npm install oracledb
命令安装模块。之后,你可以使用如下代码示例连接到Oracle数据库并执行查询:,,“javascript,const oracledb = require('oracledb');,,async function run() {, let connection;,, try {, connection = await oracledb.getConnection({, user: 'yourusername',, password: 'yourpassword',, connectString: 'yourconnectionstring', });,, const result = await connection.execute('SELECT * FROM yourtable');, console.log(result.rows);, } catch (err) {, console.error(err);, } finally {, if (connection) {, try {, await connection.close();, } catch (err) {, console.error(err);, }, }, },},,run();,
`,,请将上述代码中的
yourusername、
yourpassword、
yourconnectionstring和
yourtable`替换为你的Oracle数据库的实际信息。在JavaScript中连接Oracle数据库主要依赖于Node.js环境以及相关的驱动程序,以下步骤将详细指导您如何在JavaScript中实现与Oracle数据库的连接,包括必要的安装、配置和代码编写过程。
安装OracleDB驱动程序
要在JavaScript中使用Oracle数据库,首先需要确保您的系统中安装了Node.js环境,并在您的项目中安装了OracleDB驱动程序,可以通过在终端中运行以下命令来安装Oracledb:
npm install oracledb
确保安装过程顺利完成,这是连接Oracle数据库的第一步。
创建数据库连接
安装完成后,可以使用以下代码示例来创建一个简单的数据库连接:
const oracledb = require('oracledb'); let connection = oracledb.getConnection({ user: "yourusername", password: "yourpassword", connectString: "yourconnectionstring" }); connection.then(function(conn) { // 连接成功,可以在这里执行数据库操作 console.log("Connected to Oracle Database"); }).catch(function(err) { // 处理连接错误 console.error("Error connecting to Oracle Database", err); });
请替换yourusername
、yourpassword
和yourconnectionstring
为您的实际数据库信息。
使用连接池
为了更高效地管理数据库连接,建议使用连接池,以下是如何创建一个连接池并使用它来连接到数据库的示例:
const oracledb = require('oracledb'); let pool; oracledb.createPool({ user: "yourusername", password: "yourpassword", connectString: "yourconnectionstring", poolMin: 4, // 最小连接数 poolMax: 10, // 最大连接数 poolIncrement: 0 // 按需增加连接 }).then(function(connPool) { pool = connPool; console.log("Connection pool created"); }).catch(function(err) { console.error("Error creating connection pool", err); }); // 使用连接池获取连接并执行查询 pool.getConnection().then(function(conn) { // 使用连接对象进行查询等操作 }).catch(function(err) { console.error("Error getting connection from the pool", err); });
通过这种方式,您可以更加灵活和高效地管理多个数据库连接。
关闭连接和连接池
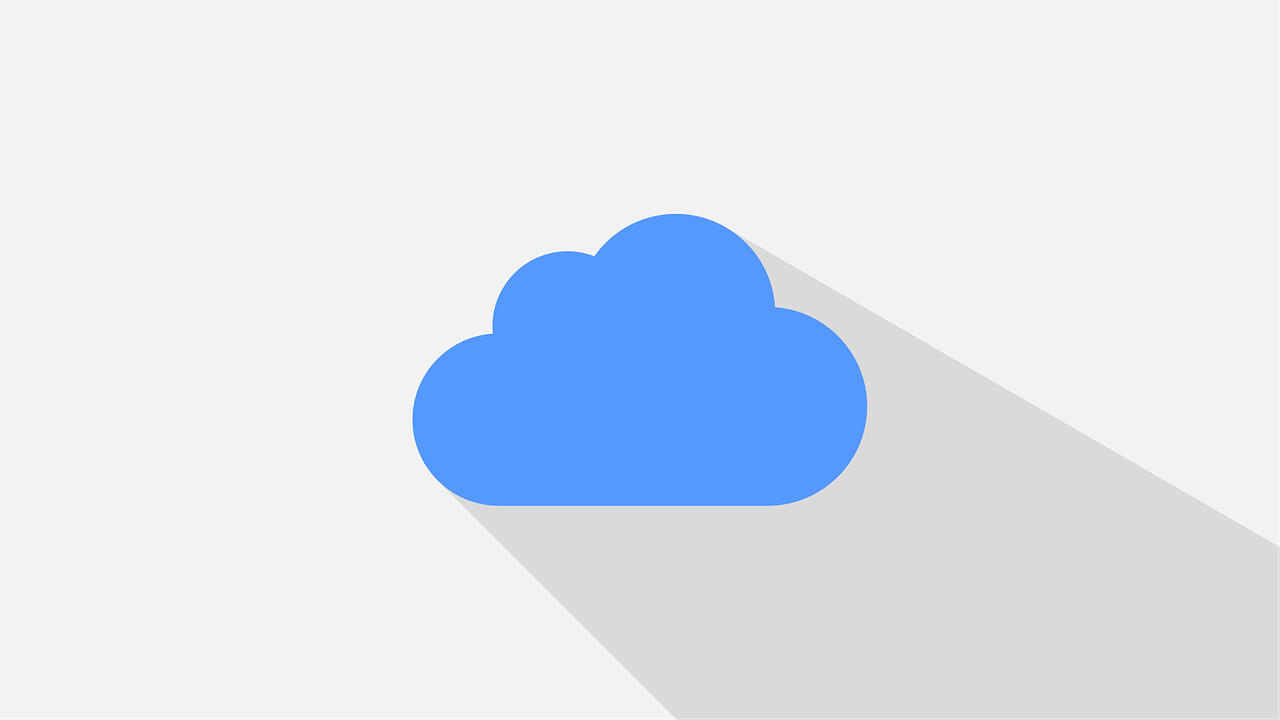
使用完毕后,记得关闭您的连接和连接池以释放资源:
connection.close(); // 关闭单个连接 pool.close(); // 关闭连接池
相关问题与解答
Q1: 安装OracleDB驱动时遇到问题怎么办?
A1: 确保您的网络连接正常,并检查Node.js环境是否正确安装,如果问题持续存在,可以尝试在不同的网络环境下重新安装或联系OracleDB支持团队。
Q2: 使用连接池有什么好处?
A2: 使用连接池可以显著提高应用程序性能,因为池化技术允许重复使用现有连接,减少因频繁打开/关闭连接而产生的开销,连接池还可以帮助管理连接的最大数量,防止资源过度使用。
通过上述步骤和注意事项,您可以在JavaScript环境中有效地连接到Oracle数据库,并进行相应的数据库操作。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1028249.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复