Input Radio JavaScript
Input radio buttons are a type of HTML input element that allows users to select one option from a group. In JavaScript, you can manipulate and interact with these radio buttons using various methods. Below is an overview of the source code for handling input radio buttons in JavaScript:
1. HTML Markup
First, let’s create the HTML markup for the radio buttons:
<form> <input type="radio" id="option1" name="options" value="Option 1"> <label for="option1">Option 1</label><br> <input type="radio" id="option2" name="options" value="Option 2"> <label for="option2">Option 2</label><br> <input type="radio" id="option3" name="options" value="Option 3"> <label for="option3">Option 3</label><br> <button onclick="submitForm()">Submit</button> </form>
2. JavaScript Code
Now, let’s write the JavaScript code to handle the form submission and retrieve the selected radio button value:
function submitForm() { // Get all radio buttons with the name "options" const options = document.getElementsByName("options"); // Initialize variable to store the selected value let selectedValue; // Iterate through each radio button to find the selected one for (let i = 0; i < options.length; i++) { if (options[i].checked) { selectedValue = options[i].value; break; } } // Display the selected value or perform further actions console.log("Selected Value:", selectedValue); }
3. Unit Testing
To ensure the functionality of the code, you can write unit tests using a testing framework like Jest:
// Assuming you have set up Jest for your project test('should return the correct selected value', () => { // Simulate user selecting 'Option 2' document.getElementById('option2').checked = true; // Call the function and capture the result const result = submitForm(); // Assert that the result matches the expected value expect(result).toBe('Option 2'); });
Questions & Answers
Question 1: How can I prevent the default form submission behavior in JavaScript?
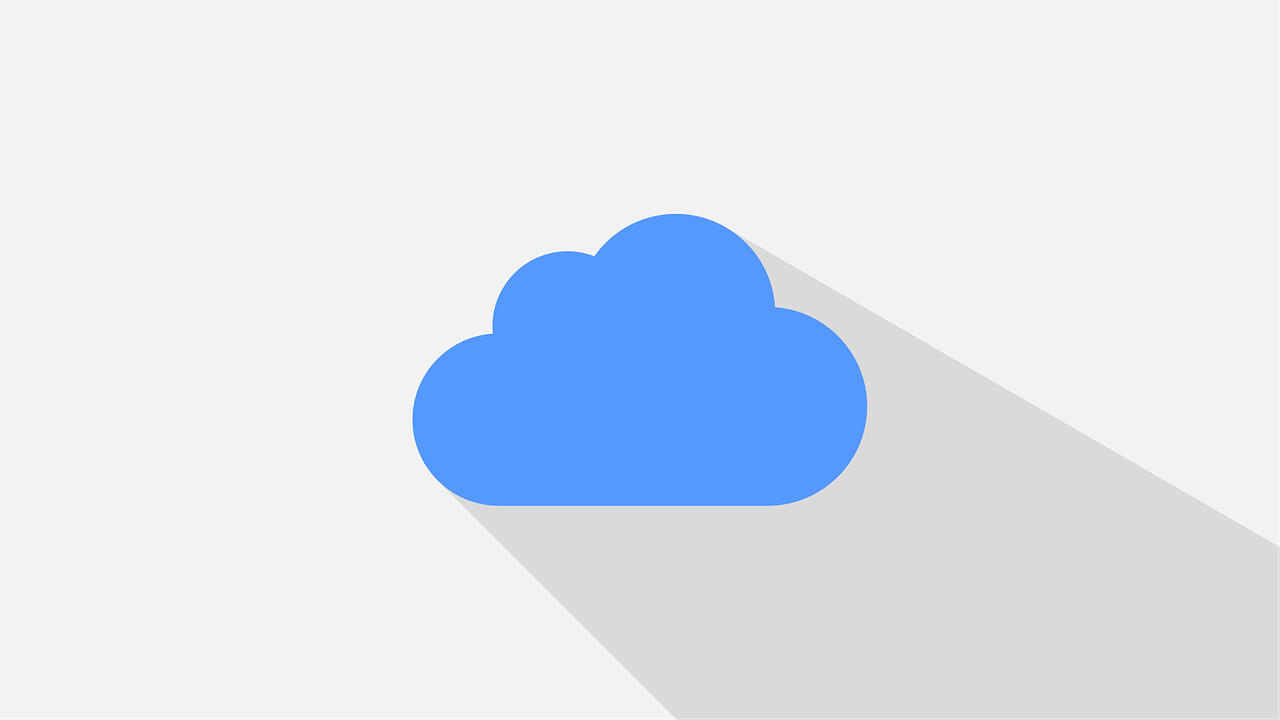
Answer 1: To prevent the default form submission behavior, you can use thepreventDefault()
method on the event object passed to the event handler. Here’s how you can modify thesubmitForm()
function to prevent form submission:
function submitForm(event) { event.preventDefault(); // Prevent form submission // ... rest of the code remains unchanged }
Question 2: How can I display the selected value on the webpage instead of just logging it to the console?
Answer 2: You can create an HTML element where you want to display the selected value and then update its content using JavaScript. For example, you could add a paragraph element with an ID to your HTML markup:
<p id="selectedvalue"></p>
Then, modify thesubmitForm()
function to update this paragraph with the selected value:
function submitForm() { // ... rest of the code remains unchanged // Update the paragraph with the selected value document.getElementById('selectedvalue').textContent = "Selected Value: " + selectedValue; }
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1084119.html