Linux 下使用
gpg
或 openssl
命令进行文件加密,确保数据安全传输。Linux C 加密
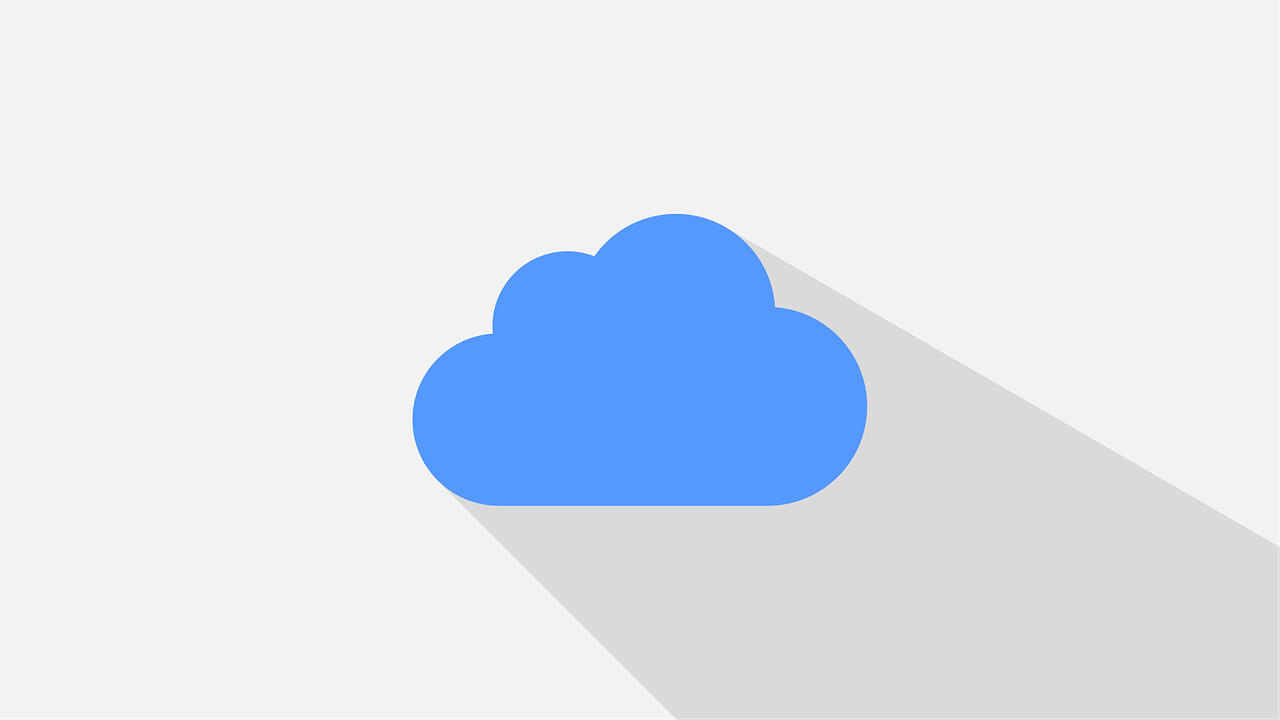
背景介绍
在Linux环境下,C语言提供了多种加密方式,包括按位异或、哈希算法(如MD5和SHA1)以及对称加密算法(如AES),本文将详细介绍这些加密方法及其实现,并通过示例代码进行解释。
按位异或加密
按位异或是一种简单的加密方式,通过与某个数值进行按位异或操作来加密和解密数据。
示例代码
#include<stdio.h> // 加密解密程序 void encrypt(char *message) { while (*message) { *message = *message ^ 31; // 对 message 的每一个字符和 31 进行按位异或 message++; } } int main() { char s[] = "Hello qizexi"; // 运行一次:进行加密 encrypt(s); printf("加密:%s ", s); // 再运行一次是解密 encrypt(s); printf("解密:%s ", s); return 0; }
输出结果
加密:Urjt~vqzdvkxvlw= 解密:Hello qizexi
MD5 加密
MD5是一种广泛使用的哈希函数,尽管它已不再安全,但仍然可以用于学习目的。
示例代码
#include <openssl/md5.h> #include <string.h> #include <stdio.h> int main() { unsigned char outmd[16]; char buffer[] = "hello "; int i; MD5_CTX ctx; MD5_Init(&ctx); MD5_Update(&ctx, buffer, strlen(buffer)); MD5_Final(outmd, &ctx); for (i = 0; i < 16; i++) { printf("%02X", outmd[i]); } printf(" "); return 0; }
编译选项
g++ MD5test.cpp -lssl -o MD5test
输出结果
B1946AC92492D2347C6235B4D2611184
SHA1 加密
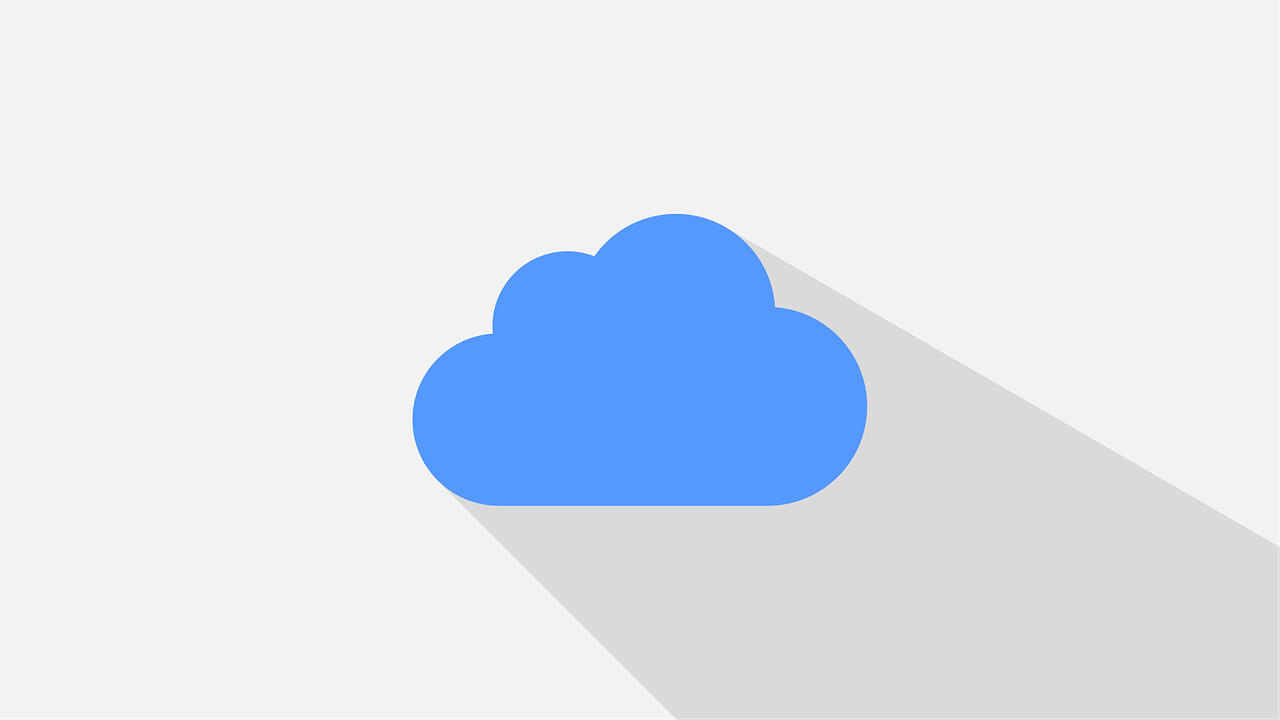
SHA1是MD5的升级版,提供更高的安全性。
示例代码
#include <openssl/sha.h> #include <string.h> #include <stdio.h> int main() { unsigned char outsh[20]; char buffer[] = "hello "; int i; SHA_CTX ctx; SHA1_Init(&ctx); SHA1_Update(&ctx, buffer, strlen(buffer)); SHA1_Final(outsh, &ctx); for (i = 0; i < 20; i++) { printf("%02X", outsh[i]); } printf(" "); return 0; }
编译选项
g++ SHA1test.cpp -lssl -lcrypto -o SHA1test
输出结果
DAF9BAE4E8CEBFB7DFFABEE38B3CD9892E6DF8BB7
AES 加密
AES是一种对称加密标准,广泛用于数据保护,使用OpenSSL库可以实现AES加密。
示例代码
#include <openssl/aes.h> #include <openssl/rand.h> #include <string.h> #include <stdio.h> #include <stdlib.h> void Encryption(const unsigned char *plaintext, int plaintext_len, unsigned char *key, unsigned char *iv, unsigned char *ciphertext) { EVP_CIPHER_CTX *ctx; int len; int ciphertext_len; if (!(ctx = EVP_CIPHER_CTX_new())) handleErrors(); if (1 != EVP_EncryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv)) handleErrors(); if (1 != EVP_EncryptUpdate(ctx, ciphertext, &len, plaintext, plaintext_len)) handleErrors(); ciphertext_len = len; if (1 != EVP_EncryptFinal_ex(ctx, ciphertext + len, &len)) handleErrors(); ciphertext_len += len; EVP_CIPHER_CTX_free(ctx); } int main() { unsigned char *key = (unsigned char *)"0123456789abcdef"; // 密钥长度为16字节(AES-128) unsigned char *iv = (unsigned char *)"1234567890abcdef"; // 初始化向量长度为16字节(AES-128) unsigned char *plaintext = (unsigned char *)"Hello qizexi"; unsigned char ciphertext[128]; int decryptedtext_len, ciphertext_len; unsigned char decryptedtext[128]; Encryption(plaintext, strlen((char *)plaintext), key, iv, ciphertext); printf("加密:"); for (int i = 0; i < strlen((char *)ciphertext); i++) { printf("%02X", ciphertext[i]); } printf(" "); EVP_CIPHER_CTX *ctx; if (!(ctx = EVP_CIPHER_CTX_new())) handleErrors(); if (1 != EVP_DecryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv)) handleErrors(); if (1 != EVP_DecryptUpdate(ctx, decryptedtext, &decryptedtext_len, ciphertext, strlen((char *)ciphertext))) handleErrors(); if (1 != EVP_DecryptFinal_ex(ctx, decryptedtext + decryptedtext_len, &len)) handleErrors(); decryptedtext_len += len; decryptedtext[decryptedtext_len] = '