#include
,然后定义一个线程函数,该函数将在新线程中执行。使用pthread_create()
函数创建新线程,并传递线程函数和参数。使用pthread_join()
函数等待线程结束。以下是一个简单的示例:,,“c,#include,#include,,void *thread_function(void *arg) {, printf("This is a new thread.,");, return NULL;,},,int main() {, pthread_t thread_id;, int result;,, result = pthread_create(&thread_id, NULL, thread_function, NULL);, if (result != 0) {, printf("Error: unable to create thread, %d,", result);, return 1;, },, pthread_join(thread_id, NULL);, return 0;,},
“创建线程
在Linux系统中,线程的创建主要通过pthread库中的函数来实现,以下是创建线程的基本步骤和相关函数:
1、包含头文件:
“`c
#include <pthread.h>
“`
2、定义线程函数:
每个线程需要执行的任务都在一个独立的函数中定义,该函数被称为线程函数,线程函数必须满足以下原型:
“`c
void* thread_function(void* arg);
“`
3、创建线程:
使用pthread_create
函数来创建一个新的线程,该函数的原型如下:
“`c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
“`
thread
:指向线程标识符的指针。
attr
:用于设置线程属性,通常设置为NULL表示使用默认属性。
start_routine
:线程函数的起始地址。
arg
:传递给线程函数的参数。
4、示例代码:
“`c
#include <stdio.h>
#include <pthread.h>
void* thread_function(void* arg) {
int thread_id = *(int*)arg;
printf("Thread %d is running.
", thread_id);
return NULL;
}
int main() {
pthread_t thread;
int thread_id = 1;
int ret = pthread_create(&thread, NULL, thread_function, &thread_id);
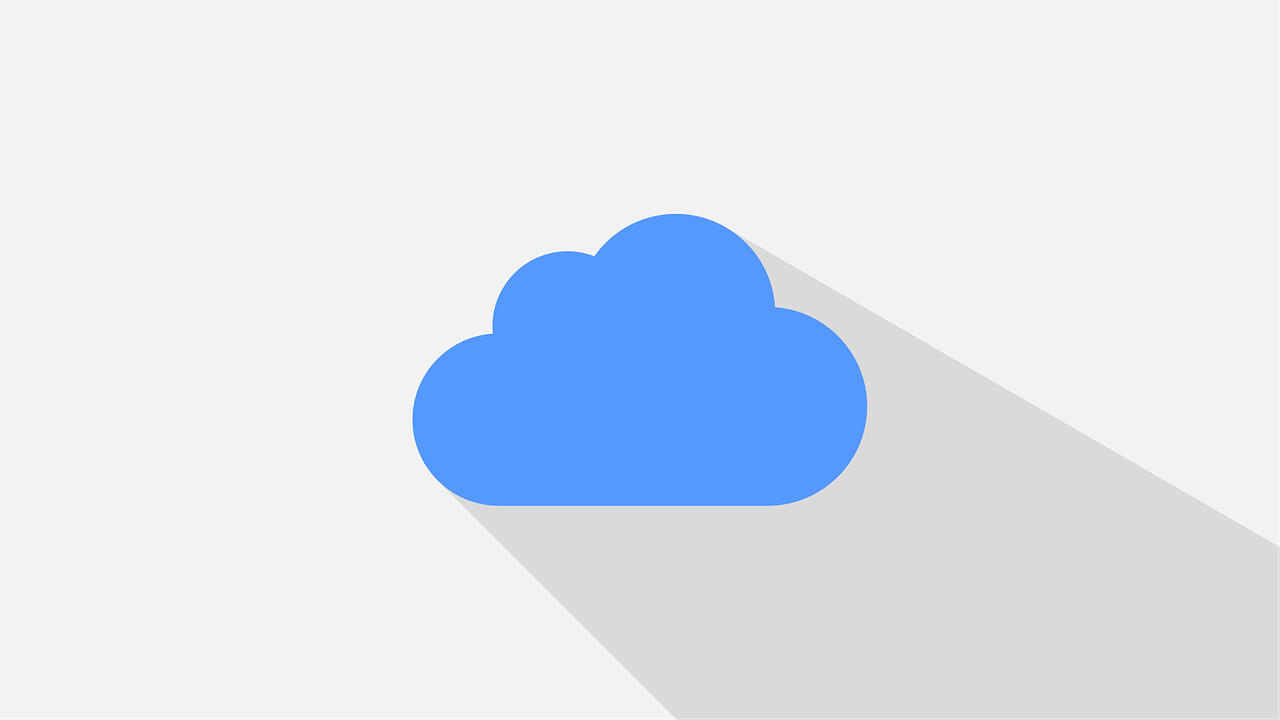
if (ret != 0) {
perror("pthread_create");
return 1;
}
pthread_join(thread, NULL);
return 0;
}
“`
线程同步
在多线程编程中,线程同步是一个关键问题,常用的线程同步机制包括互斥锁(Mutex)、条件变量(Condition Variable)和信号量(Semaphore)。
1、互斥锁:
互斥锁用于保护临界区,确保同一时间只有一个线程能够访问共享资源,常用的函数有:
pthread_mutex_init
:初始化互斥锁。
pthread_mutex_lock
:上锁。
pthread_mutex_unlock
:解锁。
pthread_mutex_destroy
:销毁互斥锁。
2、条件变量:
条件变量用于线程间的等待和通知,常与互斥锁一起使用,常用的函数有:
pthread_cond_init
:初始化条件变量。
pthread_cond_wait
:等待条件变量。
pthread_cond_signal
:发送信号。
pthread_cond_destroy
:销毁条件变量。
3、信号量:
信号量用于控制对共享资源的访问次数,适用于多个线程需要访问同一个资源的情况,常用的函数有:
sem_init
:初始化信号量。
sem_wait
:等待信号量。
sem_post
:增加信号量。
sem_destroy
:销毁信号量。
常见问题及解答FAQs
1、问题:为什么需要线程同步?
回答:线程同步是为了防止多个线程同时访问共享资源时发生竞态条件,从而保证数据的一致性和程序的正确性,当多个线程同时修改一个全局变量时,如果没有同步机制,最终结果可能会不可预知。
2、问题:如何选择合适的同步机制?
回答:选择同步机制应根据具体需求来决定,如果只需要保护临界区,可以使用互斥锁;如果需要在特定条件下进行线程间通信,可以使用条件变量;如果需要控制对资源的访问次数,可以使用信号量,根据具体应用场景选择最合适的同步机制可以提高程序的性能和可靠性。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1095550.html