JS 导航样式
一、原生 JavaScript 实现的简单导航栏
1. HTML结构
<!DOCTYPE html> <html> <head> <meta httpequiv="ContentType" content="text/html; charset=utf8"> <title>导航栏1JS原生版</title> <style> * { margin: 0; padding: 0; } body { font: 12px/2 "Courier New"; } #header { width: 550px; height: 200px; margin: 20px auto; border: 1px solid #e1e1e1; } #nav { liststyletype: none; } #nav li { width: 110px; height: 35px; background: #fff; float: left; color: #000; textalign: center; lineheight: 35px; cursor: pointer; borderbottom: 1px solid #e1e1e1; fontweight: bold; } #nav li.active { background: #11ac63; color: #fff; } #content { padding: 50px 30px; } #content ul { display: none; } #content .list { display: block; } </style> </head> <body> <div id="header"> <ul id="nav"> <li class="active">华为</li> <li>苹果</li> <li>小米</li> <li>三星</li> <li>一加</li> </ul> <div id="content"> <ul class="list"> <li>华为 Mate20</li> <li>华为 P20</li> <li>华为荣耀 10</li> </ul> <ul> <li>iPhone XS</li> <li>iPhone XS MAX</li> <li>iPhone 8</li> </ul> <ul> <li>小米 8</li> <li>小米 8 SE</li> <li>小米 Play</li> </ul> <ul> <li>三星 Note 9</li> <li>三星 S9 +</li> <li>三星 A8s</li> </ul> <ul> <li>一加手机 6</li> <li>一加手机 6T</li> <li>一加手机 5T</li> </ul> </div> </div> <script> window.onload = function() { var aLi = document.getElementById("nav").getElementsByTagName("li"); var oUl = document.getElementById("content").getElementsByTagName("ul"); var i = 0; for (i = 0; i < aLi.length; i++) { aLi[i].index = i; aLi[i].onmouseover = function() { for (var x in aLi) { aLi[x].className = ""; oUl[x].className = ""; } this.className = "active"; oUl[this.index].className = "list"; }; aLi[i].onmouseout = function() { this.className = ""; aLi[this.index].className = "active"; }; } }; </script> </body> </html>
2. 主要特点和功能
通过改变li
元素的className
:切换背景色及文字颜色。
显示和隐藏内容:根据鼠标移动到不同的导航项,切换显示对应的内容。
使用原生 JavaScript 编写:无需依赖外部库。
基于 jQuery 的导航栏
1. HTML结构
<!DOCTYPE html> <html> <head> <meta httpequiv="ContentType" content="text/html; charset=utf8"> <title>导航栏2</title> <script src="https://code.jquery.com/jquery3.3.1.min.js"></script> <style> * { margin: 0; padding: 0; } body { font: 12px/2 "Courier New"; } #header { width: 550px; height: 200px; margin: 20px auto; border: 1px solid #e1e1e1; } #nav { liststyletype: none; } #nav li { width: 110px; height: 35px; background: #fff; float: left; color: #000; textalign: center; lineheight: 35px; cursor: pointer; borderbottom: 1px solid #e1e1e1; fontweight: bold; } #nav li.active { background: #11ac63; color: #fff; } #content { padding: 50px 30px; } #content ul { display: none; } #content .list { display: block; } </style> </head> <body> <div id="header"> <ul id="nav"> <li class="active">华为</li> <li>苹果</li> <li>小米</li> <li>三星</li> <li>一加</li> </ul> <div id="content"> <ul class="list"> <li>华为 Mate20</li> <li>华为 P20</li> <li>华为荣耀 10</li> </ul> <ul> <li>iPhone XS</li> <li>iPhone XS MAX</li> <li>iPhone 8</li> </ul> <ul> <li>小米 8</li> <li>小米 8 SE</li> <li>小米 Play</li> </ul> <ul> <li>三星 Note 9</li> <li>三星 S9 +</li> <li>三星 A8s</li> </ul> <ul> <li>一加手机 6</li> <li>一加手机 6T</li> <li>一加手机 5T</li> </ul> </div> </div> <script> $(document).ready(function() { $("#nav li").hover(function() { $("#nav li").removeClass("active"); //移除所有li的active类,避免重复添加类名导致的问题。 $(this).addClass("active"); //给当前元素添加active类,使其高亮显示。 var index = $(this).index(); //获取当前元素的索引值,用于定位对应的内容区域。 $("#content ul").hide(); //隐藏所有内容区域。 $("#content ul").eq(index).show(); //显示对应索引的内容区域。 }); }); </script> </body> </html>
2. 主要特点和功能
使用 jQuery:简化了代码复杂度。
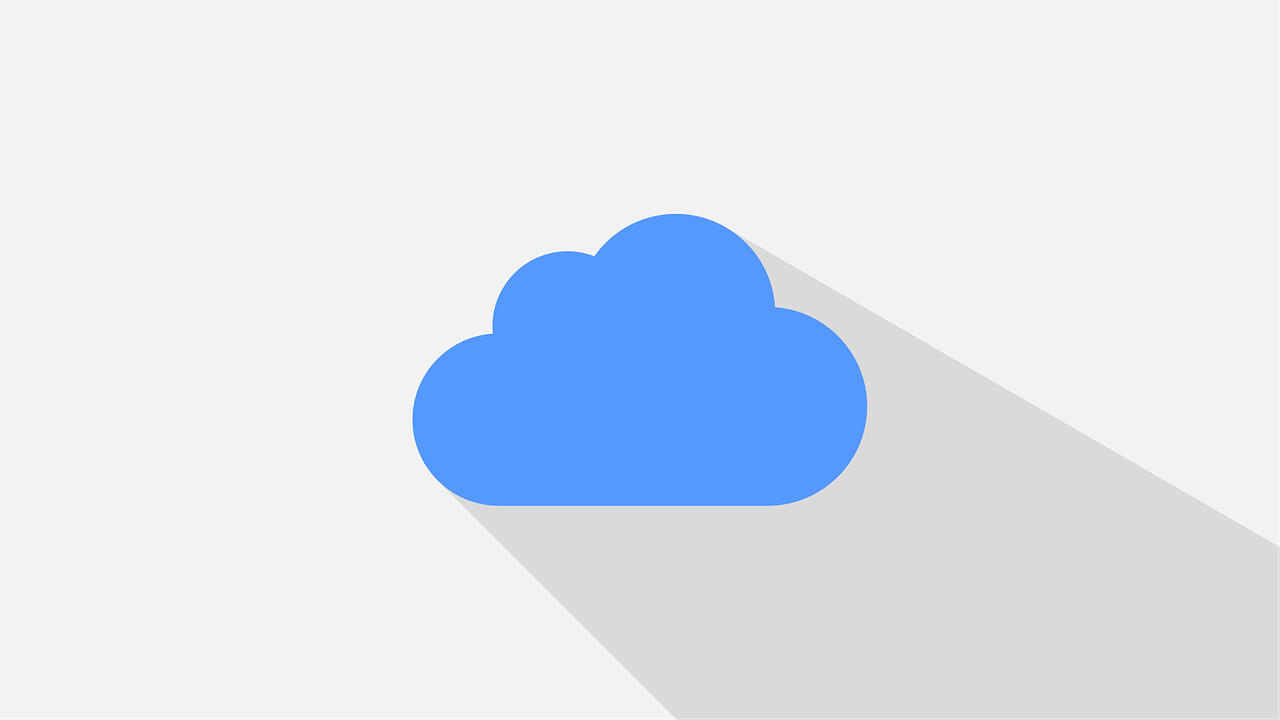
动态切换背景色和文字颜色:与原生 JavaScript 版本类似。
显示和隐藏内容:根据鼠标移动到不同的导航项,切换显示对应的内容。
更简洁的代码:由于使用了 jQuery,代码更加简洁易读。
响应式导航栏的实现
1. HTML结构
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF8"> <meta name="viewport" content="width=devicewidth, initialscale=1.0"> <title>导航栏</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="navbar" id="nav"> <div class="logo">saycode.cn</div> <div class="menuicon" id="menuIcon">☰</div> <ul id="navbarMenu"> <li><a href="#">首页</a></li> <li><a href="#">我的教程</a></li> <li><a href="#">我的创意</a></li> <div class="searchbox"> <input type="text" placeholder="Search..."> </div> <div class="buttons"> <button class="login">登录</button> <button class="register">注册</button> </div> </ul> </div> <script src="script.js"></script> </body> </html>
2. CSS样式(styles.css)
{ boxsizing: borderbox; } body { margin: 0; padding: 0; fontfamily: Arial, sansserif; backgroundcolor: #ffe7df; } .navbar { backgroundcolor: #ffffff; color: #fff; padding: 10px 40px; display: flex; alignitems: center; justifycontent: spacebetween; } .logo { fontsize: 24px; fontweight: bold; color: #232c53; } .navbar ul { liststyletype: none; margin: 0; padding: 0; display: flex; alignitems: center; } .navbar li { position: relative; marginright: 15px; } .navbar li a { color: #777b91; textdecoration: none; padding: 10px; } .navbar li a:hover { color: #141e46; } .searchbox input { width: 200px; height: 30px; border: none; padding: 5px 10px; borderradius: 6px; backgroundcolor: #eff6f8; } .buttons button { marginleft: 10px; padding: 5px 10px; border: none; cursor: pointer; } .login { color: #232c53; backgroundcolor: #fff; } .register { color: #fff; backgroundcolor: #232c53; borderradius: 10px; } @media screen and (maxwidth: 768px) { .navbar { position: relative; padding: 10px 10px; } .menuicon { display: block; cursor: pointer; } #navbarMenu { display: none; } .menuicon.open ~ #navbarMenu { display: block; } }
3. JavaScript交互(script.js)
const menuIcon = document.getElementById('menuIcon'); const navbarMenu = document.getElementById('navbarMenu'); menuIcon.addEventListener('click', () => { menuIcon.classList.toggle('open'); navbarMenu.classList.toggle('open'); }); window.addEventListener('resize', () => { if (window.innerWidth >= 768) { menuIcon.classList.remove('open'); navbarMenu.classList.remove('open'); } }); menuIcon.addEventListener('click', () => { menuIcon.classList.toggle('open'); navbarMenu.classList.toggle('open'); }); window.addEventListener('resize', () => { if (window.innerWidth >= 768) { menuIcon.classList.remove('open'); navbarMenu.classList.remove('open'); } }); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }()); }})); }})); }})); }})); }})); }})); }})); }})); }})); }})); }})); }})); }})); }})); }})); }})); }});}});}});}});}})); }});}});}});}})); }});}});}}); }});}});}});}})); }});}});}}); }});}});}});}})); }});}});}}); }});}});}});}})); }});}});}}); }});}});}});}})); }});}});}};
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1081702.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复