“getline” 是 C++ 中用于从输入流读取一行文本的函数。
在C++编程中,getline
是一个非常有用的函数,用于从输入流中读取一整行文本,与cin >>
不同,getline
可以读取包含空格的整行字符串,这使得它在处理用户输入时更加灵活,本文将详细介绍getline
的使用方式、常见用法以及一些注意事项。
`getline` 的基本语法
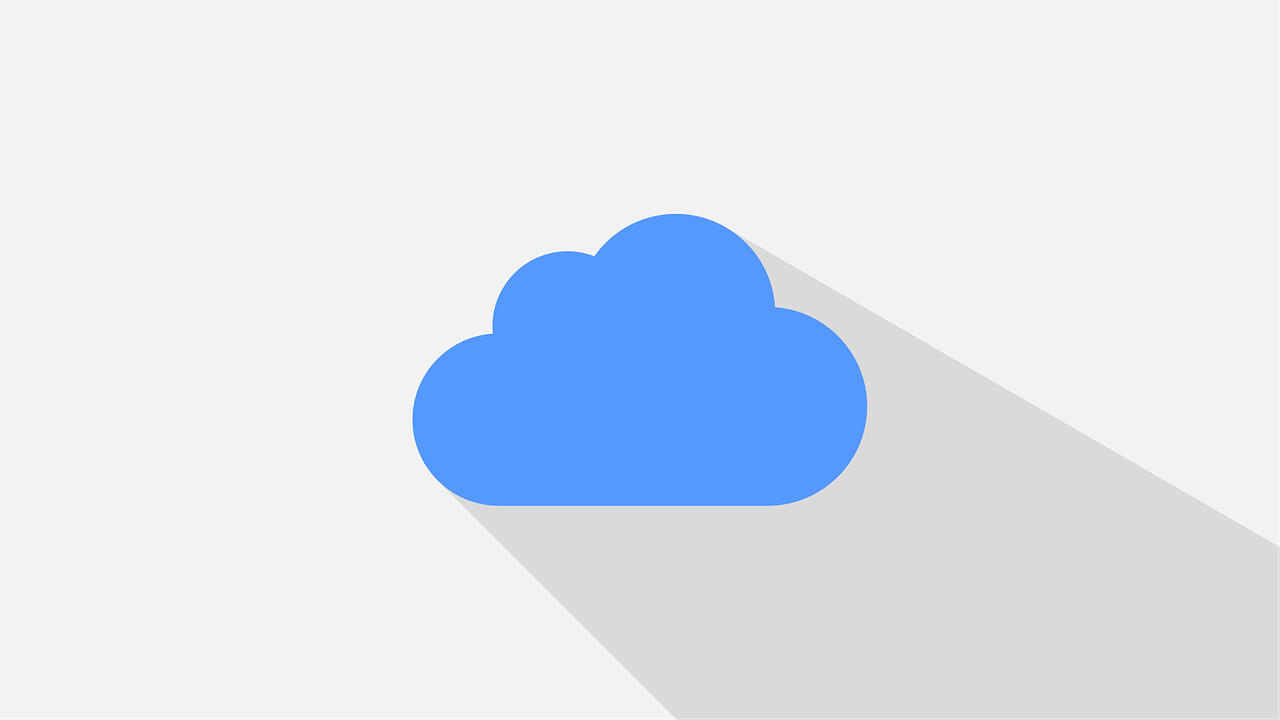
getline
是标准库中的函数,定义在头文件<string>
和<iostream>
中,其基本语法如下:
#include <iostream> #include <string> int main() { std::string line; // 从标准输入读取一行 std::getline(std::cin, line); std::cout << "You entered: " << line << std::endl; return 0; }
在上面的例子中,std::getline(std::cin, line)
从标准输入(通常是键盘)读取一行文本,并将其存储在字符串变量line
中。
`getline` 的参数解析
getline
函数有两个主要参数:
输入流:通常为std::cin
,但也可以是其他输入流,如文件流。
字符串引用:用于存储读取到的行内容。
getline
还有一个可选的第三个参数,即分隔符,默认为换行符 `’
‘`。
std::getline(std::cin, line, delimiter);
使用示例
示例1:从控制台读取多行输入
#include <iostream> #include <string> int main() { std::string line; std::cout << "Enter lines of text (type 'exit' to quit):" << std::endl; while (true) { std::getline(std::cin, line); if (line == "exit") { break; } std::cout << "You entered: " << line << std::endl; } return 0; }
在这个例子中,程序会不断读取用户输入的每一行,直到用户输入exit
。
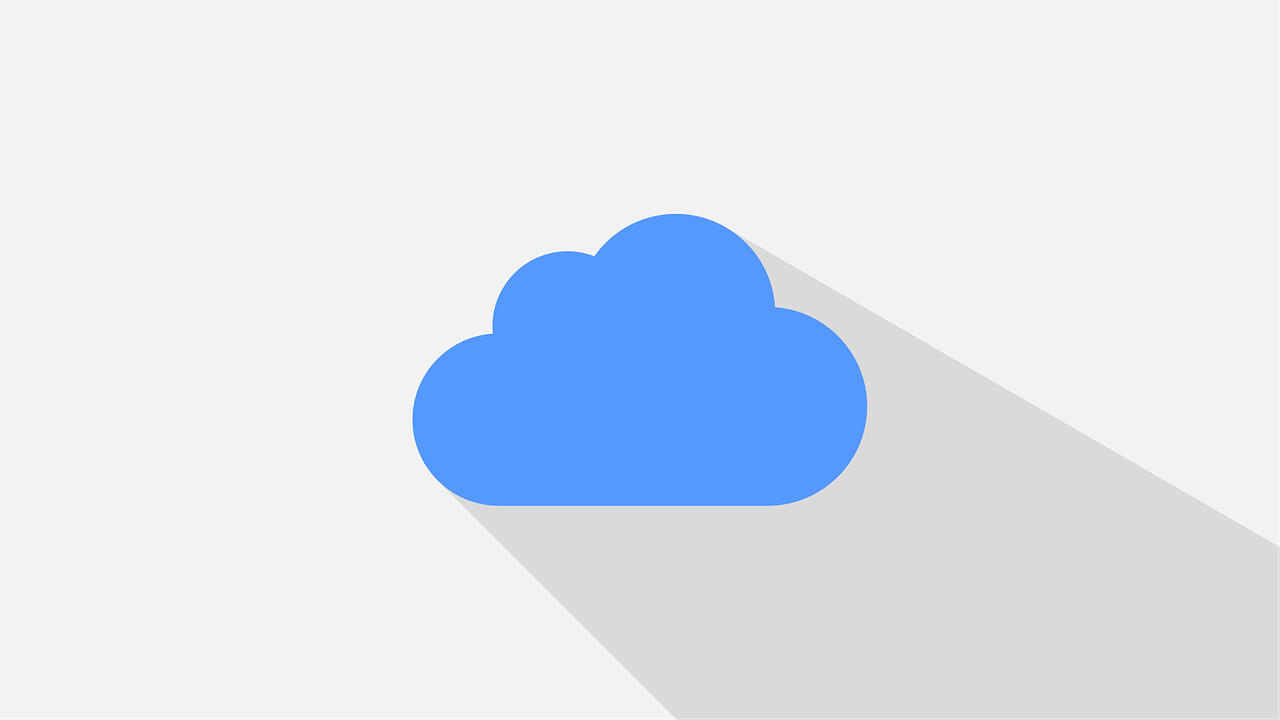
示例2:从文件中读取数据
#include <iostream> #include <fstream> #include <string> int main() { std::ifstream file("example.txt"); std::string line; if (file.is_open()) { while (std::getline(file, line)) { std::cout << line << std::endl; } file.close(); } else { std::cerr << "Unable to open file" << std::endl; } return 0; }
在这个例子中,程序会打开一个名为example.txt
的文件,并逐行读取内容,然后输出到标准输出。
注意事项
缓冲区问题:在使用getline
之前,如果使用了cin >>
,可能会导致输入缓冲区中残留换行符,影响getline
的行为,解决方法是在getline
之前调用cin.ignore()
。
#include <iostream> #include <string> int main() { int age; std::string name; std::cout << "Enter your age: "; std::cin >> age; std::cin.ignore(); // 忽略缓冲区中的换行符 std::cout << "Enter your name: "; std::getline(std::cin, name); std::cout << "Age: " << age << ", Name: " << name << std::endl; return 0; }
空行处理:如果读取的行是空行(仅包含换行符),getline
仍然会成功读取,并将结果字符串设置为空字符串。
常见问题及解答 (FAQs)
Q1: 如果我想读取固定数量的字符而不是整行,该怎么办?
A1: 如果你只想读取固定数量的字符,可以使用std::cin.read
或std::cin.get
。
#include <iostream> #include <string> int main() { char buffer[10]; std::cin.read(buffer, 9); // 读取最多9个字符,留一个位置给终止符 buffer[9] = '