javascript,function createPuzzle(image) {, // 将图片分割成小块,并打乱顺序, const pieces = splitImage(image);, shuffle(pieces);, , // 在页面上显示打乱后的拼图块, displayPieces(pieces);,},,function splitImage(image) {, // 分割图片的逻辑,},,function shuffle(array) {, // 随机打乱数组顺序的逻辑,},,function displayPieces(pieces) {, // 在页面上显示拼图块的逻辑,},
`,,这段代码定义了一个
createPuzzle`函数,用于生成拼图。它首先将图片分割成小块,然后打乱这些小块的顺序,最后在页面上显示打乱后的拼图块。拼图游戏代码解析
拼图游戏是一种常见的益智游戏,玩家需要将乱序的图片块重新组合成完整的图片,以下是一个简单的 JavaScript 拼图游戏的源码解析:
HTML 结构
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF8"> <title>拼图游戏</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div id="puzzlecontainer"> <! 拼图块将被插入到这里 > </div> <script src="puzzle.js"></script> </body> </html>
CSS 样式
/* styles.css */ #puzzlecontainer { position: relative; width: 400px; height: 400px; } .puzzlepiece { position: absolute; width: 100px; height: 100px; backgroundimage: url('puzzleimage.jpg'); border: 1px solid #ccc; }
JavaScript 逻辑
// puzzle.js const puzzleContainer = document.getElementById('puzzlecontainer'); const pieces = []; // 存储拼图块的数组 const pieceCount = 9; // 假设拼图有 9 个块 // 创建拼图块并添加到容器中 for (let i = 0; i < pieceCount; i++) { const piece = document.createElement('div'); piece.classList.add('puzzlepiece'); piece.style.left =${(i % 3) * 100}px
; piece.style.top =${Math.floor(i / 3) * 100}px
; piece.dataset.index = i; // 为每个拼图块分配一个索引 puzzleContainer.appendChild(piece); pieces.push(piece); } // 添加拖拽功能 let draggingPiece = null; let startPosition = null; pieces.forEach(piece => { piece.addEventListener('mousedown', (e) => { draggingPiece = e.target; startPosition = { x: e.clientX, y: e.clientY }; }); }); document.addEventListener('mousemove', (e) => { if (!draggingPiece) return; const offsetX = e.clientX startPosition.x; const offsetY = e.clientY startPosition.y; draggingPiece.style.left =${parseInt(draggingPiece.style.left) + offsetX}px
; draggingPiece.style.top =${parseInt(draggingPiece.style.top) + offsetY}px
; }); document.addEventListener('mouseup', () => { draggingPiece = null; startPosition = null; });
问题与解答
问题1:如何实现拼图块之间的交换?
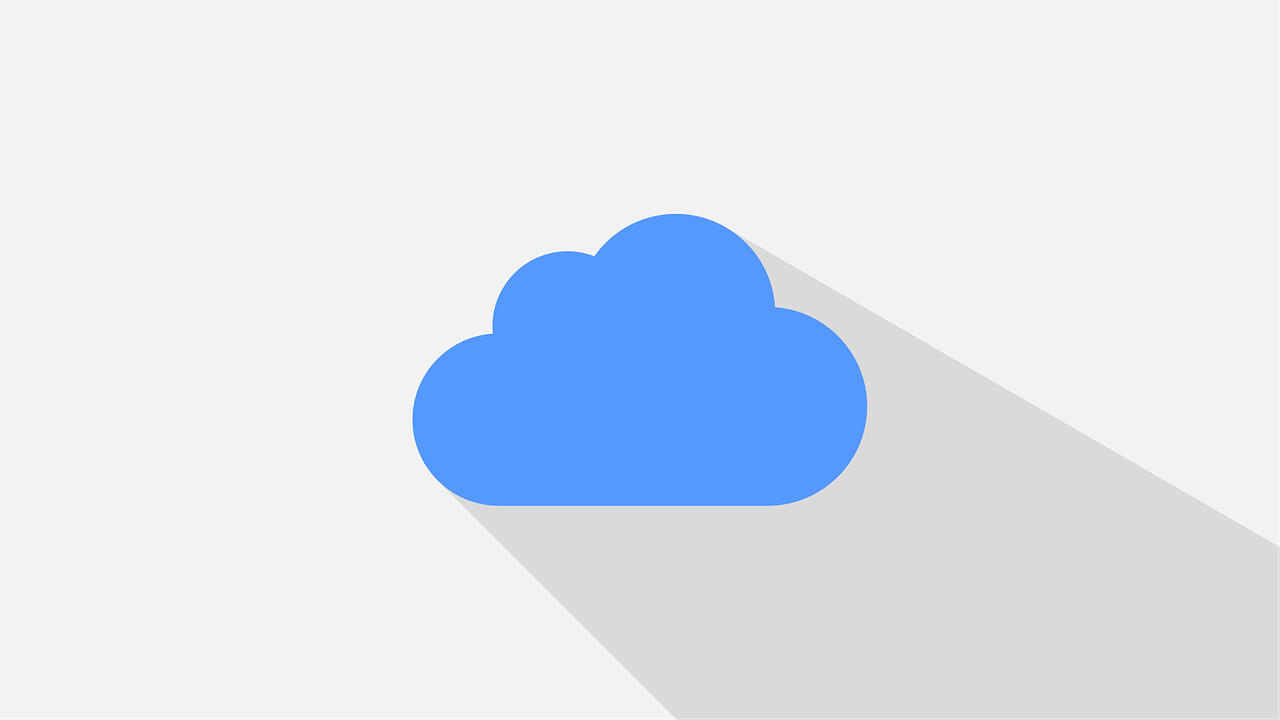
解答:可以通过监听鼠标事件来实现拼图块之间的交换,当用户拖动一个拼图块时,可以检测它与其他拼图块的碰撞,并在碰撞发生时交换它们的位置,具体实现如下:
pieces.forEach(piece => { piece.addEventListener('mousedown', (e) => { draggingPiece = e.target; startPosition = { x: e.clientX, y: e.clientY }; }); }); document.addEventListener('mousemove', (e) => { if (!draggingPiece) return; const offsetX = e.clientX startPosition.x; const offsetY = e.clientY startPosition.y; draggingPiece.style.left =${parseInt(draggingPiece.style.left) + offsetX}px
; draggingPiece.style.top =${parseInt(draggingPiece.style.top) + offsetY}px
; // 检测碰撞并交换位置的逻辑... });
问题2:如何判断拼图是否已经完成?
解答:可以通过比较每个拼图块的当前位置与其目标位置来判断拼图是否完成,如果所有拼图块都位于正确的位置上,则拼图已完成,具体实现如下:
function isPuzzleComplete() { for (let i = 0; i < pieces.length; i++) { const piece = pieces[i]; const targetLeft = (i % 3) * 100; const targetTop = Math.floor(i / 3) * 100; if (parseInt(piece.style.left) !== targetLeft || parseInt(piece.style.top) !== targetTop) { return false; } } return true; }
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1030407.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复