1. 使用Node.js和docx库创建Word文档
安装依赖
你需要安装nodedocx
库,在项目目录下运行以下命令:
npm install save nodedocx
示例代码
以下是一个简单的示例,演示如何使用nodedocx
库创建一个包含标题和段落的Word文档。
const fs = require('fs'); const { Document, Packer, Paragraph, TextRun } = require('nodedocx'); // 创建一个新的文档对象 const doc = new Document(); // 添加一个标题 const titleParagraph = new Paragraph({ children: [ new TextRun({ text: 'Hello World', bold: true, size: 28 }), ], alignment: 'center', }); doc.addSection({ children: [titleParagraph], }); // 添加一个段落 const paragraph = new Paragraph({ children: [ new TextRun({ text: 'This is a simple paragraph.' }), ], }); doc.addSection({ children: [paragraph], }); // 将文档保存为Word文件 Packer.toBuffer(doc).then((buffer) => { fs.writeFileSync('example.docx', buffer); });
2. 使用JS操作Word文档的其他功能
插入图片
要在Word文档中插入图片,可以使用ImageRun
类,以下是一个示例:
const imagePath = './path/to/image.jpg'; const imageSize = 100; // 图片大小(单位:百分比) const imageParagraph = new Paragraph({ children: [ new ImageRun({ data: fs.readFileSync(imagePath), transformation: { width: imageSize } }), ], }); doc.addSection({ children: [imageParagraph], });
插入表格
要插入表格,可以使用Table
类,以下是一个示例:
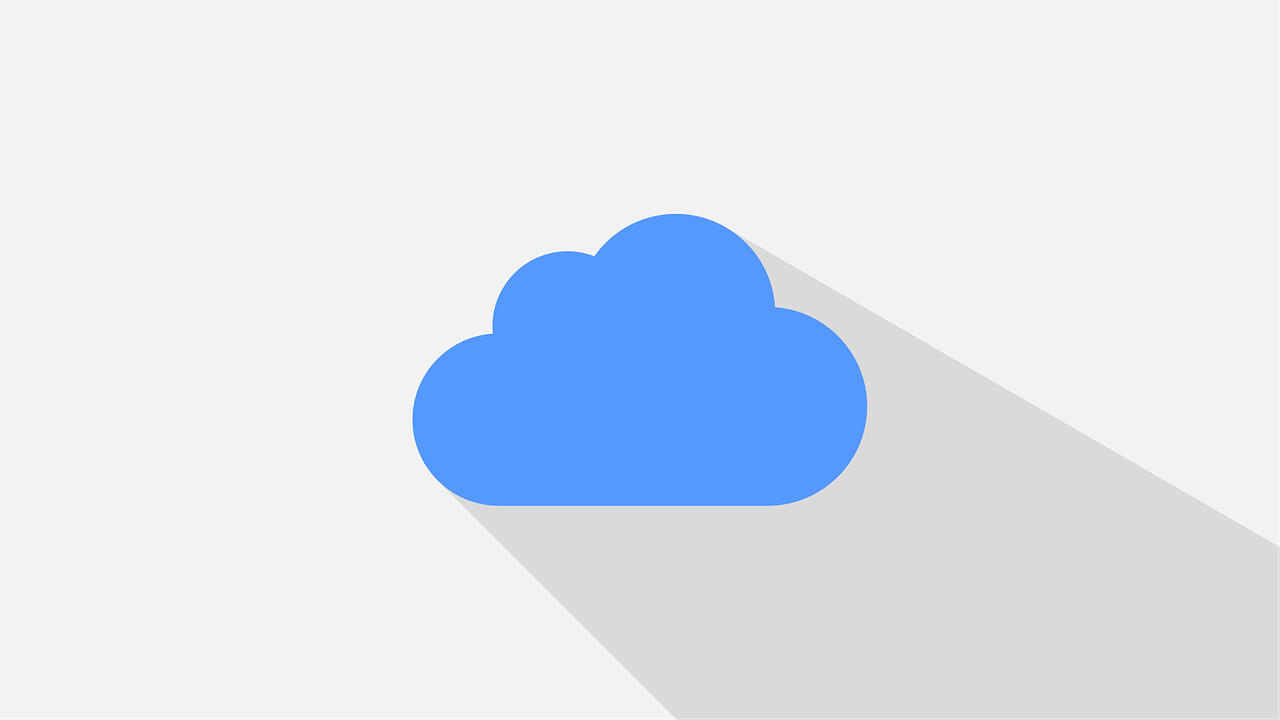
const table = new Table({ rows: [ new TableRow({ children: [ new TableCell({ children: [new Paragraph(new TextRun({ text: 'Header 1' }))], }), new TableCell({ children: [new Paragraph(new TextRun({ text: 'Header 2' }))], }), ], }), new TableRow({ children: [ new TableCell({ children: [new Paragraph(new TextRun({ text: 'Row 1, Cell 1' }))], }), new TableCell({ children: [new Paragraph(new TextRun({ text: 'Row 1, Cell 2' }))], }), ], }), ], }); doc.addSection({ children: [table], });
相关问题与解答
问题1:如何修改Word文档中的字体样式?
答:可以通过设置TextRun
对象的fontFace
属性来修改字体样式,要将字体设置为“Arial”,可以这样做:
new TextRun({ text: 'Hello World', fontFace: 'Arial' })
问题2:如何在Word文档中插入超链接?
答:可以使用HyperlinkText
类来插入超链接,以下是一个示例:
const hyperlinkParagraph = new Paragraph({ children: [ new HyperlinkText({ text: 'Visit Google', address: 'https://www.google.com', }), ], }); doc.addSection({ children: [hyperlinkParagraph], });
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1074050.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复