JS Button Input
1. Introduction
The<button>
element in HTML is used to create a clickable button that can trigger an action when clicked. In JavaScript, you can interact with this button by adding event listeners to it. The following sections will provide a detailed overview of the source code for creating and handling a button input using JavaScript.
2. HTML Code
First, let’s create the HTML structure for the button:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF8"> <meta name="viewport" content="width=devicewidth, initialscale=1.0"> <title>Button Example</title> </head> <body> <button id="myButton">Click me!</button> <script src="script.js"></script> </body> </html>
In this example, we have a single button with the text "Click me!" and an ID attribute set to "myButton". The script tag at the end of the body links to an external JavaScript file called "script.js".
3. JavaScript Code
Now, let’s write the JavaScript code to handle the button click event:
// script.js document.addEventListener('DOMContentLoaded', function() { var button = document.getElementById('myButton'); button.addEventListener('click', function() { alert('Button was clicked!'); }); });
Here’s what the code does:
1、It waits for the DOM content to be fully loaded using theDOMContentLoaded
event.
2、It retrieves the button element by its ID usingdocument.getElementById('myButton')
.
3、It adds a click event listener to the button usingaddEventListener('click', function() {...})
.
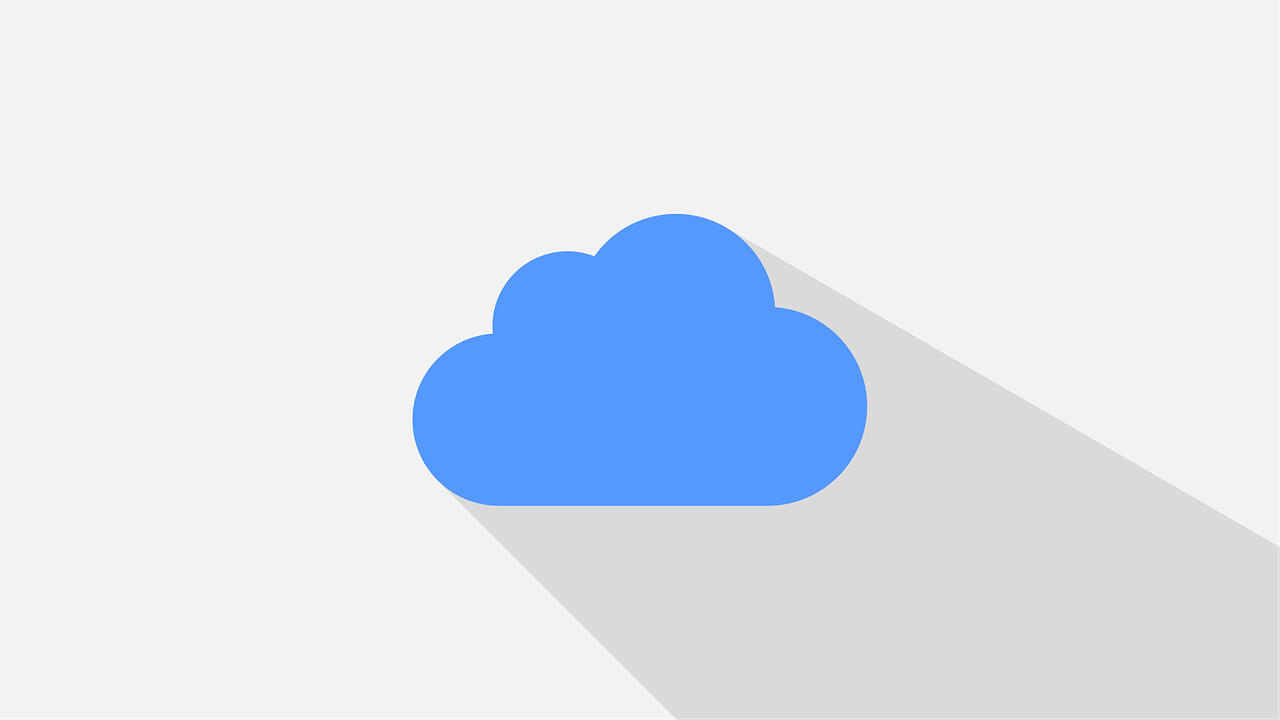
4、When the button is clicked, an alert box with the message "Button was clicked!" is displayed.
4. Conclusion
This section provided a basic introduction to creating and handling a button input using HTML and JavaScript. You can further customize the behavior of the button by modifying the JavaScript code or adding more event listeners to handle different types of events.
5. Questions & Answers
Q1: How can I change the text on the button after it has been clicked?
A1: You can change the text of the button inside the event listener function like this:
button.addEventListener('click', function() { alert('Button was clicked!'); button.innerHTML = 'Clicked!'; // Change the button text to 'Clicked!' });
Q2: How can I prevent the default behavior of the button?
A2: To prevent the default behavior of the button (like submitting a form), you can use thepreventDefault()
method within the event listener:
button.addEventListener('click', function(event) { event.preventDefault(); // Prevent the default behavior of the button alert('Button was clicked!'); });
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1030621.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复