由于P2P网贷系统的源码非常庞大,无法在这里提供完整的代码,但我可以给你一个简单的Java示例,展示如何实现一个基本的P2P借贷系统的核心功能,这个示例包括用户、借款和投资的基本类以及一些简单的操作。
我们需要创建一个User
类来表示系统中的用户:
public class User { private String name; private double balance; public User(String name, double balance) { this.name = name; this.balance = balance; } public String getName() { return name; } public double getBalance() { return balance; } public void deposit(double amount) { balance += amount; } public boolean withdraw(double amount) { if (balance >= amount) { balance = amount; return true; } else { return false; } } }
我们创建一个Loan
类来表示借款:
public class Loan { private String id; private User borrower; private double amount; private double interestRate; private int termInMonths; public Loan(String id, User borrower, double amount, double interestRate, int termInMonths) { this.id = id; this.borrower = borrower; this.amount = amount; this.interestRate = interestRate; this.termInMonths = termInMonths; } // Getter and setter methods for the fields }
我们创建一个Investment
类来表示投资:
public class Investment { private String id; private User investor; private Loan loan; private double amount; public Investment(String id, User investor, Loan loan, double amount) { this.id = id; this.investor = investor; this.loan = loan; this.amount = amount; } // Getter and setter methods for the fields }
我们可以创建一个简单的测试类来模拟用户的存款、借款和投资操作:
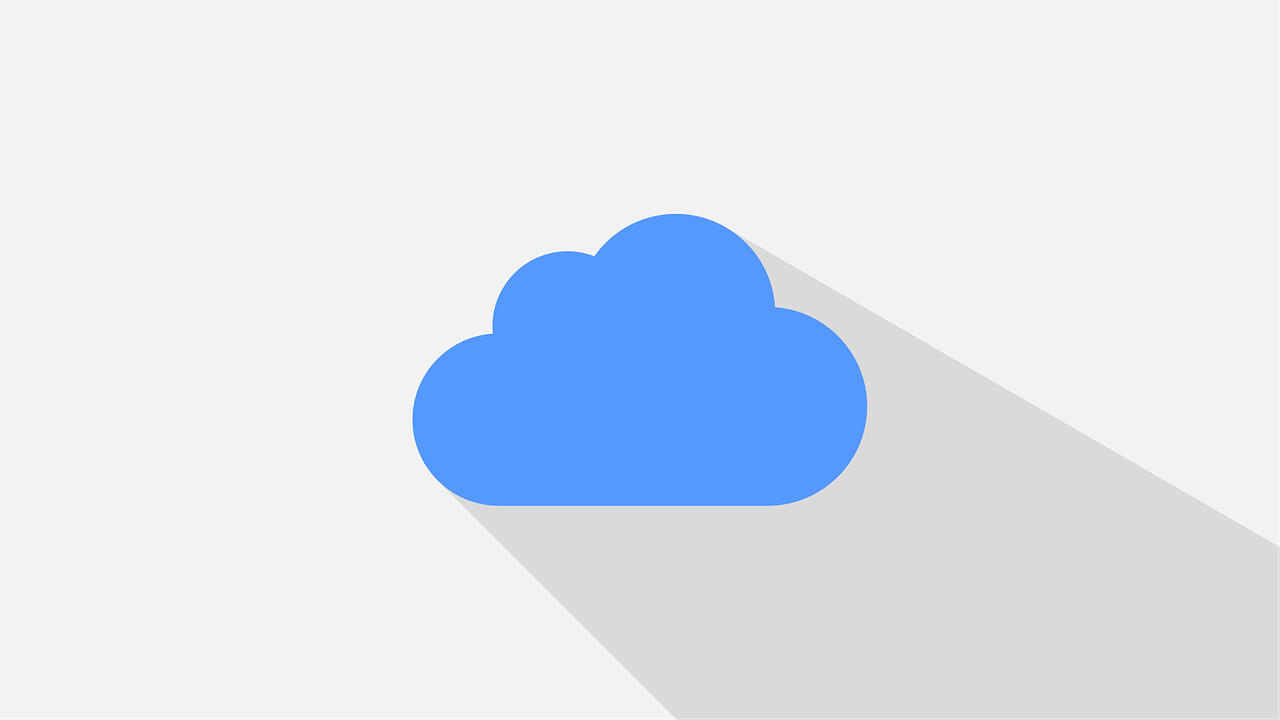
public class P2PLoanSystem { public static void main(String[] args) { User alice = new User("Alice", 1000); User bob = new User("Bob", 500); alice.deposit(200); // Alice存入200元 bob.withdraw(100); // Bob取出100元 Loan loan1 = new Loan("L1", alice, 500, 0.05, 12); // Alice借款500元,年利率5%,期限12个月 Investment investment1 = new Investment("I1", bob, loan1, 300); // Bob投资300元给Alice的借款 System.out.println("Alice's balance: " + alice.getBalance()); // Alice的余额应为1200元(1000+200) System.out.println("Bob's balance: " + bob.getBalance()); // Bob的余额应为400元(500100) } }
这只是一个非常简化的示例,实际的P2P借贷系统会涉及到更多的功能和复杂性,例如贷款审批、还款计划、风险评估等,你可以根据需要扩展这些类和方法来实现更复杂的功能。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1083130.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复