C语言中的strcat函数用于将一个字符串追加到另一个字符串的末尾,它的原型如下:
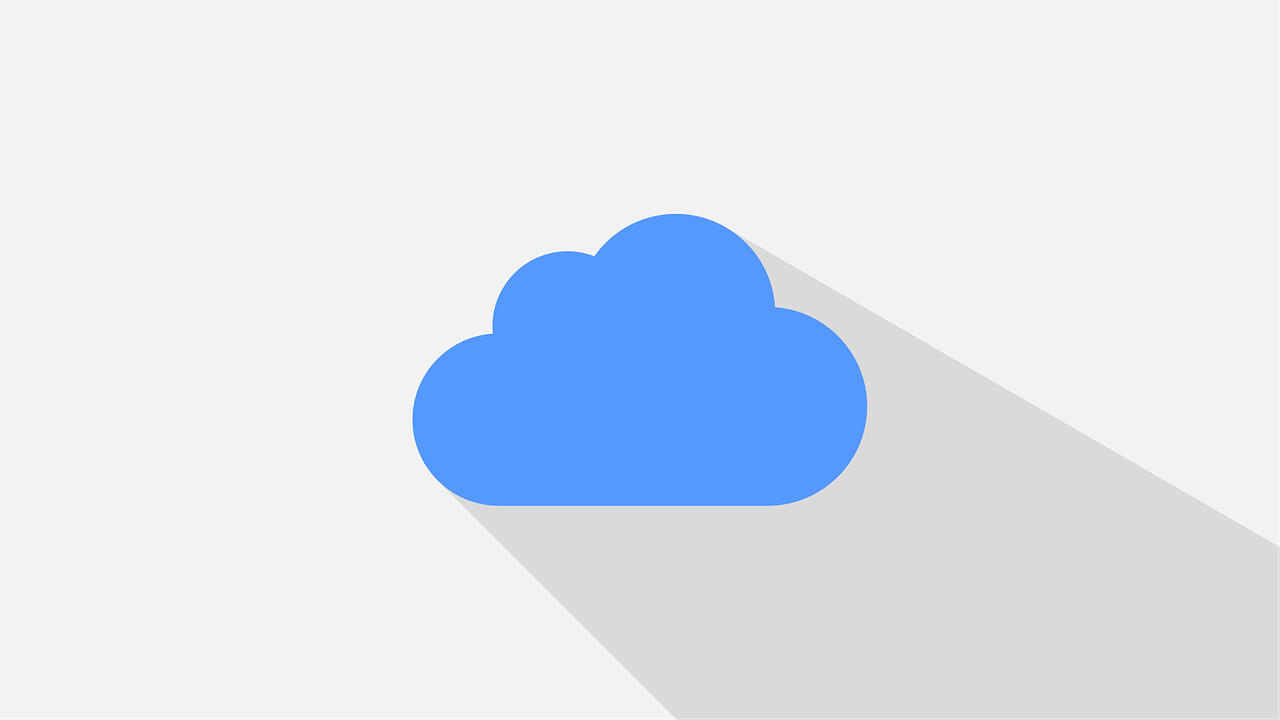
(图片来源网络,侵删)
char *strcat(char *dest, const char *src);
dest
是目标字符串,src
是要追加的字符串,函数返回目标字符串的指针。
下面是一个简单的示例,演示了如何使用strcat函数:
#include <stdio.h> #include <string.h> int main() { char dest[20] = "Hello, "; const char *src = "World!"; char result[25]; strcat(dest, src); // 将src追加到dest的末尾 result[0] = '