 1 return word_count if __name__ == "__main__": for line in sys.stdin: map_result = map_function(line) for word, count in map_result.items(): print(f"{word}t{count}")
Reduce阶段
import sys from collections import defaultdict def reduce_function(word, counts): total_count = sum(counts) return (word, total_count) if __name__ == "__main__": current_word = None current_counts = [] for line in sys.stdin: word, count = line.strip().split('t') count = int(count) if current_word == word: current_counts.append(count) else: if current_word: print(reduce_function(current_word, current_counts)) current_word = word current_counts = [count] if current_word: print(reduce_function(current_word, current_counts))
这个示例中,我们首先定义了一个map_function
,它接收一行文本作为输入,将文本分割成单词,并计算每个单词的出现次数,我们在主程序中读取标准输入的每一行,并对每一行调用map_function
,我们将结果输出为键值对的形式,其中键是单词,值是该单词的出现次数。
在Reduce阶段,我们定义了一个reduce_function
,它接收一个单词和一个计数列表作为输入,并返回该单词的总出现次数,在主程序中,我们读取标准输入的每一行,并将每行的单词和计数添加到当前单词的计数列表中,当我们遇到一个新的单词时,我们调用reduce_function
来计算当前单词的总出现次数,并输出结果,我们需要确保处理完最后一个单词及其计数。
下面是一个简单的MapReduce代码示例,用于处理一个文本文件,统计每个单词的出现次数,表格将展示Map和Reduce阶段的伪代码。
| 阶段 | 伪代码 |
| | |
|Map阶段 |
def map_function(line): # 将行分割为单词 words = line.split() # 对每个单词进行映射,输出单词和1 for word in words: emit(word, 1)
|Shuffle阶段 |
MapReduce框架自动处理,将相同key的value进行分组
|Reduce阶段 |
def reduce_function(word, counts): # 将所有count相加 total_count = sum(counts) # 输出单词和总计数 emit(word, total_count)
在这个例子中,Map函数接收一行文本,将其分割成单词,并为每个单词发出一个键值对,其中键是单词,值是1,Shuffle阶段由MapReduce框架自动处理,将具有相同键的值(即相同的单词)分组到一起,Reduce函数接收一个单词及其所有计数,计算总计数,并输出单词及其总出现次数。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1221508.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复