Button Click JavaScript
1. Introduction
JavaScript is a powerful scripting language that can be used to add interactivity and dynamic content to web pages. One of the most common uses of JavaScript is to handle button click events, allowing developers to define what should happen when a user clicks on a button.
2. Basic Structure of a Button Click Event Handler
To create a button click event handler in JavaScript, you need to follow these steps:
Step 1: Create the HTML Button
First, you need to create a button element in your HTML code. Here’s an example of a simple button:
<button id="myButton">Click me!</button>
Step 2: Add an Event Listener
Next, you need to write JavaScript code to add an event listener to the button. The event listener will listen for theclick
event and execute a function when the event occurs.
// Get the button element by its ID const button = document.getElementById('myButton'); // Define the function to be executed when the button is clicked function handleClick() { alert('Button was clicked!'); } // Add the event listener to the button button.addEventListener('click', handleClick);
Step 3: Test the Button Click Event
Now, when you open your web page and click the button, an alert box with the message "Button was clicked!" should appear.
3. Advanced Usage
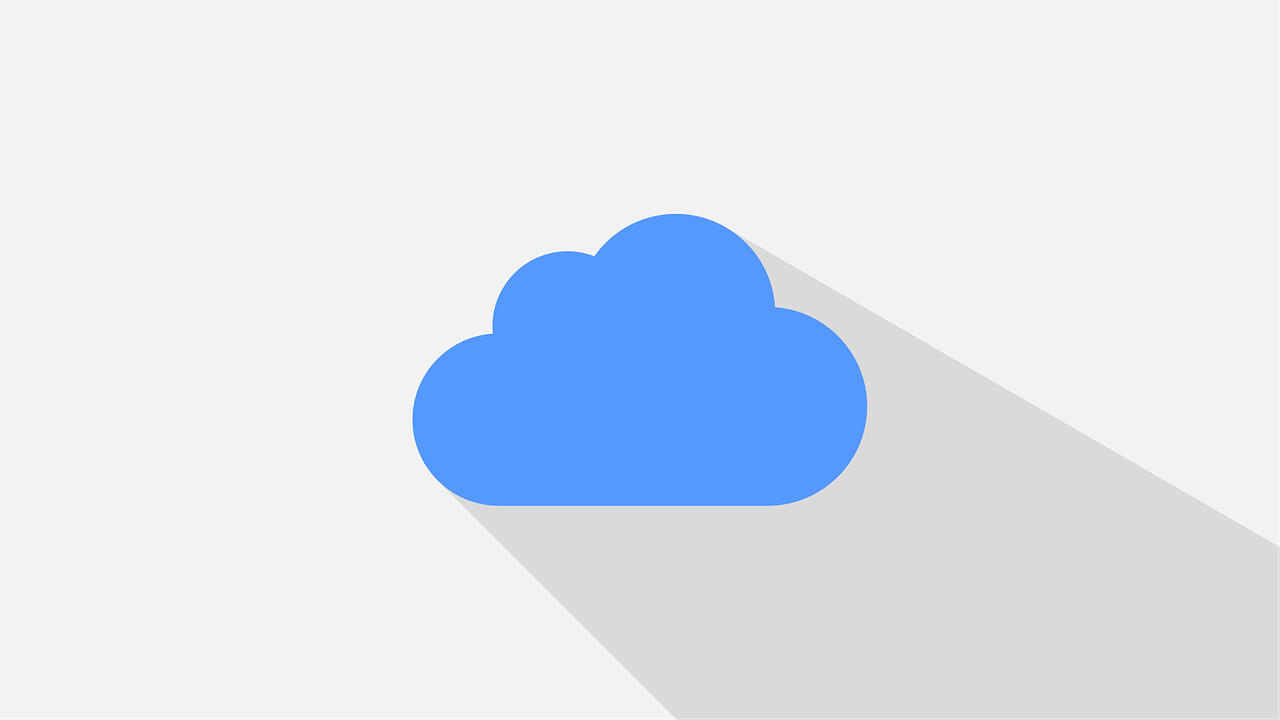
You can also pass additional parameters to the event handler function, such as the event object itself or any other data you might need. Here’s an example:
function handleClick(event) { console.log('Button was clicked!', event); } button.addEventListener('click', handleClick);
4. Related Questions & Answers
Question 1: How can I prevent the default behavior of a button click event?
Answer 1: To prevent the default behavior of a button click event, you can use thepreventDefault()
method within your event handler function. This method stops the browser from performing the default action associated with the event. Here’s an example:
function handleClick(event) { event.preventDefault(); // Prevent the default behavior console.log('Button was clicked!', event); } button.addEventListener('click', handleClick);
Question 2: How can I remove an event listener after it has been added?
Answer 2: To remove an event listener, you can use theremoveEventListener()
method. You need to pass the same function reference that was used withaddEventListener()
to successfully remove the listener. Here’s an example:
function handleClick() { alert('Button was clicked!'); } button.addEventListener('click', handleClick); // Later in your code, when you want to remove the event listener button.removeEventListener('click', handleClick);
Remember that if you use an anonymous function or an arrow function as the event handler, you won’t be able to remove it later because you won’t have a reference to it. Always use named functions if you plan to remove the event listener later.
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1081055.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复