CMS(内容管理系统)是一种基于Java的开源框架,用于快速开发和部署Web应用程序。它具有丰富的功能和灵活性,支持多种数据库系统,并提供了一套完整的API和标签库,以便于开发人员进行二次开发和扩展。
CMS(内容管理系统)是一种用于管理网站内容的系统,在Java中,我们可以使用各种框架和库来构建CMS,以下是一个简单的Java CMS源码示例,使用了Spring Boot和Thymeleaf模板引擎。
1、创建一个新的Spring Boot项目,添加以下依赖项到pom.xml
文件中:
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>springbootstarterweb</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>springbootstarterthymeleaf</artifactId> </dependency> </dependencies>
2、创建一个Article
实体类,用于表示文章内容:
public class Article { private Long id; private String title; private String content; // 构造函数、getter和setter方法省略 }
3、创建一个ArticleRepository
接口,用于操作数据库:
import org.springframework.data.repository.CrudRepository; public interface ArticleRepository extends CrudRepository<Article, Long> { }
4、创建一个ArticleController
类,用于处理HTTP请求:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestParam; @Controller public class ArticleController { @Autowired private ArticleRepository articleRepository; @GetMapping("/") public String index(Model model) { model.addAttribute("articles", articleRepository.findAll()); return "index"; } @GetMapping("/new") public String newArticle() { return "new"; } @PostMapping("/save") public String saveArticle(@RequestParam("title") String title, @RequestParam("content") String content) { Article article = new Article(); article.setTitle(title); article.setContent(content); articleRepository.save(article); return "redirect:/"; } }
5、创建src/main/resources/templates
目录下的HTML模板文件:
index.html
:显示文章列表和新建文章按钮
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>CMS Example</title> </head> <body> <h1>Articles</h1> <ul> <li th:each="article : ${articles}"> <h2 th:text="${article.title}"></h2> <p th:text="${article.content}"></p> </li> </ul> <a href="/new">New Article</a> </body> </html>
new.html
:显示新建文章表单
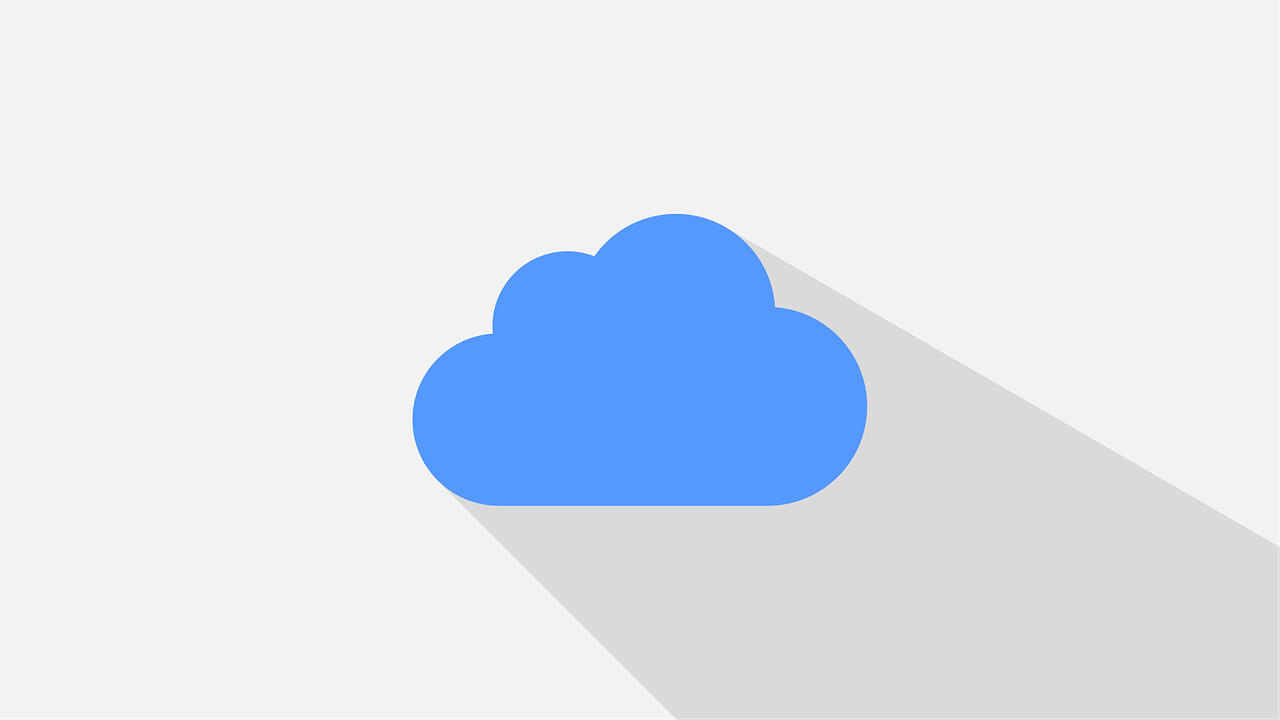
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>New Article</title> </head> <body> <h1>New Article</h1> <form action="/save" method="post"> <label for="title">Title:</label> <input type="text" id="title" name="title" required> <br> <label for="content">Content:</label> <textarea id="content" name="content" required></textarea> <br> <button type="submit">Save</button> </form> </body> </html>
6、创建一个Application
类,启动Spring Boot应用:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } }
你可以运行这个简单的CMS应用,访问http://localhost:8080
查看文章列表,点击“New Article”链接创建新文章。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1077471.html