由于篇幅原因,我无法为您提供完整的考试系统源码,我可以为您提供一个简单的Java考试系统的基本结构和关键代码片段,您可以根据这些信息自行扩展和完善。
我们需要创建一个考试类(Exam)和一个学生类(Student):
public class Exam { private String title; private List<Question> questions; public Exam(String title, List<Question> questions) { this.title = title; this.questions = questions; } // getter和setter方法 } public class Question { private String content; private List<String> options; private int correctAnswerIndex; public Question(String content, List<String> options, int correctAnswerIndex) { this.content = content; this.options = options; this.correctAnswerIndex = correctAnswerIndex; } // getter和setter方法 }
我们创建一个学生类(Student),用于存储学生的姓名和得分:
public class Student { private String name; private int score; public Student(String name) { this.name = name; this.score = 0; } // getter和setter方法 }
我们需要一个考试管理类(ExamManager),用于处理考试的逻辑:
import java.util.List; public class ExamManager { private Exam exam; private Student student; public ExamManager(Exam exam, Student student) { this.exam = exam; this.student = student; } public void startExam() { // 显示考试题目并获取学生的答案 // 计算得分并更新学生对象 } public void showResults() { // 显示学生的得分和正确答案 } }
我们可以在主类(Main)中创建考试、学生和考试管理器对象,并调用相应的方法来开始考试和显示结果:
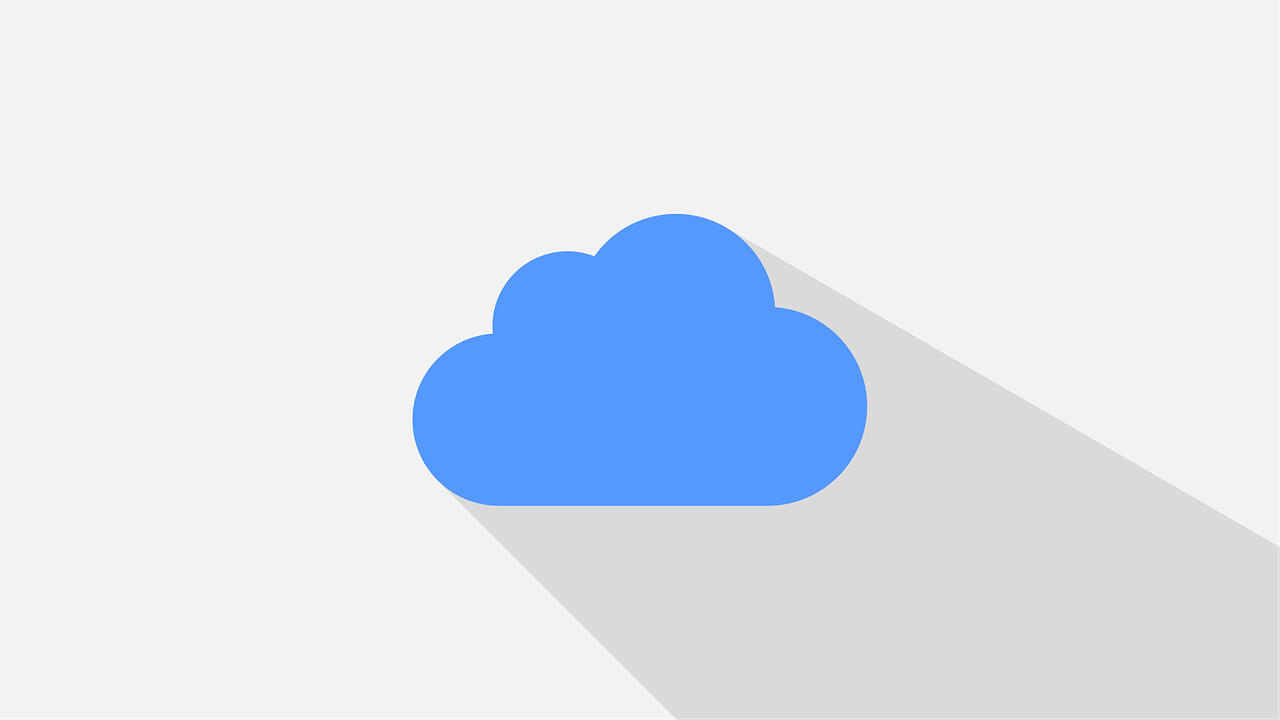
import java.util.ArrayList; import java.util.Arrays; public class Main { public static void main(String[] args) { // 创建考试题目列表 List<Question> questions = new ArrayList<>(); questions.add(new Question("问题1", Arrays.asList("选项A", "选项B", "选项C", "选项D"), 2)); questions.add(new Question("问题2", Arrays.asList("选项A", "选项B", "选项C", "选项D"), 1)); questions.add(new Question("问题3", Arrays.asList("选项A", "选项B", "选项C", "选项D"), 3)); // 创建考试对象 Exam exam = new Exam("Java考试", questions); // 创建学生对象 Student student = new Student("张三"); // 创建考试管理器对象 ExamManager examManager = new ExamManager(exam, student); // 开始考试 examManager.startExam(); // 显示结果 examManager.showResults(); } }
这只是一个简单的示例,实际的考试系统可能需要更多的功能,例如支持多个学生、记录每个学生的成绩、提供在线答题等,您可以根据自己的需求进行扩展和完善。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1079264.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复