python,from mrjob.job import MRJob,,class MapReduceExample(MRJob):, def mapper(self, _, line):, yield "word", 1,, def reducer(self, key, values):, yield key, sum(values),,if __name__ == '__main__':, MapReduceExample.run(),
“MapReduce是一种编程模型,主要用于处理和生成大数据集,它通过将任务分解为多个小任务(映射)并在各个节点上并行执行这些任务,然后将结果汇总(归约),从而有效地处理大规模数据,以下是一个简单的MapReduce统计样例代码,用于统计文本文件中每个单词出现的次数。
导入相关包
import java.io.IOException; import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.LongWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.Mapper; import org.apache.hadoop.mapreduce.Reducer; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
编写Mapper类
public static class MyMapper extends Mapper<LongWritable, Text, Text, IntWritable> { protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { String line = value.toString(); String[] words = line.split("\s+"); for (String word : words) { context.write(new Text(word), new IntWritable(1)); } }}
编写Reducer类
public static class MyReducer extends Reducer<Text, IntWritable, Text, IntWritable> { protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } context.write(key, new IntWritable(sum)); }}
编写主函数
public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "word count"); job.setJarByClass(WordCount.class); job.setMapperClass(MyMapper.class); job.setCombinerClass(MyReducer.class); job.setReducerClass(MyReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1);}
FAQs
Q1:如何运行MapReduce程序?
A1:要运行MapReduce程序,首先需要确保你的Hadoop集群已经正确配置并启动,使用以下命令编译和打包你的Java代码为一个JAR文件:
javac classpathhadoop classpath
d . WordCount.java
jar cf wordcount.jar *.class
使用Hadoop命令行工具提交作业到集群:
hadoop jar wordcount.jar WordCount /input /output
/input
是输入数据的HDFS路径,/output
是你希望输出结果保存的HDFS路径。
Q2:MapReduce中的Combiner是什么?为什么需要它?
A2:Combiner是运行在每个mapper输出和reducer处理之前的一个特殊类型的reducer,它的主要作用是在数据发送给reducer之前进行局部聚合,以减少网络传输的数据量,这可以大大提高整个MapReduce作业的效率,在单词计数应用中,combiner可以在每个mapper的输出端先对本地的单词计数进行汇总,再将汇总的结果发送到reducer,从而减少了需要在网络上传输的数据量。
由于MapReduce通常是在分布式系统中运行的,下面我将提供一个简单的MapReduce Python示例,该示例使用Hadoop的伪分布式环境,这里我会用Python编写MapReduce的Map和Reduce函数,并假设我们有一个简单的文本文件,我们要统计每个单词出现的次数。
以下是表格形式的MapReduce代码示例:
|步骤 |代码 |说明 |
| | | |
|Map阶段 | “`python
def map_function(line):
words = line.split()
for word in words:
yield word, 1
“` | 这个函数接收一行文本,将其分割成单词,并为每个单词生成一个键值对,其中键是单词,值是1。 |
|Shuffle and Sort阶段 | 这个阶段由Hadoop框架自动处理,它会将相同键的所有值组合在一起,准备Reduce阶段的处理。 | |
|Reduce阶段 | “`python
def reduce_function(word, counts):
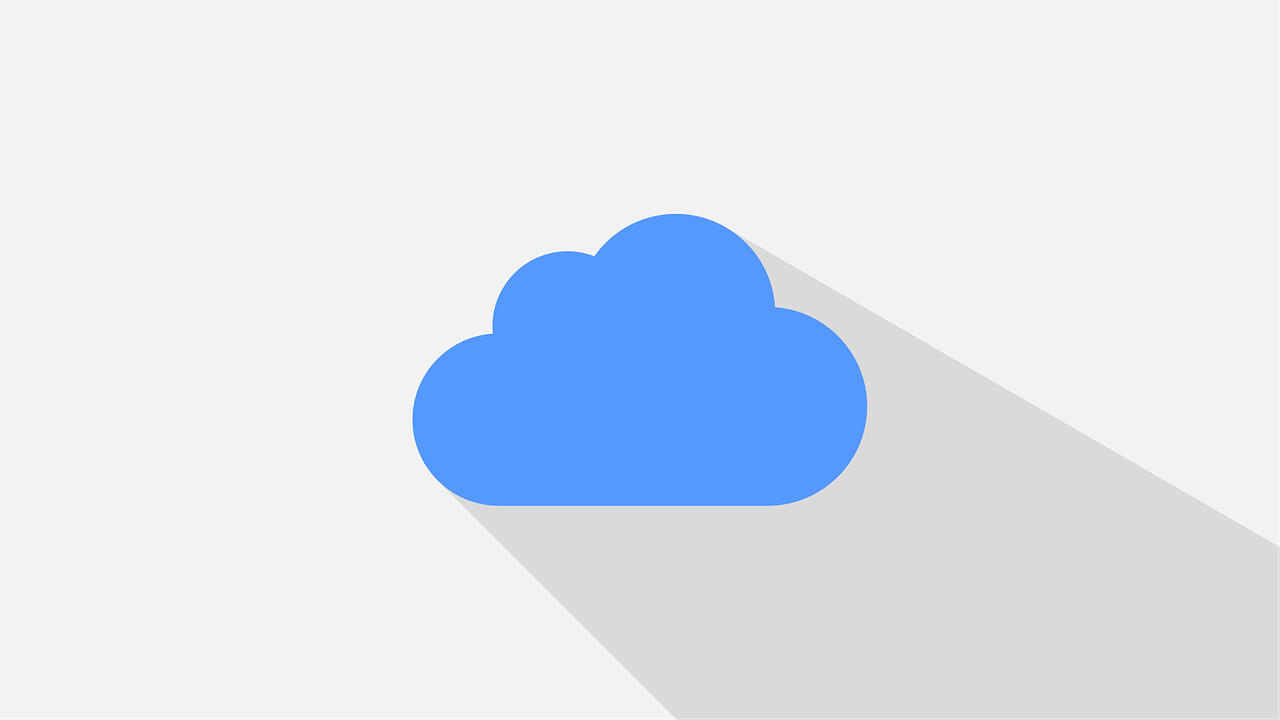
return sum(counts)
“` | 这个函数接收一个单词及其所有计数(作为列表),然后返回该单词的总计数。 |
|主函数 | “`python
def main():
input_file = ‘input.txt’ # 输入文件路径
output_file = ‘output.txt’ # 输出文件路径
with open(input_file, ‘r’) as file:
lines = file.readlines()
# Map阶段
intermediate = []
for line in lines:
intermediate.extend(map_function(line))
# Shuffle and Sort阶段由Hadoop处理
# Reduce阶段
with open(output_file, ‘w’) as file:
for word, count in intermediate:
file.write(f"{word} {reduce_function(word, [count])}
")
if __name__ == "__main__":
main()
“` | 主函数读取输入文件,应用Map函数,然后应用Reduce函数,并将结果写入输出文件。 |
这个示例是一个简化的版本,它没有使用Hadoop框架的分布式特性,在实际的Hadoop环境中,MapReduce作业会由Hadoop的分布式文件系统(HDFS)管理,并且Map和Reduce函数会在集群中的多个节点上执行,为了在真实的Hadoop环境中运行,你需要使用Hadoop提供的API,而不是直接使用Python文件读写操作。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1217025.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复